How to Decompress Gzip in Python
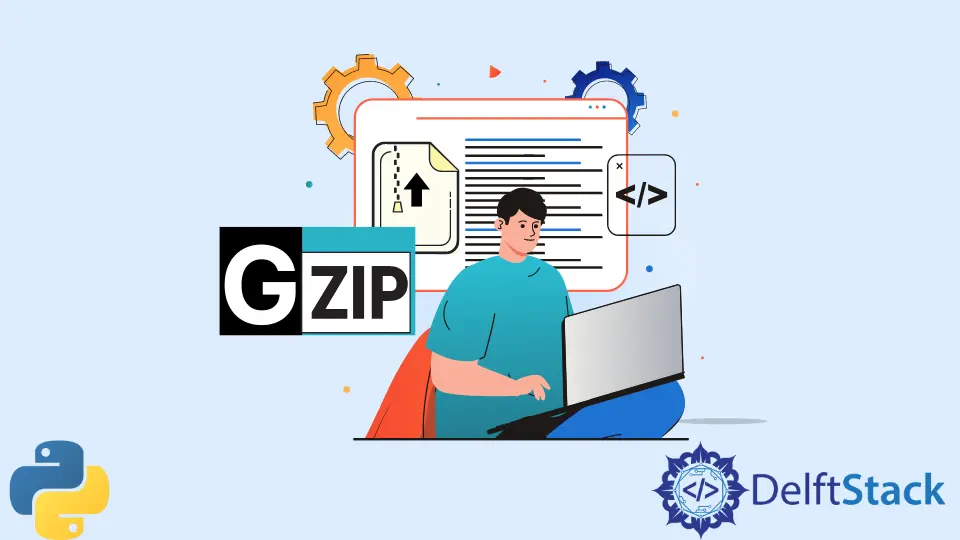
We will introduce the gzip decompression in Python. We will also introduce how we can decompress compressed content using gzip decompression.
Gzip Decompression in Python
Many libraries are built for compression and decompression purposes in Python, but we will introduce the Gzip
library. It is a popular data compression tool.
We can use gzip
to reduce the size of a file by encoding the data in a special format that cannot be read by humans and is hard to compress. We can use the gzip.decompress()
method to decompress a string’s compressed bytes into an original string.
There are two types of data compression methods in gzip
. We will discuss both methods in detail.
The first method is known as inverse compression. It is a special type of Huffman coding that can be used to reduce the size of the data.
The other method is gzip decompression, which we will use in the example. It decompresses a string’s compressed bytes into an original string.
The syntax of the gzip decompression method is shown below.
# python
gzip.decompress(stringToDecompress)
Now, let’s use this function in our examples. First, we need to install the library using the following command.
# python
pip install gzip
Once the Gzip
library is installed, we can import it using the following line of code.
# python
import gzip
Let’s start with an example, as shown below.
# python
import gzip
value = b"This string is encoded in Python."
value = gzip.compress(value)
print("compressed value is: ", value)
compressed = gzip.decompress(value)
print("Decompressed value is: ", compressed)
Output:
The example above shows that when the string is compressed using gzip
, it is encoded into a non-readable format. But when we use gzip decompression, it is decoded and converted into human-readable format.
Zlib
Library in Python
Now we will discuss another library, Zlib
, that can also be used for compression and decompression. The Zlib
library is one of Python’s most common and useful compression libraries.
The Zlib
provides some great, easy-to-use compression and decompression algorithms functions. Let’s install this library and use it in our examples.
We can easily install the library using the following command.
pip install zlib
Once the Zlib
library is installed, we can import it using the following line of code.
# python
import zlib
The Zlib
library provides some functions for compression and decompression. In this tutorial we will use gzip()
for encoding and zlib.decompress()
for decoding.
As shown below, let’s go through an example in which we will use this library.
# python
import zlib
value = b"This string is encoded in Python."
Compressed = zlib.compress(value)
print("Compressed String is ")
print(Compressed)
print("\nDecompressed String is")
print(zlib.decompress(Compressed))
Output:
The example above shows multiple libraries available for the compression and decompression of strings.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn