How to Parse a YAML File in Python
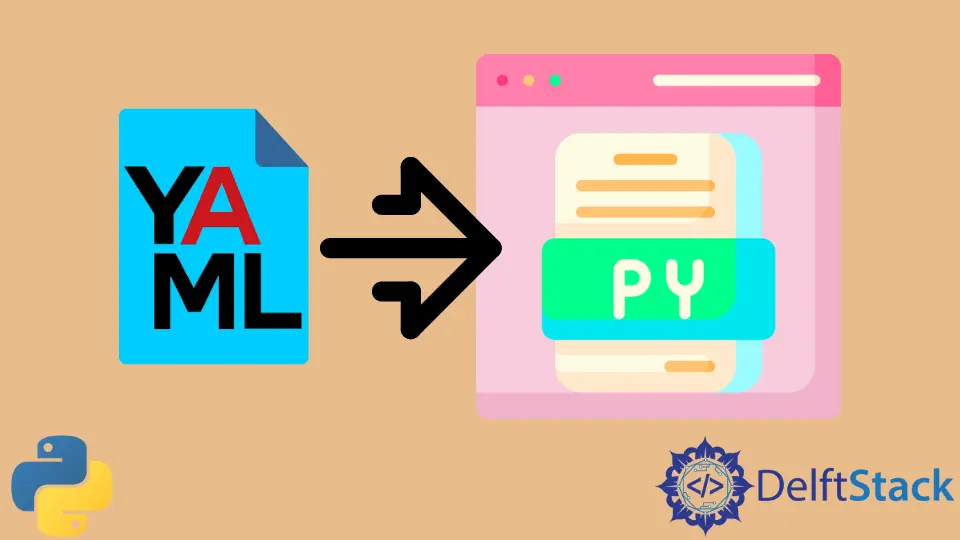
This tutorial will demonstrate different ways to parse a YAML file in Python.
YAML is a data serialization standard used as a file type to store configuration or property files within a project. YAML often replaces typical XML or JSON configuration files because files easier to serialize than both those file types.
Use the PyYAML
Package to Parse YAML in Python
pyyaml
is a Python package that provides utilities to parse and manipulate YAML files within Python.
The first step to do is to install pyyaml
using pip
since it isn’t readily available for Python.
pip install pyyaml
For Python 3.x users, use pip3
instead of pip
.
pip3 install pyyaml
Now the installation is done, proceed to parse a sample YAML file. A YAML file always will have three dashes ---
at the start of the file to denote the start of a new YAML document.
Note that spaces should exclusively be used when indenting YAML files since these files will not recognize tab spaces as indentations.
YAML files can have an extension of either .yaml
or .yml
.
sample.yaml
---
name: "John"
age: 23
isMale: true
hobbies:
- swimming
- fishing
- diving
To read the given YAML file in Python, import the yaml
module first. Afterward, open the yaml file using the function open()
. The yaml
module has a pre-defined function load()
, which accepts a file as an argument, loads a YAML file in Python, and converts it into a Python object data type.
import yaml
with open("sample.yaml", "r") as f:
print(yaml.load(f))
If you print the output of yaml.load()
, it will display the YAML file’s contents in a Python object format.
Output:
{'name': 'John', 'age': 23, 'isMale': True, 'hobbies': ['swimming', 'fishing', 'diving']}
To make sure that the YAML file exists and is not corrupted, safe to use, we can add a try
block and catch the exception using the built-in error object within the yaml
module YAMLError
. Another layer to make the code safer to use is to replace the load()
function with safe_load()
, which validates the YAML file before loading it.
import yaml
with open("sample.yaml", "r") as stream:
try:
print(yaml.safe_load(stream))
except yaml.YAMLError as exc:
print(exc)
This solution has the same output as the previous solution, although this is a much safer approach to parsing a YAML file in Python.
In summary, parsing a YAML file in Python is easily achievable by using the yaml
module. Make sure to perform pip install pyyaml
before using the module since it is not readily built-in.
It is always good practice to encapsulate file manipulation functions with try...except
blocks to make sure that file manipulation goes smoothly and is safe to perform.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn