How to Use Global Variables Across Multiple Files in Python
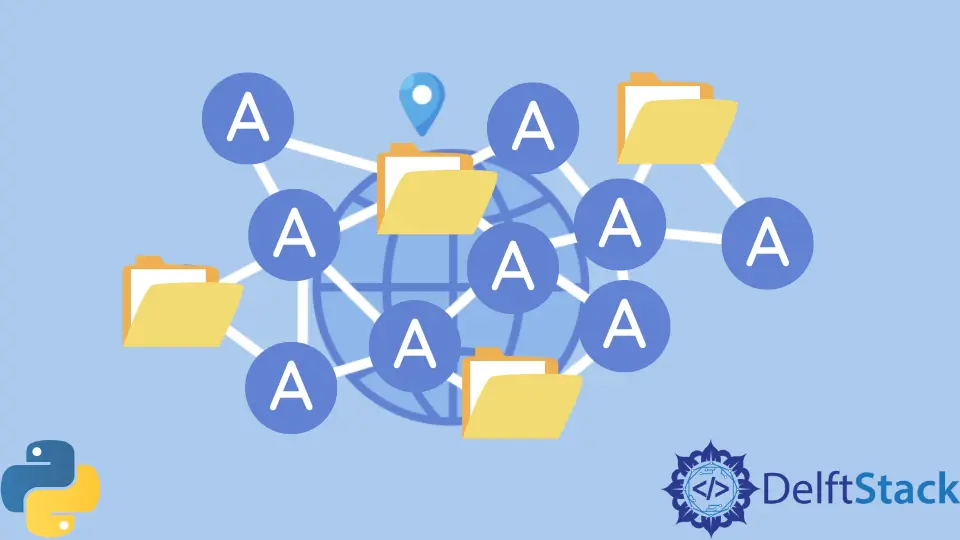
This trivial guide is about using global variables across multiple files in Python. But before jumping into the main topic, let’s briefly look at global variables and their uses in multiple files.
Global Variables in Python
Global variables are the variables that are not a part of the function scope and can be used globally across the program. This suggests that the global variables can also be used within or outside the function body.
Let’s look at an example:
x = "my global var"
def fun1():
print("Inside fun1(): ", x)
fun1()
print("In global context: ", x)
We have defined a global variable x
in this code and assigned it some value. Then, we printed the x
variable inside and outside the function to see the value in both the scope.
Output:
The output suggests that the variable’s value is the same inside and outside the function. If we need to change the value of a global variable in some local scope, like in a function, then we need to use the keyword global
when declaring a variable.
Use Global Variables Across Multiple Files in Python
If we are using multiple files for our program and these files need to update the variable, then we should declare the variable with a global
keyword like this:
global x
x = "My global var"
Consider an example scenario where we have to deal with multiple Python code files and a global variable of the list of students. The resource.py
file has a global list of the students, and the prog.py
has a method to append students to this global list.
We can realize the concept using the following code:
Code- resource.py
:
def initialize():
global students
students = []
Code- prog.py
:
import resource
def addStudent():
resource.students.append("John")
resource.students.append("Dave")
Code- Main.py
:
import resource
import prog
resource.initialize()
prog.addStudent()
print(resource.students[0])
print(resource.students[1])
In the first resource.py
file, we have defined a function in which we declare a list studentList
and initialize it to an empty list. In the next file (i.e., prog.py
), we have included the resource
module and then defined a function addStudent
, in which we have appended two objects in the global list studentList
.
In the main file Main.py
, we have included both modules, resource
and prog
. Later, we called the function initialize
and addStudent
of both modules, respectively.
Afterward, when we printed the list indexes, we got the following output:
Thus, we can use the global
keyword to define a global variable in a Python file for use across the other files. Now, to access the global variable of a file in another file, import the file having the global variable as a module in the other file and directly access any global variable of the imported module without additional complexities.