How to Remove Parentheses From String in Python
-
Use the
str.replace()
Function to Remove Parentheses From a String in Python - Use Regular Expressions to Remove Parentheses From a String in Python
- Use a Loop and Result String to Remove Parentheses From a String in Python
-
Use List Comprehension and the
join()
Function to Remove Parentheses From a String in Python - Conclusion
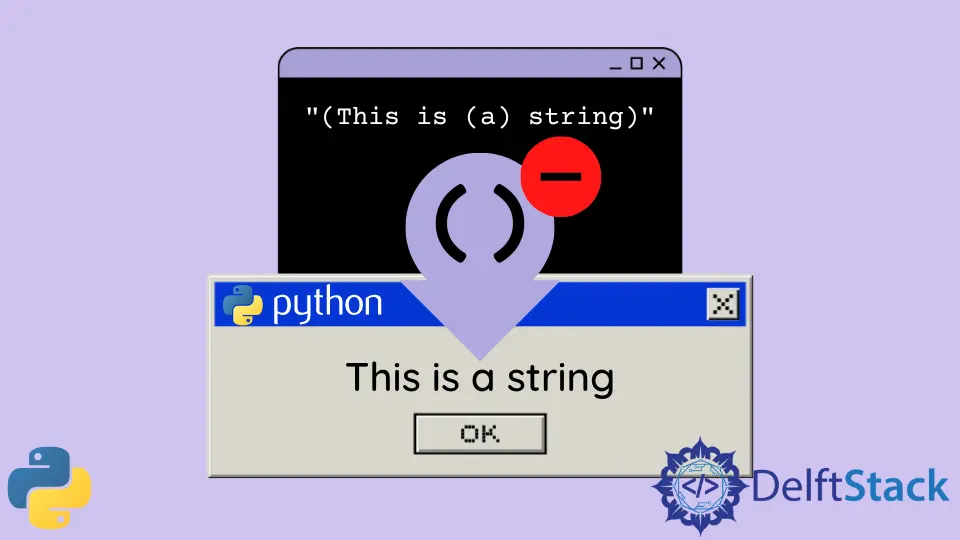
In Python, one common operation is the removal of specific characters, such as parentheses, from a string. In this tutorial, we will explore four methods to accomplish this task effectively.
Use the str.replace()
Function to Remove Parentheses From a String in Python
The str.replace()
function in Python is a built-in string method used to replace all occurrences of a specified substring or character(s) within a given string with another substring.
In the following syntax, str.replace()
is a method used to modify a string by finding and replacing occurrences of a specified substring (old_substring
) with another substring (new_substring
) within the original_string
. The result is stored in the new_string
variable.
Syntax:
new_string = original_string.replace(old_substring, new_substring, count)
Parameters:
new_string
: The resulting string after the replacement is performed.original_string
: The string we want to perform the replacement on.old_substring
: The substring we want to find and replace in theoriginal_string
.new_substring
: The substring that will replaceold_substring
in theoriginal_string
. If we want to remove theold_substring
, we can replace it with an empty string""
.count
(optional): The maximum number of replacements to make. It’s an optional parameter, and if not provided, all occurrences ofold_substring
are replaced.
Code Example:
string = "(This is (a) string)"
string = string.replace("(", "").replace(")", "")
print(string)
In this code, we start with the string "(This is (a) string)"
. We use the str.replace()
method twice consecutively.
In the first call to string.replace("(", "")
, we search for all instances of open parentheses '('
, and replace them with an empty string, effectively removing them from the original string. In the second call to replace(")", "")
, we do the same for the close parentheses ')'
, replacing them with an empty string as well.
The result is a modified string, and after these replacements, the string becomes "This is a string"
with all the parentheses removed.
Output:
This is a string
The output This is a string
, which is the original string with all the parentheses removed.
Use Regular Expressions to Remove Parentheses From a String in Python
The regular expression [()]
matches and identifies open and closed parentheses in a string, allowing you to remove or replace them as needed in the text. When this regular expression is used with the re.sub()
function, it effectively matches and identifies every occurrence of an open parenthesis '('
or a close parenthesis ')'
in the input string.
Once matched, the regular expression replaces these matched characters with an empty string.
In the following syntax, we can use regular expressions in Python to search for specific patterns in a string (pattern
) and replace them (with replacement
). The result is stored in result_string
, providing us with the modified string based on the defined regular expression pattern.
Syntax:
import re
result_string = re.sub(pattern, replacement, original_string)
Parameters:
pattern
: The regular expression pattern that defines what we want to find in theoriginal_string
. In this case, it’s typically a pattern that matches open and closed parentheses, like"[()]"
.replacement
: The string that will replace the matched patterns. Here, we replace the parentheses with an empty string""
.original_string
: The string in which we want to perform the replacement using the specified regular expression pattern.
Code Example:
import re
string = "(This is (a) string)"
string = re.sub("[()]", "", string)
print(string)
In this code, we are using the Python re
module to manipulate a string. We start with the string "(This is (a) string)"
that contains parentheses.
Then, we employ the re.sub()
function to perform a substitution. Specifically, we use the pattern "[()]"
to search for and match any occurrence of open parenthesis '('
or close parenthesis ')'
in the string.
Next, we replace these matched parentheses with an empty string, effectively removing them. The result is then stored back in the string
variable, then we print the modified string, which outputs This is a string
.
Output:
This is a string
The output This is a string
, which is the original string with all the parentheses removed.
Use a Loop and Result String to Remove Parentheses From a String in Python
We can use a loop and a result string to iteratively process characters in an original string, selectively excluding parentheses, and build a result string without them.
In the following syntax, we initialize a result_string
variable as an empty string to hold the modified string. We then use a for
loop to iterate through each character char
in the original_string
.
Within the loop, we check if the current character char
is not in the set of characters '()'
(which represents open and closed parentheses). If the condition is met, indicating that the character is not a parenthesis, we append that character to the result_string
.
As we continue looping through each character in the original_string
, we build the result_string
by selectively excluding the characters that are parentheses.
Syntax:
result_string = ""
for char in original_string:
if char not in "()":
result_string += char
Variables:
original_string
: This variable holds the original string from which we want to remove characters, in this case, parentheses. It serves as the input to the process.result_string
: This variable represents the result or output where the modified string, without the specified characters, is stored. It’s updated as the loop iterates through theoriginal_string
.
The method doesn’t use formal parameters like a function but directly operates on the input string, creating the modified result string without parentheses.
Code Example:
original_string = "(This is (a) string)"
string_without_parentheses = ""
for char in original_string:
if char not in "()":
string_without_parentheses += char
print(string_without_parentheses)
In this code, we have an original_string
which is initially set to "(This is (a) string)"
and contains parentheses. We initialize an empty string called string_without_parentheses
to store the modified string without the parentheses.
Then, we use a for
loop to iterate through each character in the original_string
. Within the loop, we check if the current character char
is not an open parenthesis '('
or a close parenthesis ')'
and if it’s not a parenthesis, we append it to the string_without_parentheses
.
After the loop completes, string_without_parentheses
holds the modified string, which is the original string with the parentheses removed. Finally, we print string_without_parentheses
, which results in the output This is a string
.
Output:
This is a string
The output shows This is a string
, which is the original string with all the parentheses removed.
Use List Comprehension and the join()
Function to Remove Parentheses From a String in Python
In the following syntax, we use list comprehension and the join()
function to create result_string
. We start by iterating through each character char
in the original_string
using list comprehension.
Within the list comprehension, we evaluate if each character is not an open parenthesis '('
or a close parenthesis ')'
. If a character is not a parenthesis, it is included in the list.
After we’ve filtered out the parentheses, we use the join()
function to concatenate the characters together without any separators. The final result is stored in result_string
.
Syntax:
result_string = "".join([char for char in original_string if char not in "()"])
Variables:
original_string
: This variable holds the original string from which we want to remove characters, in this case, parentheses. It serves as the input to the process.result_string
: This variable represents the result or output where the modified string, without the specified characters, is stored. It’s updated as the loop iterates through theoriginal_string
.
This method doesn’t involve formal parameters. Instead, it directly operates on the original_string
to create a modified result string, result_string
.
Code Example:
original_string = "(This is (a) string)"
string_without_parentheses = "".join(
[char for char in original_string if char not in "()"]
)
print(string_without_parentheses)
In this code, we start with the original_string
, which is initially set to "(This is (a) string)"
and contains parentheses. We use a list comprehension within a join()
function to create string_without_parentheses
.
The list comprehension iterates through each character in the original_string
, and we only include characters in string_without_parentheses
if they are not open or close parentheses '('
or ')'
. This effectively filters out the parentheses from the original_string
.
Finally, we print string_without_parentheses
, which results in the output "This is a string"
.
Output:
This is a string
The output This is a string
, which is the original string with all the parentheses removed.
Conclusion
In this tutorial, we have explored four different methods for removing parentheses from a string in Python. Whether you prefer the simplicity of str.replace()
, the versatility of regular expressions, the control of a loop and a result string, or the elegance of list comprehension and join()
, you now have a range of tools at your disposal to tackle similar tasks in your Python projects.
Understanding these methods equips you with the knowledge to confidently manipulate and transform strings in Python, opening up a world of possibilities for text processing and data manipulation.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn