How to Read Environment Variables From Env File in Python
- Read Environment Variables From a Local Environment File
- Read Environment Variables by Running a Bash Script
- Conclusion
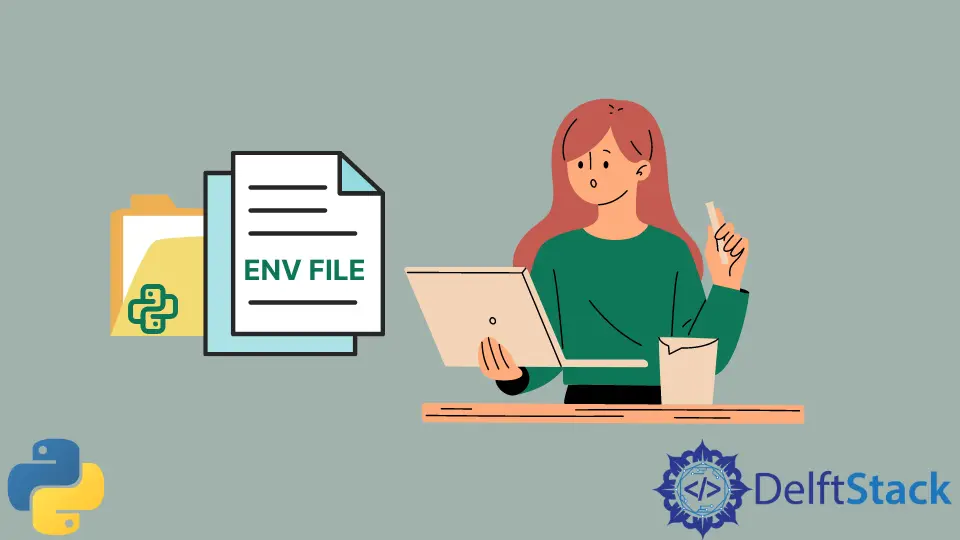
An environment file stores all the dependencies a program needs so that it does not need to interact with packages from multiple paths.
This article will focus on how to read .env
files in Python and import environment variables in Python from a local environment file.
Read Environment Variables From a Local Environment File
Most modern programs use dockers to run their programs inside environments. A docker is like an alternative virtual machine with efficient memory management.
But during testing, a program must be removed from a docker container and tested locally using a local environment file.
This article will explain how to set up a local environment file and import and read .env
files in Python.
Create an Environment File for Python Scripts
An environment file is created by providing key-value pairs of the program inside a text file and saving it with a .env
extension.
Create a .env
file by following the steps:
-
Open Notepad and write the environment variables in it. For example,
MY_ENV_VAR="This is my env var content."
-
Save this file with a name followed by the
.env
extension, for example,config.env
. This will create a.env
file. -
We now need to create a Python script in the same directory where the
.env
file is located to readenv
files in Python.
Create a Python Script That Reads the Environment Variables
This program uses the python-dotenv
library to read .env
files in Python from a local environment file. To install it, open Command Prompt or PowerShell and write the command:
pip install python-dotenv
Once python-dotenv
is installed, we can move ahead to load scripts and read env
files in Python. The program uses two library packages: python-dotenv
and os
.
The environment variables are loaded into the code using the library function load_dotenv()
. The function load_dotenv()
is used to extract environment variables from the .env
file.
Another library function is dotenv_values
, which is similar to the previous one, except it does not interact with environment variables but instead returns a dict
from the .env
file with the parsed values.
Below is the Python program:
import os
from dotenv import load_dotenv
load_dotenv()
MY_ENV_VAR = os.getenv("MY_ENV_VAR")
The last line of code imports the values of the variable MY_ENV_VAR
from the .env
file using the os.getenv
function and stores it inside a Python program with a variable of a similar name.
Printing the variable MY_ENV_VAR
will display the contents from the .env
file:
This is my env var content.
Read Environment Variables by Running a Bash Script
This is the second method that also solved the problem of when the program cannot read .env
files in Python, and the load_dotenv
library function returns none
instead of environment variables. This happens because the .env
file is not sourced, which means the Python program cannot read the .env
file and fails to load the data variables.
In systems that run shell scripts, loading the environment file and running the Python script can be wrapped together. This allows you to load the .env
file in a virtual environment and then run the Python script inside that environment.
This section will show how to load environment variables and wrap them into a shell script to read .env
files in Python.
Create a .env
File
This process is similar to the one in the previous section, but instead of using a generic word editor like Notepad, a text editor is required that offers EOL conversions.
EOL conversions convert the end of lines in a text file. To create a file like that, we need to:
-
Download and install Notepad++ or any other text editor that offers end-of-line conversions.
-
Write some environment variables, like:
TYPE=prod PORT=5000
-
Go to
Edit > EOL
conversion and select Unix(LF). -
Save the file with a
.env
extension, for example,config.env
This will create an environment file that shell scripts can read. Now, let’s write some Python code.
Create a Python Script That Can Read .env
Files in Python
This program imports and reads .env
files in Python without needing any external Python library. The program imports the library package os
and uses the library function os.environ
to extract values from variables.
The extracted values are then printed.
import os
print(os.environ.get("TYPE"))
print(os.environ.get("PORT"))
However, this script cannot independently read .env
files in Python. Running the script returns None
.
Win 10@DESKTOP-8MEIQB8 MINGW64 ~/try
$ python test.py
None
None
This happens because the Python script cannot access the environment file, even though it is inside the same directory. This is why a shell script is used, which sources the .env
file and runs the Python script simultaneously.
Create a Shell Script That Loads the .env
File Into the Python Script
This process is the same as creating an environment file, as the Bash commands are to be written in Unix EOL conversion.
Open a new file in Notepad++, and write Bash commands like:
#!/usr/bin/env bash
set -a
source config.env
set +a
python test.py
The first line of code is a shebang, a sequence of characters that usually needs to be stated in a Bash script. Here, it displays the file path from where Bash is accessed.
The set -a
allows all the variables to be exported outside into various child processes. Here, that child process is the Python script that needs to be run.
It is a shortcut for the Unix function allexport
.
The environment file is soured or, in other words, loaded into the shell script using the syntax source config.env
. The -a
allows exporting all the variables inside the .env
file that enters the environment.
The set +a
works opposite to the set -a
and turns off the exporting of variables so that no other file or code gets affected.
The last line of code starts a child process using the syntax python test.py
. It runs the Python script inside the environment, reads .env
files in Python, and returns the output of the Python script.
Once the code inside the shell script is written, its EOL needs to be converted to Unix and then saved with a .sh
extension, like run.sh
. Save the file into the same directory where the environment file and Python scripts are located.
When executed inside Bash, the tree
command shows all three files inside the same directory.
jay@DESKTOP-8MEIQB8:/mnt/c/Users/Win 10/try$ tree
.
├── config.env
├── run.sh
└── test.py
After completing the above steps, it is time to run the Bash script.
Run the Bash Script Using a Command Line Runner
A command line runner like a command prompt or PowerShell helps to run the Bash script in Windows. A better option is to install git-bash
, which allows us to run Bash directly from any directory in Windows.
This section will explain both ways.
Install Bash in Command Prompt or PowerShell
The Windows Subsystem for Linux
feature or WSL must be turned on to run Bash in Windows. It can be found inside the Turn Windows Features On or Off option.
Go to the Start menu and search for this option in the search bar. Clicking on it will open a pop-up window with a list of options and tick boxes. Scroll down until you find Windows Subsystem for Linux
and tick mark the checkbox beside it.
Once WSL is turned on in Windows, go to the Microsoft store and install a Linux distribution such as Ubuntu. Once installed, restart the computer, and Bash will be ready to be used in the command prompt and PowerShell.
To check if it is properly installed and working, go to command prompt or PowerShell and write bash
. The terminal will switch to Bash.
Install Git-Bash
An alternate option to the WSL method is the Git-Bash method. Bash can be directly downloaded and installed into the system through Git.
Open the installer after downloading it. Choose a preferred place of installation, and leave all the other settings as default.
The installer prompts multiple windows with many settings, so click Next
repeatedly until installation starts.
Run the Shell Script
Launch Bash in the directory where the Python and shell scripts are stored. If Git-bash is used, directly go to the directory, right-click and select Git Bash Here
.
If PowerShell or command prompt is used, the Bash terminal must be executed manually after going to the required directory. Once inside the directory, type the command bash
.
Inside Bash, the shell script needs to run. The shell script is named run.sh
in this example, so the Bash command to run a shell script is:
./run.sh
This will activate the environment and run the Python script inside it, and return the Output:
Win 10@DESKTOP-8MEIQB8 MINGW64 ~/try
$ ./run.sh
prod
5000
Conclusion
This article explains multiple ways to read .env
files in Python, extract environment variables from a .env
file and then use it in Python scripts. The reader should be able to easily create .env
files and shell scripts that bind Python codes and .env
files.