How to List of Numbers From 1 to N in Python
- How To Create a List of Numbers from 1 to N in Python Using a User-Defined Function
-
How To Create a List of Numbers from 1 to N in Python Using the
range()
Function -
How To Create a List of Numbers from 1 to N in Python Using the
numpy.arange()
-
How To Create a List of Numbers from 1 to N in Python Using
list()
andmap()
-
How To Create a List of Numbers from 1 to N in Python Using a
while
Loop - FAQ
- Generate a Python List from 1 to N of Conclusion
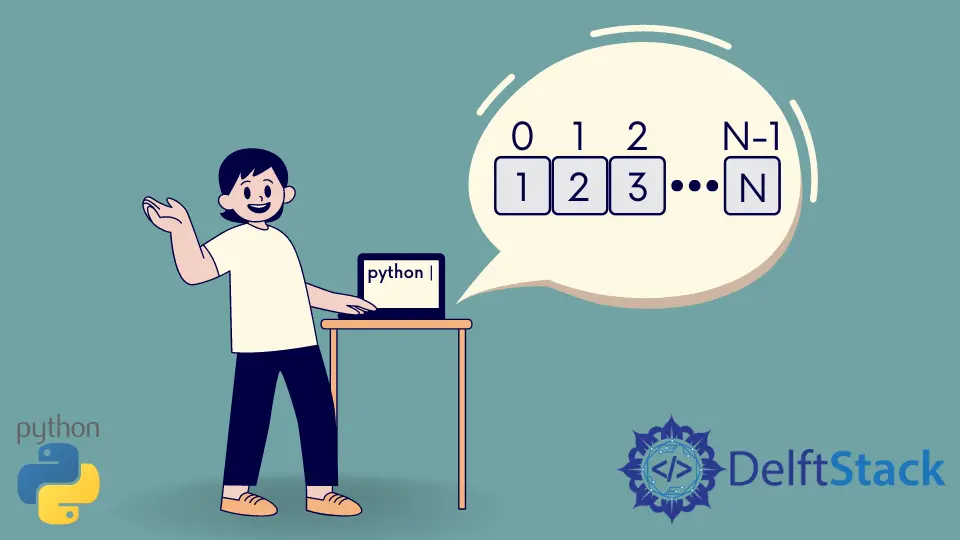
Whether you’re a Python beginner or looking for different ways to expand your coding toolkit, creating a list of numbers from 1 to a specified value is a fundamental skill.
In Python, there are multiple approaches to generate a list of numbers ranging from 1 to n.
One common method utilizes the built-in range
function, which takes up to three arguments: start value, end value (n+1
for an inclusive range from 1 to n), and an optional step value, allowing the specification of incrementing patterns.
Alternatively, the NumPy module, a powerful numerical computing library, offers a concise way to achieve the same result by utilizing its numpy.arange
function. This function also accepts three arguments—start, stop (n+1
), and step values—and returns a NumPy array containing the desired sequence.
Whether you opt for the simplicity of the Python built-in range
or the versatility of NumPy, these methods exemplify the flexibility and ease with which you can generate lists of numbers in Python.
In this article, we’ll explore various methods, from simple and classic to more advanced and efficient approaches.
How To Create a List of Numbers from 1 to N in Python Using a User-Defined Function
In Python, you can easily generate a list of numbers from 1 to N using a specified value by creating a user-defined function. This method involves defining a function that takes the desired number, iterates up to that value using a for
loop, and appends each number to a list.
Let’s start by defining this function and breaking down its components:
def create_number_list(end_number):
number_list = [] # Initialize an empty list to store the numbers
for i in range(1, end_number + 1):
number_list.append(i) # Append each number to the list
return number_list # Return the final list
Where:
end_number
: The parameter representing the desired end number.number_list
: An empty list initialized to store the generated numbers.for i in range(1, end_number + 1)
: Afor
loop that iterates from 1 to the specified end number (inclusive).number_list.append(i)
: Each iteration appends the current numberi
to the list.return number_list
: The function returns the final list of numbers.
Now, let’s put the function into action with an example.
Code Input:
def create_number_list(end_number):
number_list = [] # Initialize an empty list to store the numbers
for i in range(1, end_number + 1):
number_list.append(i) # Append each number to the list
return number_list # Return the final list
result_list = create_number_list(12)
print(result_list)
Here, the create_number_list
function is defined with the parameter end_number
, representing the upper limit of the desired range. Inside the function, a list named number_list
is initialized.
The for
loop iterates from 1 to end_number
(inclusive). In each iteration, the current value of i
is appended to the list using number_list.append(i)
.
Finally, the function returns the completed list. The example usage at the end demonstrates calling the function with an argument of 10
, and the resulting list is printed.
Code Output:
The output of the provided code will be a list of numbers from 1 to 12.
This result is obtained by calling the create_number_list
function with an argument of 12
. The function generates a list containing numbers from 1 to 12 (inclusive), and the printed output shows the resulting list.
How To Create a List of Numbers from 1 to N in Python Using the range()
Function
The range()
function is a built-in Python function that generates a sequence of numbers within a specified number or range. Its basic syntax is as follows:
range(start, stop, step)
Where:
start
: The starting value of the sequence (optional, default is 0).stop
: The end value of the sequence (not inclusive).step
: The step size between numbers in the sequence (optional, default is 1).
Let’s take a simple example of creating a list of numbers from 1 to 10.
Code Input:
numbers = list(range(1, 13))
print(numbers)
In this example, range(1, 11)
generates a sequence of numbers starting from 1 and stopping before 11. The list()
function is then used to convert this sequence into a list, which is stored in the variable numbers
.
Code Output:
The output [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
is produced because the range(1, 13)
function generates a sequence of numbers starting from 1 up to, but not including, 13.
You can also customize the sequence by adjusting the start
, the stop
value, and step
parameters of the range()
function.
Code Input:
even_numbers = list(range(2, 11, 2))
print(even_numbers)
Code Output:
In this example, range(2, 11, 2)
generates a sequence of even numbers starting from 2, stopping before 11, and with a step size of 2.
Moreover, you can also take user input to determine the end value for the sequence.
Code Input:
end_value = int(input("Enter the end value for the sequence: "))
numbers = list(range(1, end_value + 1))
print(numbers)
Code Output:
List comprehension also provides a concise way to create lists in Python. The following example demonstrates using list comprehension with the range()
function.
Code Input:
number_list = [i for i in range(1, 12 + 1)]
print(number_list)
This one-liner achieves the same result as the previous example. It iterates through the range(1, 10 + 1)
sequence, appending each value to the list.
The output remains [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
.
Code Output:
This code uses a list comprehension to generate a list of numbers from 1 to 12 (inclusive) and then prints the resulting list.
How To Create a List of Numbers from 1 to N in Python Using the numpy.arange()
When it comes to numerical computing and working with arrays in Python, the NumPy library stands out as a powerful and efficient tool. NumPy provides a function called arange()
that enables you to create a list of numbers with regularly and evenly spaced values within a specified range.
Its syntax is similar to that of the built-in range()
function but offers more flexibility and capabilities.
import numpy as np
np.arange(start, stop, step)
Where:
start
: The starting value of the sequence.stop
: The end value of the sequence (not inclusive).step
: The step size between numbers in the sequence.
Let’s begin with a simple example of creating a list of numbers from 1 to 10.
Code Input:
import numpy as np
numbers = np.arange(1, 13)
print(numbers)
Code Output:
In this example, np.arange(1, 11)
generates an array of numbers starting from 1 and stopping before 11. The result is stored in the variable numbers
.
Similar to the range()
function, you can customize the sequence by adjusting the start
, stop
, and step
parameters of numpy.arange()
.
Code Input:
import numpy as np
even_numbers = np.arange(2, 11, 2)
print(even_numbers)
Code Output:
Here, np.arange(2, 11, 2)
generates an array of even numbers starting from 2, stopping before 11, and with a step size of 2.
Unlike the range()
function, numpy.arange()
allows you to use non-integer step sizes.
Code Input:
import numpy as np
numbers_with_float_step = np.arange(0, 1.1, 0.1)
print(numbers_with_float_step)
Code Output:
This example generates an array of numbers from 0 to 1 (inclusive) with a step size of 0.1.
How To Create a List of Numbers from 1 to N in Python Using list()
and map()
Another approach to generating lists of numbers in Python involves using the built-in list()
function along with the map()
function. This combination provides a concise and elegant way to successfully create a list of numbers from 1 to a specified value.
The list()
function in Python is a built-in function that can convert an iterable (such as a sequence or range object) into a list. The map()
function, on the other hand, applies a given function to all items in an iterable (or iterables) and returns an iterable map object.
When combined, these functions can be a powerful tool for creating lists with a specified structure.
Let’s start with a simple example of creating a list of numbers from 1 to 10 using the list()
and map()
functions.
Code Input:
numbers = list(map(int, range(1, 13)))
print(numbers)
Code Output:
In this example, range(1, 11)
generates a sequence of numbers from 1 to 10. The map(int, ...)
part applies the int()
function to each element of the sequence, ensuring that all elements are integers.
Finally, the list()
function converts the resulting iterable map object into a list, which is stored in the variable numbers
.
Also, you can easily customize the sequence by adjusting the parameters of the range()
function. For example, creating a list of even numbers from 2 to 10.
Code Input:
even_numbers = list(map(int, range(2, 16, 2)))
print(even_numbers)
Code Output:
Here, range(2, 11, 2)
generates a sequence of even numbers, and the map(int, ...)
ensures that all elements are integers.
Similar to the previous example, you can also take user input to determine the end value for the sequence.
Code Input:
end_value = int(input("Enter the end value for the sequence: "))
numbers = list(map(int, range(1, end_value + 1)))
print(numbers)
Code Output:
This example prompts the user to input the end value for the sequence, and then it generates the list accordingly.
How To Create a List of Numbers from 1 to N in Python Using a while
Loop
The while
loop in Python is a fundamental control flow structure that allows you to execute a particular code block repeatedly as long as a specified condition is true. Leveraging the while
loop is an effective way to create a list of numbers from 1 to a specified value.
Let’s start with a basic example of using the while
loop to generate a list of numbers from 1 to 10.
Code Input:
start = 1
end_value = 12
numbers = []
while start <= end_value:
numbers.append(start)
start += 1
print(numbers)
Code Output:
In this example, we begin by initializing the start
variable to 1 and the end_value
variable to 10. Additionally, an empty list called numbers
is created.
The while
loop is then employed to iteratively append the current value of start
to the numbers
list as long as the condition start <= end_value
remains true.
Within the loop, the start
variable is incremented by 1 in each iteration. This process effectively generates a list of numbers from 1 to 10.
You can also easily customize the sequence by adjusting the initial values and the loop condition. Take a look at the following code example.
Code Input:
start = 2
end_value = 10
even_numbers = []
while start <= end_value:
even_numbers.append(start)
start += 2
print(even_numbers)
Code Output:
Similar to the first example, we initialize the start
variable to 2, as we want to start with the first even number. The end_value
is set to 10, and an empty list called even_numbers
is created.
The while
loop appends the current value of start
to the even_numbers
list and increments the start
value by 2 in each iteration. This ensures that only even numbers are included in the final list.
You can also take user input (a specific number) to determine the end value for the sequence.
Code Input:
end_value = int(input("Enter the end value for the sequence: "))
start = 1
user_sequence = []
while start <= end_value:
user_sequence.append(start)
start += 1
print(user_sequence)
Code Output:
This example prompts the user to input the end value for the sequence. The end_value
variable is assigned the user’s input, and a new list called user_sequence
is initialized.
The while
loop, similar to the first example, appends the current value of start
to the user_sequence
list while incrementing start
by 1 in each iteration.
FAQ
Q1: How do you create a list of numbers from 1 to N in Python?
A1: To create a list of numbers from 1 to N in Python, you can leverage the range
function or use a list comprehension method.
Q2: Why do we use N + 1
in the range function or list comprehension?
A2: Yes, you can also use a loop, create a list, or other built-in functions like numpy.arange
to create a similar list.
Q3: What is the significance of creating a list from 1 to N in Python?
A3: Generating such a list is a common task in programming for various applications, including data manipulation, algorithm implementation, and numerical analysis.
Generate a Python List from 1 to N of Conclusion
Python offers a rich set of tools for creating lists of numbers, allowing you to choose the most suitable method based on your specific requirements, whether it’s through user-defined functions, built-in functions, libraries like NumPy, or the classic while
loop, Python’s flexibility shines in its ability to accommodate diverse preferences and coding styles.
By understanding these different approaches and examples, you can enhance your proficiency in list generation optimizing code for readability, efficiency, and functionality.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn