How to Parse ISO 8601 Date in Python
- Structure of the ISO 8601 Date
-
Use
strptime()
andstrtime()
to Parse ISO 8601 Date in Python -
Use
fromisoformat()
to Parse ISO 8601 Date in Python -
Use
dateutil
to Parse ISO 8601 Date in Python
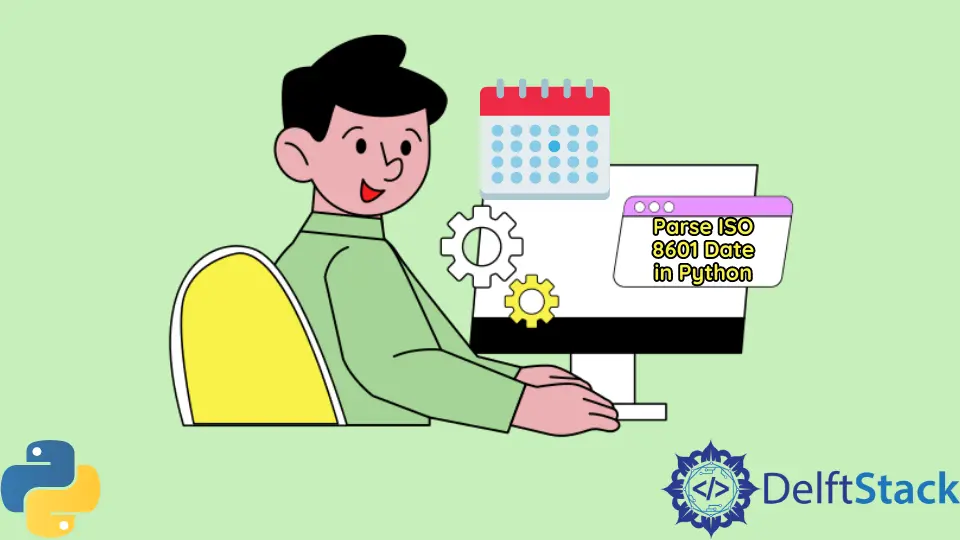
Dates are important to everyone and crucial to many applications we use. As a developer, it can be a little confusing with the different time zones or time objects that exist and working around them.
However, standards often exist that make the work easier, and that’s what this article entails. Moreover, we will discuss the structure of a typical ISO 8601 date and show you how to parse typical DateTime objects to the standardized DateTime that can be used all over.
Structure of the ISO 8601 Date
The ISO (International Organization for Standardization) works on creating standardization for its member countries across different fields, which often encompasses technical and non-technical areas. Areas such as engineering are part of their priorities.
The numerous standardizations include the ISO 8601 DateTime, represented by the month, day, hour, minutes, seconds, and milliseconds. It is used as the DateTime standard worldwide for any data and is time-based which helps prevent misrepresentation and errors.
If you are to build a Python application that is used worldwide, understanding the ISO 8601 DateTime object is important, and parsing it might be a requirement.
The ISO 8601 datetime is represented with the year starting at the left and ends with a millisecond parameter and is often combined with other computer characters from T
to Z
.
2022-08-10 17:00:00.000
The above ISO DateTime object represents the 11th day of August in the year 2022 at 5 p.m. within the local time zone.
It is important to state the ISO 8601 DateTime string requires the date section, but the time section is optional. They are different variants of ISO 8601, but the above would be the reference of this article.
Use strptime()
and strtime()
to Parse ISO 8601 Date in Python
Python comes pre-installed with different modules that are useful for different operations, and one of those pre-installed modules is datetime
, which helps with date and time operations. With the datetime
module, you can obtain datetime(year, month, day[, hour[, minute[, second[, microsecond[,tzinfo]]]]])
values.
The now()
method helps us to get and develop the current date and time and gives the typical DateTime object that we can manipulate or parse to get whatever information we need.
from datetime import datetime
todaysDate = datetime.now()
print(todaysDate)
Output:
2022-09-28 06:55:56.832983
Also, the today()
method can be used to achieve the same value. Although, the now()
method is preferred to the today()
method.
AnoTodaysDate = datetime.today()
Output:
2022-09-29 10:48:43.311880
Before, we parse the typical Python DateTime object to the standardized ISO 6801 DateTime. Let’s reverse this by creating the ISO 6801 DateTime with the Python DateTime object.
To achieve this, we need the strftime()
method, which helps us to convert DateTime objects to their string representation - this time, the ISO 6801 DateTime string.
from datetime import datetime
todaysDate = datetime.now()
todaysDateInISO = todaysDate.strftime("%Y-%m-%dT%H:%M:%S.%f%z")
print(todaysDateInISO)
Output:
2022-09-29T11:08:29.413183
The todaysDate
binding holds the Python DateTime object for the current date and time, and then we pass the binding as an argument to the strftime()
method to create the ISO 8601 DateTime string equivalent.
With these now, how do we convert the ISO 8601 DateTime string to a Python DateTime object? We need a method that does the opposite of the strftime()
method, the strptime()
.
This method creates a DateTime object from a DateTime string.
Therefore, we will pass an ISO 6801 DateTime string as the first argument of the strptime()
and another argument that defines the date format you want - the Python DateTime object %Y-%m-%dT%H:%M:%SZ
.
parsedDate = datetime.strptime("2022-03-04T21:08:12Z", "%Y-%m-%dT%H:%M:%SZ")
print(parsedDate)
Output:
2022-03-04 21:08:12
The Y-%m-%dT%H:%M:%SZ
date format processes the year, month, day, hour, minute, and second using the format codes specified within the Python DateTime object.
Use fromisoformat()
to Parse ISO 8601 Date in Python
Similar to the strftime()
and strptime()
methods, there are dedicated methods that can create ISO 6801 DateTime strings or convert them to Python DateTime objects. The isoformat()
method helps return the time formatted according to ISO, which is processed in the YYYY-MM-DD HH:MM:SS.mmmmmm
format.
And more importantly, the fromisoformat()
method takes the ISO 8601 DateTime string and returns the Python DateTime object equivalent.
To create an ISO 8601 from a Python DateTime object, you can first create the DateTime object using the now()
method and obtain the ISO 8601 property (format) of the result using isoformat()
.
from datetime import datetime
todaysDate = datetime.now()
todaysDateInISO = todaysDate.isoformat()
print(todaysDateInISO)
Output:
2022-09-28T07:46:13.350585
The result often contains a separator between the date and time. Here, it is T
and can be changed. You can change the separator by passing an argument.
todaysDateInISO = todaysDate.isoformat("$")
print(todaysDateInISO)
Output:
2022-09-28$07:48:11.060212
Now to the crux of the matter, we can parse the ISO 8601 DateTime string to a typical Python DateTime object.
parsedDate = datetime.fromisoformat(todaysDateInISO)
print(parsedDate)
Output:
2022-09-29 14:38:17.928655
Use dateutil
to Parse ISO 8601 Date in Python
The dateutil
Python module is a third-party module that you can use to extend the functionalities accessible via the built-in datetime
module.
To install it, you can utilize the pip
command:
pip install python-dateutil
Output:
Collecting python-dateutil
Downloading python_dateutil-2.8.2-py2.py3-none-any.whl (247 kB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 247.7/247.7 kB 506.3 kB/s eta 0:00:00
Collecting six>=1.5
Downloading six-1.16.0-py2.py3-none-any.whl (11 kB)
Installing collected packages: six, python-dateutil
Successfully installed python-dateutil-2.8.2 six-1.16.0
In addition, you can also introduce time zone functionalities using another third-party module called pytz
, which can be installed using the pip
command.
pip install pytz
These libraries can easily create an ISO 8601 date format based on a time zone and parse the ISO 8601 DateTime string to the typical Python DateTime object.
For the example, we will make use of the US/Eastern
time zone and create an ISO 8601 DateTime string using the timezone()
method, the now()
method, and the isoformat()
method.
from datetime import datetime
import pytz
usEasternDate = datetime.now(pytz.timezone("US/Eastern"))
print(usEasternDate)
usEasternDateISO = usEasternDate.isoformat()
print(usEasternDateISO)
Output:
2022-09-29 10:01:17.654150-04:00
2022-09-29T10:01:17.654150-04:00
Then to parse the ISO 8601 DateTime string to the Python DateTime object, we can use the parse()
method with the ISO date passed as its argument.
parsedDate = parser.parse(usEasternDateISO)
print(parsedDate)
Output:
2022-09-29 10:01:17.654150-04:00
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedIn