How to Read Outlook Email using Python
-
Use the
win32com.client
Module to Read Email From the Outlook Application - Filter Emails With Properties
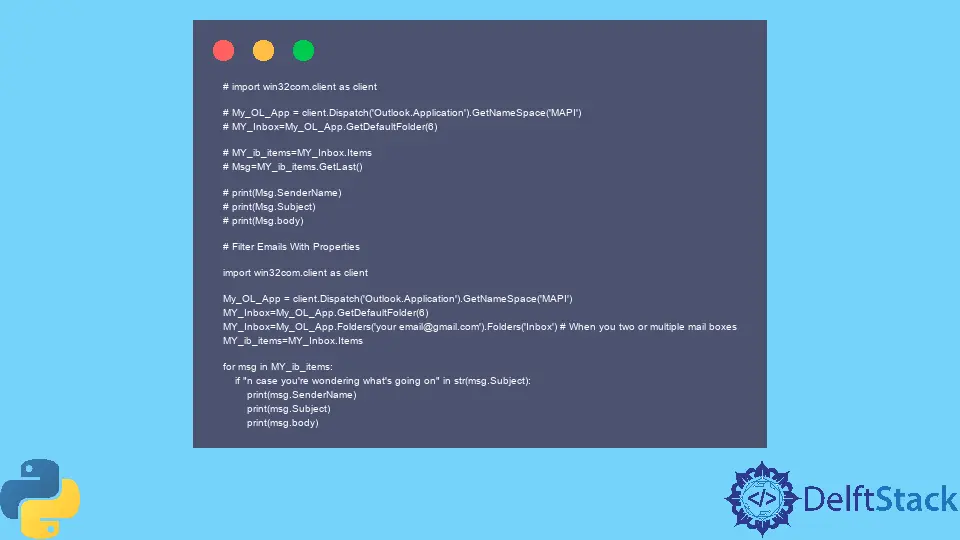
This article will discuss how to read emails from the outlook application with the help of win32com.client module. We also learn how to filter emails with different properties in Python.
Use the win32com.client
Module to Read Email From the Outlook Application
Let’s say you want to keep monitoring a certain product on the amazon website to check if the price of that product fluctuates. To keep it simple, we will just read emails from Outlook using Python script.
We will create a Python program that will read the latest email and let us know who is the email’s sender and what the email’s subject is. This is also going to let us know what the body of the email is.
First, we will install the pywin32
package.
pip install pywin32
This package calls Outlook and reads emails from Outlook. Once this is done, we are good to go to write our program by importing the client
module from win32com
.
import win32com.client as client
Now we create the variable called My_OL_App
, and we will get objects using Dispatch()
. The Dispatch()
function accepts an Outlook.Application
, and we will call GetNameSpace()
, which takes an API named MAPI
.
In the next line, we create another variable called MY_Inbox
, then we will call My_OL_App.GetDefaultFolder()
will store data that is getting fetched from the Outlook application. Now we pit 6 inside the parenthesis, which denotes the inbox folder.
The numbers denote different kinds of folders; for example, 5 denotes the sent item, and similarly, for deleted items, for archived items. We have a list of different numbers that denote folders has been mentioned in this link you can check it if you want to traverse through any other folder.
My_OL_App = client.Dispatch("Outlook.Application").GetNameSpace("MAPI")
MY_Inbox = My_OL_App.GetDefaultFolder(6)
We will have access to the whole inbox while calling Items
to store fetched messages or different emails, and we will store it in the MY_ib_items
variable. We will create another variable called Msg
, then call MY_ib_items.GetLast()
, which will fetch the latest email.
MY_ib_items = MY_Inbox.Items
Msg = MY_ib_items.GetLast()
If you want to traverse through all the emails, we will show you that later in this tutorial. Now, we can print the SenderName
, Subject
and body
using the following code.
Make sure your email is connected with the outlook application.
print(Msg.SenderName)
print(Msg.Subject)
print(Msg.body)
Filter Emails With Properties
Now we will look at how to filter emails by choosing the mail message properties like SenderName
, Subject,
etc. We have seen how to read the newest email using the GetLast()
method from the outlook application.
We will also look at how to extract the newest and oldest emails from the outlook application. Since we have learned how to create an instance of Outlook, which means we are loading Outlook in the memory as well as we are familiar with the following code.
import win32com.client as client
My_OL_App = client.Dispatch("Outlook.Application").GetNameSpace("MAPI")
# MY_Inbox=My_OL_App.GetDefaultFolder(6)
MY_Inbox = My_OL_App.Folders("your email@gmail.com").Folders(
"Inbox"
) # When you two or multiple mail boxes
MY_ib_items = MY_Inbox.Items
You could have two different mailboxes, so when you want to read multiple outlook mailboxes, you can define the mailbox and the folder you want to load in your variable. The above code is the same instead of this line of code; if you have only one mailbox, you do not need to put the following code.
MY_Inbox = My_OL_App.Folders("your email@gmail.com").Folders("Inbox")
We can put the relevant email address connected to your Outlook application. We can extract all the inbox items using the Items
property which will be a collection, and then we can loop on these items one by one.
When looping on these items, we are loading emails from the mailbox one by one. We will filter emails using the Subject
property, and the loop will iterate through the inbox and then check whether the email’s subject matches.
for msg in MY_ib_items:
if "n case you're wondering what's going on" in str(msg.Subject):
print(msg.SenderName)
print(msg.Subject)
print(msg.body)
You can filter emails using different properties such as; SenderEmailAddress
and SenderName
, or you can define conditions with multiple properties in a single if
statement.
if (
"n case you're wondering what's going on" in str(msg.Subject)
and msg.SenderEmailAddress == "emailaddress"
):
pass
There is one more property called ReceivedTime
, so if you want to filter emails using the date and time when this email was received, this is how you can also use this property. Find more properties here and visit here for reading more solutions.
Complete Source Code:
# import win32com.client as client
# My_OL_App = client.Dispatch('Outlook.Application').GetNameSpace('MAPI')
# MY_Inbox=My_OL_App.GetDefaultFolder(6)
# MY_ib_items=MY_Inbox.Items
# Msg=MY_ib_items.GetLast()
# print(Msg.SenderName)
# print(Msg.Subject)
# print(Msg.body)
# Filter Emails With Properties
import win32com.client as client
My_OL_App = client.Dispatch("Outlook.Application").GetNameSpace("MAPI")
MY_Inbox = My_OL_App.GetDefaultFolder(6)
MY_Inbox = My_OL_App.Folders("your email@gmail.com").Folders(
"Inbox"
) # When you two or multiple mail boxes
MY_ib_items = MY_Inbox.Items
for msg in MY_ib_items:
if "n case you're wondering what's going on" in str(msg.Subject):
print(msg.SenderName)
print(msg.Subject)
print(msg.body)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn