How to Use a DLL File From Python
- Understanding DLL Files
- Setting Up Your Environment
- Loading a DLL with ctypes
- Calling Functions in a DLL
- Error Handling When Using DLLs
- Conclusion
- FAQ
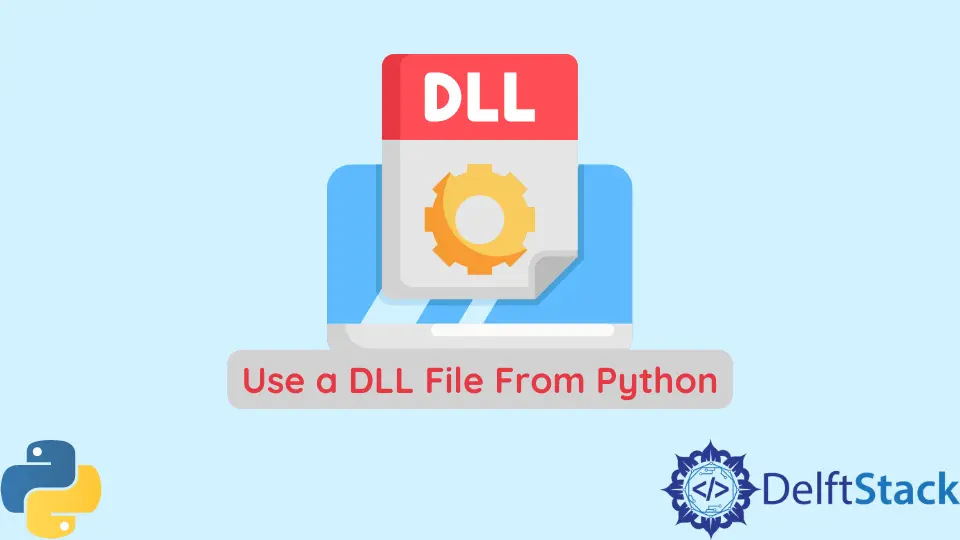
Using a DLL (Dynamic Link Library) file in Python can significantly enhance your application’s functionality by allowing you to leverage pre-existing C or C++ libraries.
This tutorial will guide you step-by-step on how to use a DLL file from Python, making it easier for you to integrate powerful functionalities into your projects. Whether you’re looking to optimize performance or access specialized libraries, this guide will provide you with the necessary tools and code examples to get started. By the end, you’ll have a solid understanding of how to interact with DLLs in your Python applications.
Understanding DLL Files
Before diving into the practical aspects, let’s clarify what a DLL file is. A DLL file is a compiled code library that contains code and data that can be used by multiple programs simultaneously. This allows for code reuse and modularization, which can be particularly beneficial for large projects. In the context of Python, utilizing a DLL can help you access low-level system functions or optimized algorithms that are not natively available in Python.
Setting Up Your Environment
To get started using a DLL file from Python, you’ll need to have a few things in place:
- Python Installed: Ensure that you have Python installed on your system. You can download it from the official Python website.
- ctypes Library: This library is included in Python’s standard library and allows you to call functions in DLLs. No additional installation is necessary.
Once you have these prerequisites, you can begin working with your DLL file.
Loading a DLL with ctypes
The ctypes
library makes it easy to load and use DLL files in Python. Here’s how you can do it:
import ctypes
my_dll = ctypes.CDLL('path_to_your_dll.dll')
In this code snippet, replace path_to_your_dll.dll
with the actual path to your DLL file. The CDLL
function loads the DLL, making its functions accessible.
Output:
DLL loaded successfully
After loading the DLL, you can call its functions directly. For example, if your DLL has a function named add
, you can call it like this:
result = my_dll.add(5, 10)
print(result)
Output:
15
In this example, the add
function takes two integers and returns their sum. The ctypes
library handles the data types for you, making it straightforward to work with functions in the DLL.
Calling Functions in a DLL
Once your DLL is loaded, you can call its functions. However, you need to define the argument and return types to ensure proper data handling. Here’s how to do that:
my_dll.add.argtypes = (ctypes.c_int, ctypes.c_int)
my_dll.add.restype = ctypes.c_int
result = my_dll.add(5, 10)
print(result)
Output:
15
By specifying argtypes
and restype
, you help ctypes
understand what types it should expect and return. This is crucial for avoiding errors and ensuring that your program runs smoothly. If the types do not match, you may encounter unexpected behavior or crashes.
Error Handling When Using DLLs
When working with DLLs, it’s essential to implement error handling to catch any issues that may arise. Here’s an example of how to add basic error handling:
try:
result = my_dll.add(5, 10)
print(result)
except Exception as e:
print(f"An error occurred: {e}")
Output:
15
In this code, we wrap the function call in a try
block. If an error occurs, the program will catch it and print a meaningful message. This practice can save you a lot of debugging time and provide clarity when things go wrong.
Conclusion
Using a DLL file from Python can dramatically expand your software’s capabilities, allowing you to utilize existing libraries and optimize performance. By following the steps outlined in this tutorial, you can easily load DLLs, call their functions, and handle errors effectively. Whether you’re working on a personal project or a professional application, understanding how to integrate DLL files will empower you to create more robust and efficient solutions. As you gain experience, you’ll find even more ways to leverage DLLs in your Python applications.
FAQ
-
What is a DLL file?
A DLL file is a compiled library that contains code and data that can be used by multiple programs simultaneously. -
How do I load a DLL in Python?
You can load a DLL in Python using thectypes
library with theCDLL
function. -
Can I call functions from a DLL in Python?
Yes, you can call functions from a DLL in Python after loading it withctypes
. -
What are argtypes and restype in ctypes?
argtypes
specifies the types of arguments a function accepts, andrestype
specifies the return type. -
How can I handle errors when using DLLs in Python?
You can handle errors using try-except blocks around your function calls to catch exceptions.