Python Pipe
- What is the pipe() Method?
- Practical Applications of pipe()
- Error Handling with pipe()
- Conclusion
- FAQ
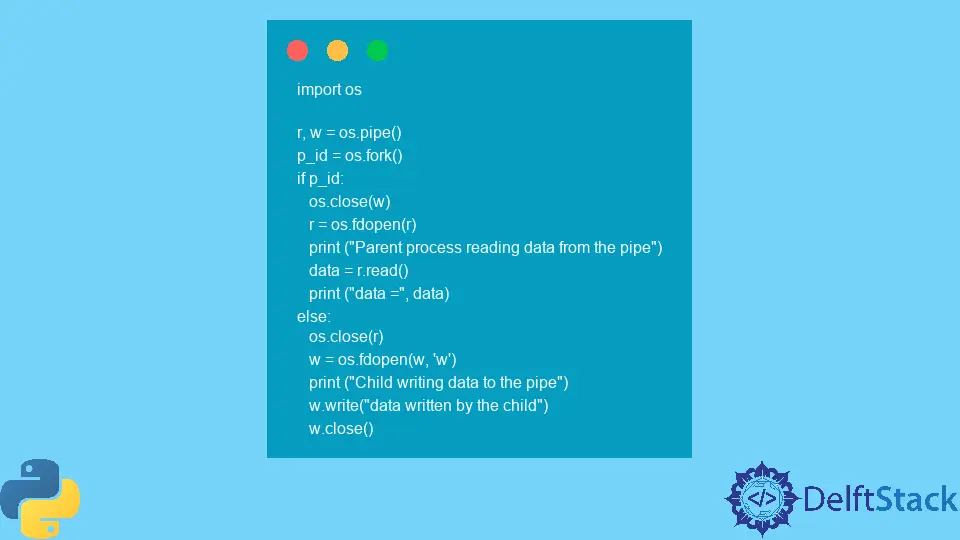
When working with Python, especially in scenarios that involve inter-process communication, the pipe()
method from the os
module is a powerful tool. This method allows you to create a unidirectional communication channel between processes, enabling data to flow seamlessly from one to another.
In this tutorial, we will delve into the intricacies of the pipe()
method, exploring its functionality and providing practical examples to illustrate its use. Whether you are a beginner or an experienced Python developer, this guide will enhance your understanding of how to effectively utilize the pipe()
method for your projects.
What is the pipe() Method?
The pipe()
method in Python’s os
module creates a pair of file descriptors: one for reading and one for writing. This is particularly useful in scenarios where you want to send data from one process to another without the need for intermediate files. The file descriptors returned by pipe()
can be used with other methods to read from or write to the pipe.
Example of Using pipe()
Let’s look at a simple example to illustrate how to use the pipe()
method effectively. In this example, we’ll create a pipe and write a message to it, which will then be read from the other end.
import os
read_fd, write_fd = os.pipe()
os.write(write_fd, b'Hello, World!')
os.close(write_fd)
message = os.read(read_fd, 1024)
os.close(read_fd)
print(message.decode())
Output:
Hello, World!
In this code snippet, we first import the os
module. We then create a pipe using os.pipe()
, which returns two file descriptors: read_fd
for reading and write_fd
for writing. We write a byte string to the pipe using os.write()
, close the writing end, and then read from the pipe using os.read()
. Finally, we decode the byte string and print the message. This example demonstrates the basic functionality of the pipe()
method, showcasing how data can be transferred between processes.
Practical Applications of pipe()
The pipe()
method is not just a theoretical concept; it has practical applications in various scenarios. One common use case is in the implementation of concurrent programs where processes need to communicate. For instance, if you are building a multi-threaded application, you can use pipes to send data between threads safely and efficiently.
Example of a Concurrent Application
Consider a scenario where you have a producer process that generates data and a consumer process that processes that data. Here’s how you might implement this using the pipe()
method:
import os
import time
import multiprocessing
def producer(write_fd):
for i in range(5):
message = f'Message {i}\n'
os.write(write_fd, message.encode())
time.sleep(1)
os.close(write_fd)
def consumer(read_fd):
while True:
message = os.read(read_fd, 1024)
if not message:
break
print(f'Consumed: {message.decode()}')
os.close(read_fd)
read_fd, write_fd = os.pipe()
p1 = multiprocessing.Process(target=producer, args=(write_fd,))
p2 = multiprocessing.Process(target=consumer, args=(read_fd,))
p1.start()
p2.start()
p1.join()
p2.join()
Output:
Consumed: Message 0
Consumed: Message 1
Consumed: Message 2
Consumed: Message 3
Consumed: Message 4
In this example, we define two functions: producer
and consumer
. The producer generates messages and writes them to the pipe, while the consumer reads from the pipe and processes the messages. We use the multiprocessing
module to create separate processes for each function. This setup allows for efficient communication between the producer and consumer, demonstrating the power of the pipe()
method in real-world applications.
Error Handling with pipe()
When working with pipes, it’s essential to implement error handling to manage potential issues that may arise during the read and write operations. This ensures that your application remains robust and can handle unexpected scenarios gracefully.
Example of Error Handling
Here’s how you can implement error handling in a pipe operation:
import os
try:
read_fd, write_fd = os.pipe()
os.write(write_fd, b'Test message')
os.close(write_fd)
message = os.read(read_fd, 1024)
if not message:
raise ValueError('No message received.')
print(message.decode())
except Exception as e:
print(f'An error occurred: {e}')
finally:
if 'read_fd' in locals():
os.close(read_fd)
Output:
Test message
In this example, we wrap our pipe operations in a try-except block to catch any exceptions that may occur. If no message is received, we raise a ValueError
. The finally block ensures that the file descriptor is closed, preventing resource leaks. This approach not only improves the reliability of your code but also enhances its maintainability.
Conclusion
Understanding the pipe()
method in Python is crucial for anyone looking to implement inter-process communication effectively. By creating a unidirectional channel for data transfer, you can streamline the way processes interact with each other. Through practical examples, we have explored how to use the pipe()
method, its applications in concurrent programming, and the importance of error handling. As you continue to develop your skills in Python, mastering the use of pipes will undoubtedly enhance your programming toolkit.
FAQ
-
What is the purpose of the pipe() method in Python?
The pipe() method creates a unidirectional communication channel between processes, allowing data to be transferred from one process to another. -
Can I use the pipe() method for multi-threaded applications?
While the pipe() method is primarily designed for inter-process communication, it can also be used in multi-threaded applications to facilitate communication between threads. -
How do I handle errors when using the pipe() method?
You can implement error handling using try-except blocks to catch exceptions that may arise during read and write operations, ensuring your application remains robust. -
Is the pipe() method suitable for large data transfers?
The pipe() method is best suited for smaller data transfers, as it has limitations on buffer sizes. For larger data, consider using other methods, such as files or sockets. -
Can I use the pipe() method on Windows?
Yes, the pipe() method is available on Windows as well as Unix-based systems, making it a versatile option for inter-process communication across different platforms.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn