How to Exit the if Statement in Python
-
How to Exit an
if
Statement in Python Usingbreak
-
How to Exit an
if
Statement in Python Using thereturn
Statement Within a Function -
How to Exit an
if
Statement in Python Usingsys.exit()
-
How to Exit an
if
Statement in Python Using a Flag Variable -
How to Exit an
if
Statement in Python UsingTry/Except
-
How to Exit an
if
Statement in Python FAQ -
How to Exit an
if
Statement in Python Conclusion
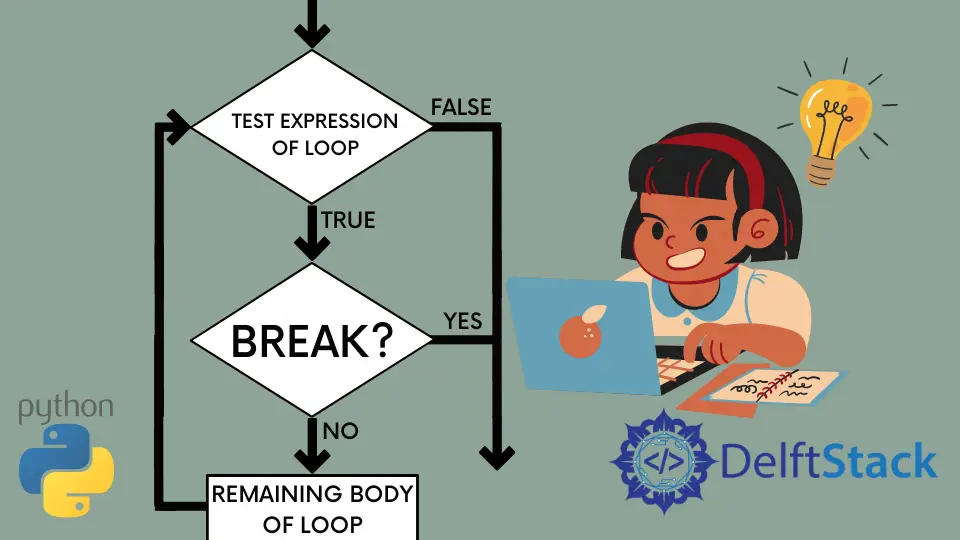
In Python, the if
statement is a fundamental control structure that allows you to execute a block of code conditionally. There are scenarios where you might want to exit an if
statement early, either without executing the rest of the code within the if
block or without proceeding to subsequent elif
or else
blocks.
This article will discuss the various methods to exit an if
statement in Python.
How to Exit an if
Statement in Python Using break
The break
statement is often used to exit loops (e.g., for
, while
) prematurely when a certain condition is met. However, if you have a loop inside an if
statement and you want to exit the if
block and the loop simultaneously, you can use the break
keyword effectively.
Code Input
def example_function(condition):
if condition:
while True:
user_input = input(
"Do you want to exit the loop and the if block? (yes/no): "
)
if user_input.lower() == "yes":
print("Exiting the loop and the if block.")
break # Exit the loop and the if block
else:
# Continue the loop or additional logic within the if block
print("Continuing the loop.")
# Usage
example_function(True)
In this example, we have an if
block that contains a while
loop. The break
statement is used inside the if
block to exit both the while
loop and the if
statement when the user inputs yes
.
Code Output:
Here, we used the following input: no
, no
, and yes
. Whenever the input was no
, Continuing the loop.
is printed on the console and the user is asked for a new input.
Once we used yes
as input, the code breaks and the Exiting the loop and the if block.
message is printed on the console.
Exit Multiple Nested if
Statements With break
break
can also be used to exit multiple levels of nested if
statements when combined with labeled loops. This approach allows for a more complex control flow.
Code Input:
def example_function(condition1, condition2):
if condition1:
while True:
if condition2:
print("Exiting both if statements.")
break # Exit
else:
# Additional logic within the inner if block
pass
# Additional logic outside the inner if block
else:
# Rest of the code if condition1 is not met
pass
# Usage
example_function(True, True)
Code Output:
In the above example, the break
statement is used to exit both the outer and inner if
statements based on conditions, effectively ending the loop and the if
block.
How to Exit an if
Statement in Python Using the return
Statement Within a Function
While the return
statement is primarily used to return a value from a function, it can also be employed to exit an if
or nested if
statement when placed within a function.
To do this, we can enclose the logic inside a function and use the return
to exit the function whenever we wish to break out of the conditional branches.
Let’s modify the previous example accordingly.
Code Input:
def something(i):
if i % 2 == 0:
if i == 0:
return
if i > 0:
print("even")
if __name__ == "__main__":
something(0)
print("Broken out")
Code Output:
In this modified example, we’ve encapsulated the nested if
statement inside the something
function.
When the conditions are met, we use the return
to exit the function, effectively bypassing any further code execution within the function.
It’s advisable to adhere to Python’s conventional approach and use the return
within functions to exit from if
statements, ensuring code readability, maintainability, and compatibility with standard practices.
How to Exit an if
Statement in Python Using sys.exit()
The sys.exit()
is another method to exit an if
block and terminate the entire Python script. This is part of the sys
module.
Here’s a simple example demonstrating how to use sys.exit()
to exit an if
statement.
Code Input:
import sys
# Some condition
condition = True
if condition:
print("Condition is True, exiting...")
sys.exit()
# Code after the if statement will not be executed if sys.exit() is called.
print("This will not be printed.")
Code Output:
In this example, if the condition
is true, the script will print a message and exit using sys.exit()
. The message after the sys.exit()
call will not be printed because the script terminates before reaching that point.
How to Exit an if
Statement in Python Using a Flag Variable
A flag variable is a Boolean variable that is used to control the flow of a program. It acts as a signal or indicator, determining when a specific condition is met or when certain actions should be taken.
In the context of exiting an if
statement, a flag variable can be used to signal when to prematurely exit the if
block.
Here are the steps to achieve this:
-
Begin by initializing a flag variable, usually set to
False
. The flag will be used to indicate whether the condition to exit theif
statement is met.
exit_flag = False
-
Inside the
if
statement, evaluate the condition that determines whether theif
block should be exited. If the condition is met, set the flag variable toTrue
and perform any necessary actions.
if condition_to_exit:
exit_flag = True
# Perform actions when exiting the if statement
# ...
-
After the
if
statement, check the flag variable. If it’sTrue
, exit the current scope using areturn
,break
, or another appropriate control statement.
if exit_flag:
return # or use break or other appropriate exit statement
Let’s illustrate this with a simple example.
Code Input:
def process_data(data):
exit_flag = False
for item in data:
if item == "exit_condition":
exit_flag = True
break # Exit the loop
# Process the item
print("Processing item:", item)
if exit_flag:
return # Exit
# Sample data
data = ["item1", "item2", "exit_condition", "item3", "item4"]
# Process the data
process_data(data)
Code Output:
In this example, the process_data
function iterates over a list of items. If it encounters an item that matches the exit condition, it sets the flag to True
and exits the loop and the function.
How to Exit an if
Statement in Python Using Try/Except
In Python, the try/except
construct is not typically used to exit an if
statement but rather to handle exceptions — unexpected errors that occur during execution. However, in some unique cases, this method can be creatively applied to exit from an if
instruction, especially when dealing with operations that might raise exceptions.
Here’s a simple example demonstrating how to use Try/Except
to exit an if
statement.
Code Input:
def check_divisibility(number, divisor):
try:
if divisor == 0:
raise ValueError("Divisor cannot be zero")
result = number % divisor
return result == 0
except ValueError as e:
print("Error:", e)
return False
# Test the function
number = 10
divisor = 0
is_divisible = check_divisibility(number, divisor)
print("Is divisible:", is_divisible)
Code Output:
In this example, the function check_divisibility
checks whether a number is divisible by a given divisor. The if
instruction inside the try block checks if the divisor is zero and raises a ValueError
if it is.
The except block catches this specific error, prints an error message, and returns False
. This method effectively exits the if
segment when encountering a zero divisor, which would otherwise lead to a division-by-zero error.
How to Exit an if
Statement in Python FAQ
Q1: What Are the Different Methods to Exit an if
Statement in Python?
A1: Python provides several methods to exit an if
segment, including the use of keywords like break
within loops, the return
statement, the sys.exit()
method, and the implementation of a flag
variable.
Q2: Can I Exit an if
Statement and a Loop Simultaneously in Python?
A2: Yes, it’s possible to exit both an if
instruction and a loop in Python simultaneously. One common approach is to use the break
syntax within a loop inside the if
block, preventing the execution from reaching any subsequent else
block.
Q3: How Does the Python Exit if
Statement Approach Contribute to Efficient Code Flow Control?
A3: The Python exit if
statement approach is essential for optimizing code flow control. It not only enhances code readability but the method also ensures that the execution flow aligns with the desired logic, contributing to more efficient and maintainable code.
How to Exit an if
Statement in Python Conclusion
Exiting an if
statement can be achieved using various methods, such as using the break
statement (if the if
statement is nested within a loop), the return
statement, the exit()
function (to exit the program), structuring the if
statement with a flag variable, or the try/except
method when dealing with exceptions.
Each approach serves a different purpose, so choose the one that best fits your specific use case. Understanding these methods will help you write more efficient and organized code.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn