How to Use MD5 Hash in Python
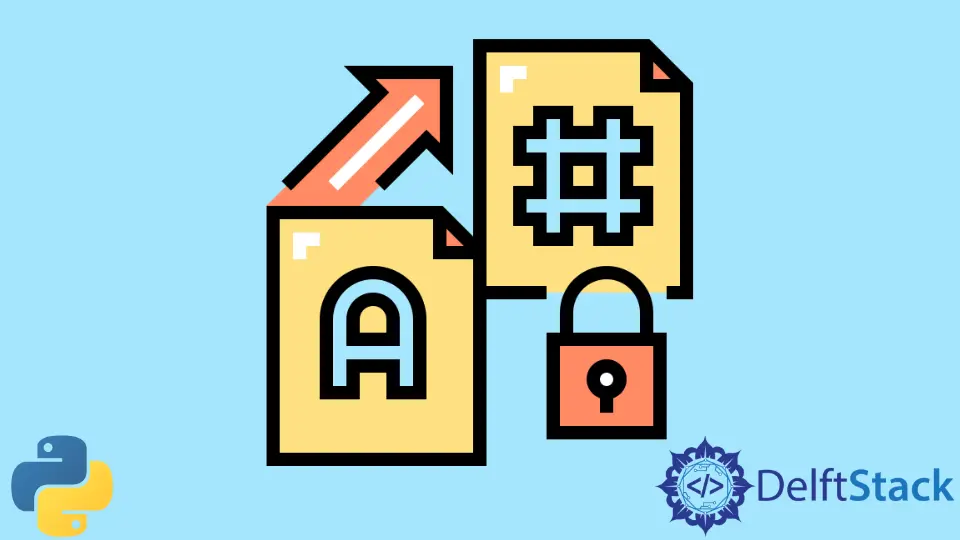
This article will demonstrate how to use the MD5 hash using the Python hashlib
module.
What Is Hash?
A hash is a function that takes data of variable length and converts it to a fixed length. The value returned from a hash function is called a hash value, hash code, or digest. The hash values are usually used to index a fixed-sized table called a hash table.
What Is MD5?
MD5 message-digest algorithm is a popular hash function that produces a 128-bit hash value. Initially designed as a cryptographic hash function, it is now commonly used to verify data integrity due to multiple security issues and vulnerabilities.
Python hashlib
Module
The Python standard library consists of a module that implements many different secure hash and message digest algorithms called hashlib
. It may also include additional algorithms available depending on the OpenSSL library that Python uses on your platform.
To use the hashing algorithms, import the hashlib
module.
import hashlib
We can now use the hashing algorithms supported by this module. To check the available hash algorithms in the running Python interpreter, use the constant attribute algorithms_available
.
import hashlib
print(hashlib.algorithms_available)
Output:
{'md5', 'blake2s', 'sha256', 'sha384', 'sha3_512', 'blake2b', 'md4', 'md5-sha1', 'sha512_224', 'sha224', 'sha3_224', 'ripemd160', 'sha3_256', 'shake_256', 'sm3', 'shake_128', 'sha3_384', 'sha1', 'sha512_256', 'whirlpool', 'sha512'}
The list above are the available algorithms in hashlib
, including available algorithms of OpenSSL
To check the hash algorithms guaranteed to be supported on all platforms by this module, use the constant attribute algorithms_guaranteed
.
import hashlib
print(hashlib.algorithms_guaranteed)
Output:
{'sha3_256', 'sha256', 'sha3_224', 'sha224', 'blake2s', 'sha3_512', 'shake_128', 'sha512', 'sha3_384', 'shake_256', 'md5', 'blake2b', 'sha384', 'sha1'}
md5
is in the list of algorithms_guaranteed
, but some FIPS compliant upstream vendors offer a Python build that excludes it.Use the MD5 Algorithm in Python
To use the md5 algorithm, we will use the md5()
constructor and feed the hash object with byte-like objects using the update()
method or pass the data as a parameter of the constructor.
To obtain the hash value, use the digest()
method, which returns a bytes
object digest of the data fed to the hash object.
import hashlib
md5_hash = hashlib.md5()
md5_hash.update(b"Hello World")
print(md5_hash.digest())
Output:
b'\xb1\n\x8d\xb1d\xe0uA\x05\xb7\xa9\x9b\xe7.?\xe5'
You can also pass the data as a parameter to the constructor and obtain the hash value
import hashlib
print(hashlib.md5(b"Hello World").digest())
Output:
b'\xb1\n\x8d\xb1d\xe0uA\x05\xb7\xa9\x9b\xe7.?\xe5'
Similar to the digest()
method, you can also use hexdigest()
, which returns a string object of the digest containing only hexadecimal digits.
import hashlib
md5_hash = hashlib.md5()
md5_hash.update(b"Hello World")
print(md5_hash.hexdigest())
Output:
b10a8db164e0754105b7a99be72e3fe5
Notice that there is a b
before the string literal passed to the update()
method. It is used to create an instance of type bytes
instead of type str
. As the hashing function only accepts a sequence of bytes as a parameter. Passing string objects to the update()
method is not supported.
You can also do multiple calls to the update()
method, which is equivalent to a single call with all the arguments being concatenated.
import hashlib
first_hash = hashlib.md5()
first_hash.update(b"Hello World, Hello Python")
print(first_hash.hexdigest())
second_hash = hashlib.md5()
second_hash.update(b"Hello World,")
second_hash.update(b" Hello Python")
print(second_hash.hexdigest())
Output:
b0f2921c8c0e63898b0388777908893a
b0f2921c8c0e63898b0388777908893a
In summary, we can use the md5
hash algorithm through the hashlib
module that can be fed with data by passing it as a parameter of the md5()
constructor or using the update()
method. We can obtain the hash value using the digest()
method, which returns a bytes
object of the digest()
or hexdigest()
method, which returns a string object of the digest containing only hexadecimal digits.