The Schedule Package in Python
- Install the Schedule Library in Python
- Use Seconds as Interval to Schedule a Job Periodically
- Use Minutes, Hours, and Days as Interval to Schedule a Job Periodically
-
Use Time in
HH:MM
Format as Interval to Schedule a Job Periodically - Use the Function Decorator to Schedule a Job
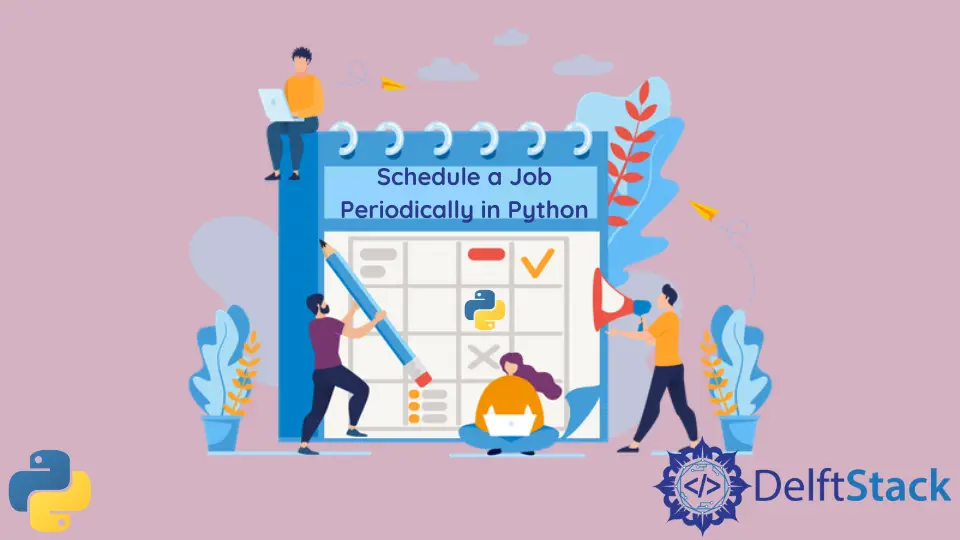
This tutorial will demonstrate the Schedule package in Python to schedule jobs periodically after a specific time interval.
The Schedule is a lightweight process scheduler library in Python used to schedule a task to run periodically at a specified time interval.
We can call a function or any callable object using a human-friendly syntax to automate tasks like sending emails, setting up a reminder, fetching the price of a currency, or bitcoin periodically.
Install the Schedule Library in Python
We have to install this package first using the following command to use it.
#Python 3.x
pip install schedule
To schedule a job, we use the following syntax.
Syntax:
# Python 3.x
Schedule.every(interval).[timeframe].do(function)
Parameters:
- The
interval
can be any integer. - The
timeframe
can be seconds, minutes, hours, days, or even the name of weekdays like Monday, Tuesday, etc. - The
do()
method takes the function name as an argument to schedule.
Use Seconds as Interval to Schedule a Job Periodically
We have a method named task
in the following code, scheduled to run periodically after every five seconds. We define the time interval (five seconds in our case) as an argument to the every()
method.
We specify the function to schedule as an argument to the do()
method that automatically runs once the machine reaches the specified time. Finally, we execute the run_pending()
function in the while
loop to run the scheduled jobs continuously.
In the output, the message Task is running
keeps displaying after every five seconds as programmed.
Example Code:
# Python 3.x
import schedule as s
import time as t
def task():
print("Task is running")
s.every(5).seconds.do(task)
while True:
s.run_pending()
t.sleep(1)
Output:
#Python 3.x
Task is running
Task is running
Task is running
Task is running
Task is running
Use Minutes, Hours, and Days as Interval to Schedule a Job Periodically
If we want to change the interval to any specified minutes, hours, or days of the week, we can write the code like this. The output will keep displaying the message according to the time set.
Example Code:
# Python 3.x
import schedule as s
import time as t
def task():
print("Task is running")
s.every(5).minutes.do(task)
s.every(10).hours.do(task)
s.every().monday.do(task)
while True:
s.run_pending()
t.sleep(1)
Output:
#Python 3.x
Task is running
Task is running
Task is running
Use Time in HH:MM
Format as Interval to Schedule a Job Periodically
We can also set a specific time in the HH:MM:SS
format as an argument to a function. The message will keep displaying periodically after every specified interval.
Example Code:
# Python 3.x
import schedule as s
import time as t
def task():
print("Task is running")
s.every().day.at("04:21").do(task)
s.every().monday.at("12:00").do(task)
while True:
s.run_pending()
t.sleep(1)
Output:
#Python 3.x
Task is running
Use the Function Decorator to Schedule a Job
A function decorator accepts a function as an input, adds some functionality, and returns a function. We will use the @repeat
to schedule the task function here.
Example Code:
# Python 3.x
from schedule import every, run_pending, repeat
import time as t
@repeat(every(5).seconds)
def task():
print("Task is running")
while True:
run_pending()
t.sleep(1)
Output:
#Python 3.x
Task is running
Task is running
Task is running
Run a Job at Once
We can run a job at once by canceling it after it runs using CancelJob
with the schedule instance.
Example Code:
# Python 3.x
import schedule as s
import time as t
def task():
print("Task is running")
return s.CancelJob
s.every(5).seconds.do(task)
while True:
s.run_pending()
t.sleep(1)
Output:
#Python 3.x
Task is running
Cancel All Jobs
We can cancel all the jobs from the scheduler using the clear()
method with the scheduler instance. Here, we have used the get_jobs()
method to print the scheduled jobs from the scheduler.
Example Code:
# Python 3.x
import schedule as s
import time as t
def task():
print("Task is running")
s.every(5).seconds.do(task)
print("Jobs:", s.get_jobs())
s.clear()
print("Jobs:", s.get_jobs())
Output:
#Python 3.x
Jobs: [Every 5 seconds do task() (last run: [never], next run: 2022-04-08 16:59:37)]
Jobs: []
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn