The Sscanf() Functionality in Python
-
the
sscanf()
Functionality in Python -
Use Regular Expressions From the
re
Library -
Use the
Neuron
Library -
Possible Error Encounter in Using Python
scanf
- Conclusion
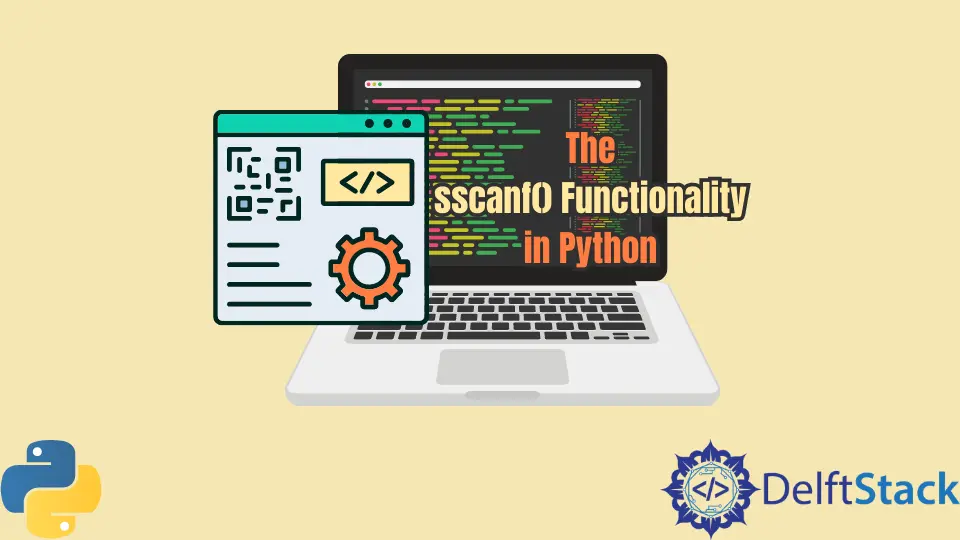
Input processing is a fundamental aspect of programming.
The function sscanf()
is from the programming languages C and C++. While Python does not offer any exact equivalent method or library for this method, there may be other ways to execute this functionality.
In Python, the sscanf
function provides a powerful way to parse formatted input strings, akin to its counterpart in the C programming language.
While Python offers various input methods, such as input()
and string manipulation functions, sscanf
streamlines the process of reading formatted input, making it particularly useful when dealing with structured data.
This article will make you better understand what sscanf()
has to offer and how we can mimic it in a Python script.
the sscanf()
Functionality in Python
The sscanf()
method extracts a string from an already provided string. This is how we declare the method:
int sscanf(const char *str, const char *format, ...)
This method will essentially read formatted input from a string, and unlike scanf()
, the data for sscanf()
is read from a string instead of a console. The data is read from the buffer and into the location addresses provided as arguments in the method declaration.
Every argument provided points towards a variable with the same type as the format string. The method returns the values that were successfully converted and assigned.
There are no direct equivalent libraries or modules for sscanf()
in Python itself. However, there are two ways to mimic the functionality.
Use Regular Expressions From the re
Library
A regular expression helps specify a string’s format or describe a string’s format, which can then be used to validate a different string. A regular expression may contain special as well as ordinary characters.
Characters like A
, B
, b
, or 0
are good examples of the simplest of ordinary characters in an expression. The library can also search for specific characters in a string or a list of characters in a particular order.
Look at the example script below that searches for the string def
in a provided string.
Example Code:
import re
m = re.search("(?<=abc)def", "abcdef")
m.group(0)
print(m)
The code snippet utilizes the re
module to perform a pattern search within a string using regular expressions.
It employs the re.search()
function to find the substring "def"
preceded by "abc"
within the string "abcdef"
.
Once the match is found, the code accesses the matched group using the group(0)
method of the match object m
.
Subsequently, the match object m
is printed, displaying information about the matched pattern, specifically the substring "def"
.
Output:
The program returns def
as output.
It is also possible to search for strings within strings separated by special characters. For example, in the script below, we search for the word provided after a hyphen.
Example Code:
import re
m = re.search(r"(?<=-)\w+", "spam-emails")
m.group(0)
print(m)
The provided Python code snippet showcases the utilization of the re
module for pattern matching using regular expressions.
With the re.search()
function, the code seeks a pattern in the string "spam-emails"
, specifically targeting alphanumeric characters (\w+
) preceded by a hyphen ((?<=-)
).
Following successful pattern matching, the code accesses the matched group via the group(0)
method of the match object m
.
Subsequently, the code prints the match object m
, displaying information regarding the matched pattern, which in this case would be the substring "emails"
.
Output:
The output for this is emails
. The possibilities of parsing with re
are endless.
Another library similar to re
is regex
, which is API-friendly. Regex is backward compatible with re
and comes with additional functionalities.
Following is a conditional pattern test made possible with a regex
module. We will, of course, need to import the library before executing this.
Example Code:
# Make sure to install the `regex` module first (e.g., via `pip install regex`)
import regex
# Match one or more digits if the string starts with a digit; otherwise, match one or more word characters
pattern = r"(?(?=\d)\d+|\w+)"
# Test cases
input_str1 = "123abc"
input_str2 = "abc123"
# Perform pattern matching
match1 = regex.match(pattern, input_str1)
match2 = regex.match(pattern, input_str2)
# Print match results
print(match1) # Output: <regex.Match object; span=(0, 3), match='123'>
print(match2) # Output: <regex.Match object; span=(0, 6), match='abc123'>
We import the regex
module, which provides extended functionality for regular expressions.
Then, we define the pattern (?(?=\d)\d+|\w+)
, where (?(?=\d)\d+|\w+)
is a conditional expression. It checks if the input string starts with a digit using the positive lookahead (?=\d)
.
If it does, it matches one or more digits (\d+
); otherwise, it matches one or more word characters (\w+
).
Next, we test the pattern against the input strings '123abc'
and 'abc123'
using the regex.match()
function.
Lastly, we print the match objects match1
and match2
, which contain information about the matched patterns and their locations within the input strings.
Output:
For the input string '123abc'
, the pattern matches '123'
as it starts with digits, resulting in a match object with a span from index 0 to index 3, and the matched string is '123'
.
For the input string 'abc123'
, the pattern matches the entire string 'abc123'
as it doesn’t start with digits, resulting in a match object with a span from index 0 to index 6, and the matched string is 'abc123'
.
Use the Neuron
Library
The neuron
library (not from Python itself) can also be used to import sscanf()
in a Python script.
The code snippet below utilizes the NEURON
simulation environment in Python (from neuron import h
). It aims to parse a float value from the string "0.3"
using the h.sscanf()
function, which is a NEURON
-specific function for parsing formatted input similar to the standard C sscanf()
function.
Example code:
from neuron import h
x = h.ref(0)
h.sscanf("0.3", "%f", x)
print(x[0])
The string "0.3"
is parsed as a floating-point number using the format specifier "%f"
in h.sscanf()
.
The parsed value is stored in the NEURON h.ref()
object x
.
When printing x[0]
, it displays the value stored in the NEURON
reference object.
Output:
0.30000001192092896
The resulting output would be 0.30000001192092896
.
Here are some more examples we can go through to understand the use of sscanf()
in Python via the neuron
library.
This code snippet utilizes the NEURON
simulation environment in Python, with the NEURON
module imported as hoc
for convenience.
It demonstrates the usage of the hoc.sscanf()
function, which is a NEURON
-specific function for parsing formatted input similar to the standard C sscanf()
function.
Example Code:
from neuron import h as hoc
string = hoc.ref("")
range_list = [hoc.ref(0) for i in range(50)]
hoc.sscanf("This is a test\n", "%s", string)
print(string[0])
hoc.sscanf("This is a test\n", "%[^\n]", string)
print(string[0])
hoc.sscanf("This is a test\n", "%*s%s", string)
print(string[0])
hoc.sscanf(
"1 2 3 4 5 6 7 8 9 10",
"%f%f%f%f%f%f%f%f%f%f%f",
range_list[0],
range_list[1],
range_list[2],
range_list[3],
range_list[4],
range_list[5],
range_list[6],
range_list[7],
range_list[8],
range_list[9],
range_list[10],
range_list[11],
range_list[12],
)
print("Should only have non-zero values for range_list indices 0 - 9")
for i in range(13):
print("%d %g" % (i, range_list[i][0]))
The code initializes the NEURON
simulation environment and aliases it as hoc
.
It defines a NEURON
reference object string
to hold parsed string values. Additionally, it creates a list range_list
of NEURON
reference objects to store parsed floating-point values.
It parses a string "This is a test\n"
using the format specifier "%s"
and stores the result in string[0]
.
It parses the same string excluding the newline character using the format specifier "%[^\n]"
.
It demonstrates the use of the *
assignment suppression specifier in "%*s%s"
, which discards the first string and stores the second in string[0]
.
It parses a space-separated list of floating-point numbers "1 2 3 4 5 6 7 8 9 10"
using the format specifier "%f%f%f%f%f%f%f%f%f%f%f"
, storing the results in the respective elements of range_list
.
Finally, the code prints the values stored in range_list
to verify successful parsing, indicating that only indices 0 to 9 should contain non-zero values.
Output:
This
This is a test
is
Should only have non-zero values for range_list indices 0 - 9
0 1
1 2
2 3
3 4
4 5
5 6
6 7
7 8
8 9
9 10
10 0
11 0
12 0
Overall, the output demonstrates the successful parsing of different patterns from the input string using the hoc.sscanf()
function, showcasing its versatility in handling formatted input within the NEURON
simulation environment.
Possible Error Encounter in Using Python scanf
One common error that can be encountered when using the scanf()
function in Python is a mismatch between the format specifier and the input string.
This can occur if the format specifier expects a certain type of input data, but the actual input string does not match that format. For example, trying to parse a string as an integer or vice versa can result in a ValueError
.
To handle such errors gracefully, you can use exception handling with a try-except
block. Here’s how you can do it:
from scanf import scanf
# Attempt to parse input string according to format specifier
result = scanf("%d", "abc")
# Check if result is None (indicating failure to parse)
if result is None:
print("Error: Failed to parse input string as an integer.")
else:
print(result)
We use a try-except
block to encapsulate the scanf()
function call.
Inside the try
block, we attempt to parse the input string "abc"
as an integer using the format specifier "%d"
.
If a ValueError
occurs during the parsing process (e.g., because "abc"
cannot be parsed as an integer), the control flow jumps to the except
block.
In the except
block, we catch the ValueError
and handle it by printing an error message.
Output:
Error: Failed to parse input string as an integer.
Conclusion
In conclusion, input processing is a crucial aspect of programming, facilitating data manipulation and analysis.
Although Python does not offer an exact equivalent to the sscanf()
function found in languages like C and C++, various alternatives exist to achieve similar functionality.
Regular expressions, exemplified through Python’s re
and regex
modules, provide robust pattern-matching capabilities for parsing formatted input strings.
Additionally, specialized libraries like NEURON’s hoc
module offer specific functions like hoc.sscanf()
for parsing input within their respective environments.
Handling errors, such as mismatched format specifiers, is essential for robust input processing, which can be achieved through exception handling in Python.
By leveraging these techniques, programmers can effectively process input data to meet the requirements of their applications.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn