How to Perform Authentication Using the Requests Module in Python
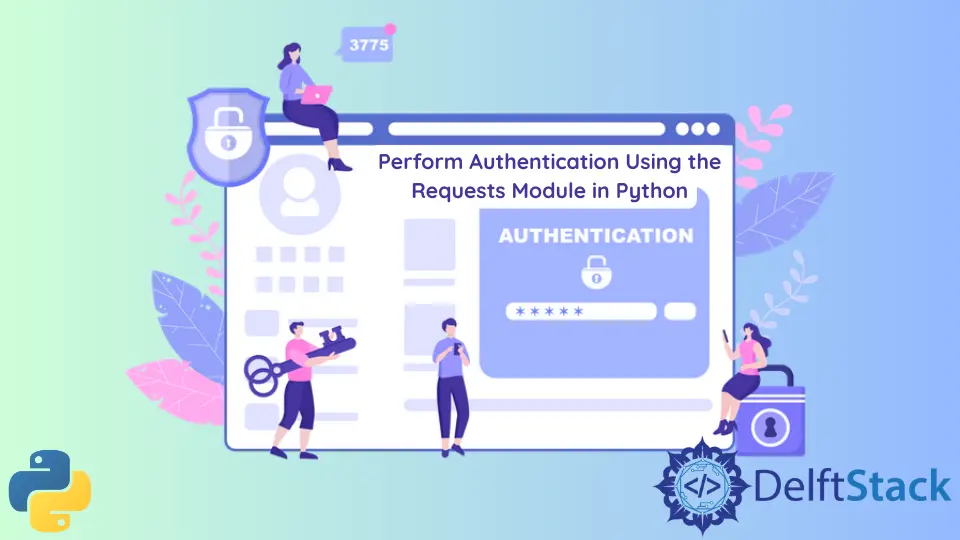
The Hypertext Transfer Protocol is the basis of the World Wide Web or the internet. Essentially, it is an application-layer protocol whose main task is to transfer data from web servers to web browsers such as Chrome, Edge, Firefox, and Brave.
The HTTP requests
are made to unique web addresses or URLs to fetch required resources and media documents such as HTML, PDFs, text files, images, videos, etc.
A URL, also known as Uniform Resource Locator, is a unique web address or path used to locate content over the internet, and each URL points to specific content.
Python is a dynamically-typed multi-purpose programming language backed with a massive pool of libraries. A requests
module offers utilities to perform HTTP requests using Python programming language.
Perform Authentication Using the requests
Module in Python
To perform authentication with the help of the requests
module, we can use the HTTPBasicAuth
class from the requests
library. This class accepts two parameters, a username, and a password.
This class represents an HTTP Basic Authentication
, and it is attached to a request. The authentication object is offered to the auth
parameter of the get()
method.
Let us understand this class and its usage with the help of an example. Refer to the following Python code.
from requests.auth import HTTPBasicAuth
url = "<any valid url>"
basic = HTTPBasicAuth("username", "password")
requests.get(url, auth=basic)
A response object with a 200
status code is obtained on successful authentication.
An alternative and more straightforward way to write the above code is as follows.
from requests.auth import HTTPBasicAuth
url = "<any valid url>"
requests.get(url, auth=("username", "password"))
One can provide a username and password to the auth
parameter in a tuple.
the Digest Authentication in Python
Another prevalent and straightforward form of HTTP Authentication is Digest Authentication
. This type of authentication can be performed with the help of HTTPDigestAuth
.
Like the HTTPBasicAuth
class, this class also accepts a username and a password.
Let us look at an example. Refer to the following Python code.
from requests.auth import HTTPBasicAuth
url = "<any valid url>"
requests.get(url, auth=HTTPDigestAuth("username", "password"))
the OAuth 1
Authentication in Python
The OAuth 1
Authentication is a robust form of authentication.
Here, OAuth
stands for Open Authorization. This form of authentication works well with web APIs or Application Programming Interface that use OAuth
.
This type of authentication can be performed with the help of the OAuth1
class. This class is a part of the requests-oauthlib
library. To install this Python library, use the following pip
command.
pip install requests-oauthlib
Let us understand this class with the help of an example. Refer to the following Python code for the same.
import requests
from requests_oauthlib import OAuth1
url = "<any valid url>"
YOUR_APP_KEY = "YOUR_APP_KEY"
YOUR_APP_SECRET = "YOUR_APP_SECRET"
USER_OAUTH_TOKEN = "USER_OAUTH_TOKEN"
USER_OAUTH_TOKEN_SECRET = "USER_OAUTH_TOKEN_SECRET"
auth = OAuth1(YOUR_APP_KEY, YOUR_APP_SECRET, USER_OAUTH_TOKEN, USER_OAUTH_TOKEN_SECRET)
requests.get(url, auth=auth)
the OAuth 2
Authentication in Python
In 2012, OAuth 1
was replaced by a newer and more reliable authentication protocol called OAuth 2
.
With the help of OAuth 2
, developers and business owners can provide consented access to resources over their websites and restrict fishy actions.
The OAuth 2
authentication uses access tokens. Access tokens are a special kind of data that allows users to authenticate.
A user has to include its access token in every request it makes to a website’s server to prove its identity authentication in layman terms
and access whatever content they have rights for.
Since one can access tokens easily using a web browser, every access token has an expiration date and time for security purposes.
Using OAuth 2
authentication, users can authenticate over multiple platforms, saving time for the end-user and promoting a satisfactory user experience.
One can perform OAuth 2
authentication using the requests_oauthlib
module. This module has a class OAuth2Session
that we can use.
Following is the class signature of OAuth2Session
.
class requests_oauthlib.OAuth2Session(client_id=None, client=None, auto_refresh_url=None, auto_refresh_kwargs=None, scope=None, redirect_uri=None, token=None, state=None, token_updater=None, **kwargs)
Let us understand its usage with the help of an example.
from requests_oauthlib import OAuth2Session
client_id = "your_client_id"
client_secret = "your_client_secret"
url = "<any valid url>"
oauth = OAuth2Session(client_id, redirect_uri=url)
token = oauth.fetch_token("<url to fetch access token>", client_secret=client_secret)
r = oauth.get("<url to a resource>")
The OAuth2Session
class accepts a client_id
and a client_secret
used to perform the actual authentication.
The url
is a good website for which the authentication must be completed. We fetch our new access token using this class object’s fetch_token()
method.
The token will use for the rest of the session to access the content. Here, <url to fetch access token>
should be an HTTPS web address.
Once the token is obtained, we can safely access content over the website using this class object’s get()
method.
To learn more about this method, refer to the official documentation here.
Other Authentication Methods in Python
Apart from the methods mentioned above, we can also perform authentication using two Python libraries.
requests Kerberos/GSSAPI
requests-ntlm
The requests Kerberos/GSSAPI
library is an open-source Python-based library that amplifies the scope of the requests
library.
A requests
is for HTTP
requests, but the requests Kerberos/GSSAPI
library supports Kerberos/GSSAPI authentication and mutual authentication.
The following Python depicts how to use this library to perform basic authentication.
import requests
from requests_kerberos import HTTPKerberosAuth
r = requests.get("<any valid url>", auth=HTTPKerberosAuth())
The requests-ntlm
library is an open-source Python-based library for HTTP NTLM
authentication. NTLM
stands for Windows NT LAN Manager.
The NTLM
protocol is generally considered insecure because it is a pretty old protocol and is based on an outdated cryptography
algorithm.
Hence, it is vulnerable to fishy attacks such as pass-the-hash attacks and brute-force attacks. To install this library, execute the following pip
command in any terminal.
pip install requests_ntlm
The following Python depicts how to use this library to perform NTLM
authentication.
import requests
from requests_ntlm import HttpNtlmAuth
requests.get("<any valid url>", auth=HttpNtlmAuth("domain\\username", "password"))