SCP in Python
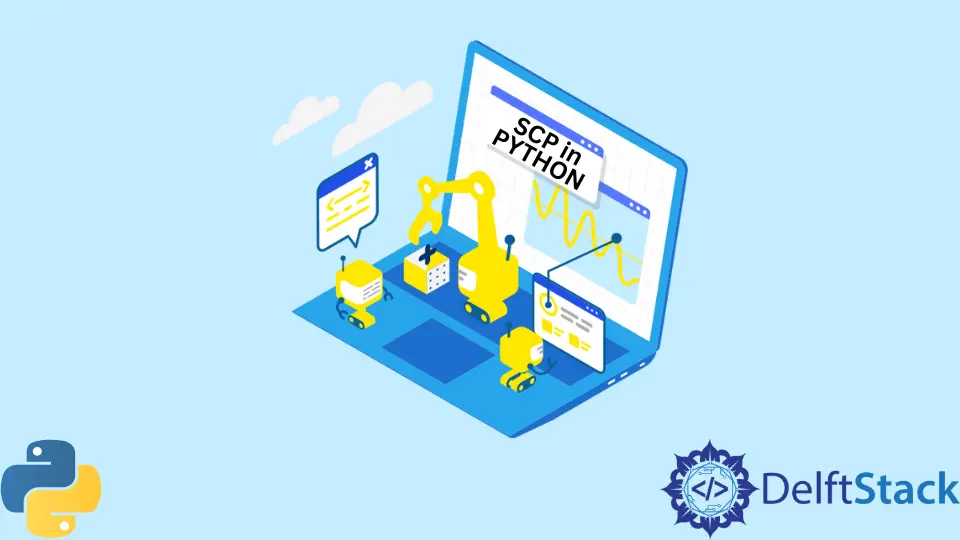
We will introduce SCP
in Python and how to use SCP
in our Python applications to send and receive files.
Using the Scp.py
Library in Python
With the advancement in technology, programming plays an important role. Python is one of the languages that has proved its coverage over a wide variety of domains with utmost versatility.
Python has many modules that help programmers cover many domains and allow Python to succeed. The scp.py
is an open-source Python library used to send and receive files between the client and server using the paramiko
transport.
SCP provides the programming interface to use the SCP1
protocol. SCP1
protocol helps us have multiple conversations over a single TCP
connection between the server and a client.
SCP provides several methods that provide different functionality of sharing file resources between servers and clients.
Now, let’s install the SCP module using CLI, as shown below.
# python
pip install scp
This command will install the library of SCP
, and now we can use it in our examples. So in our example, we will import SSHClient
from the paramiko
module to establish a connection between the client and the server.
We will also import the SCPClient
from the SCP
module to define file sharing protocols. After importing, we will initiate the client object and connect it through the server.
Now, we will initiate the SCP client object that will take the SSHClient
transport as the argument.
After that, we will send our file using the put()
method. The put()
method uses the file name as the argument.
We can also download the files from the server using another method, get()
. We will use it to download the files from the server, as shown below.
# python
from paramiko import SSHClient
from scp import SCPClient
ssh = SSHClient()
ssh.load_system_host_keys()
ssh.connect("test.net")
scp = SCPClient(ssh.get_transport())
scp.put("example.txt", "example2.txt")
scp.get("example2.txt")
Now let’s discuss what we can do if we have to upload a directory instead of a file. We can use the put()
method as shown below.
# python
scp.put("example", recursive=True, remote_path="/home")
As you can see from the above example, we sent the whole directory named example
from our local computer to the server. We can also specify the path as the argument for the server computer using the keyword argument name remote_path
.
Now let’s go through an example in which we will track the progress of the file we are sending or downloading from the server. We will implement a progress function that writes the progress of the shared file.
As shown below, we will use another function known as progress()
.
# python
from paramiko import SSHClient
from scp import SCPClient
import sys
ssh = SSHClient()
ssh.load_system_host_keys()
ssh.connect("test.com")
def progress(filename, filesize, filesent):
sys.stdout.write(
"%s's progress: %.2f%% \r"
% (filename, float(filesent) / float(filesize) * 100)
)
scp = SCPClient(ssh.get_transport(), progress=progress)
def progress4(filename, filesize, filesent, peername):
sys.stdout.write(
"(%s:%s) %s's progress: %.2f%% \r"
% (peername[0], peername[1], filename, float(filesent) / float(filesize) * 100)
)
scp = SCPClient(ssh.get_transport(), progress4=progress4)
scp.put("example.txt", "~/example.txt")
scp.close()
So in this way, we can easily send and download files from the server using the SCP
library in Python.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn