Coverage in Python
- Installation of Coverage Module
- Basic Usage of Coverage Module
- Interpreting Coverage Reports
- Advanced Coverage Features
- Conclusion
- FAQ
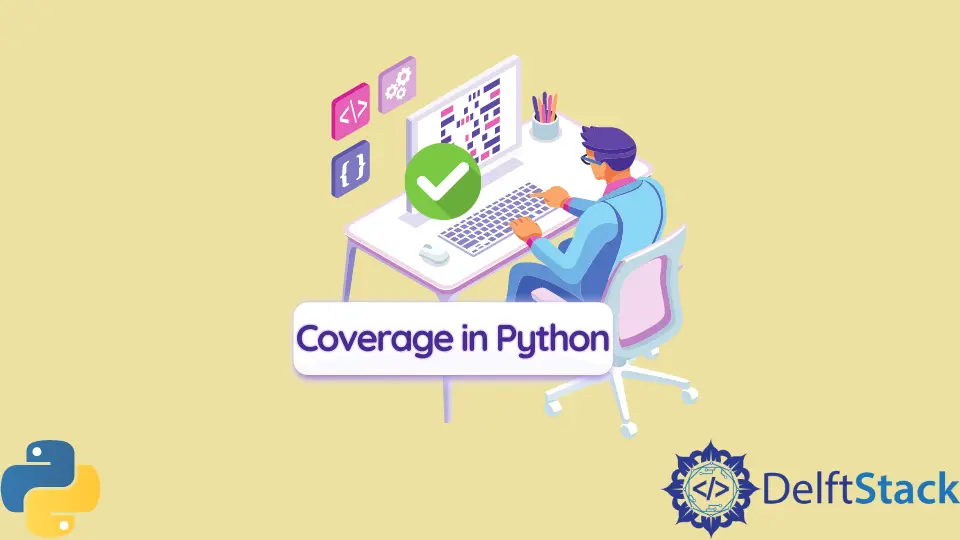
In the world of software development, ensuring the quality of your code is paramount. This is where the coverage module in Python comes into play. Coverage is a powerful tool that helps you measure how much of your code is executed during testing. By identifying untested parts of your codebase, you can improve your testing strategies and enhance the reliability of your applications.
In this tutorial, we will explore how to effectively use the coverage module for testing Python programs. We will cover installation, basic usage, and how to interpret the results to make informed decisions about your code. Whether you’re a seasoned developer or just starting, this guide will equip you with the knowledge to leverage coverage effectively.
Installation of Coverage Module
Before diving into the usage of the coverage module, you need to install it. The installation process is straightforward and can be done using pip, Python’s package installer. Open your terminal or command prompt and run the following command:
pip install coverage
After executing this command, the coverage module will be installed in your Python environment. You can verify the installation by checking the version:
coverage --version
Output:
Coverage x.x.x
Once you have confirmed that the coverage module is installed, you are ready to start measuring the coverage of your Python programs. The installation process is simple, but it lays the foundation for effective testing. With coverage installed, you can now begin to instrument your code and gather valuable insights about your testing efforts.
Basic Usage of Coverage Module
Now that you have the coverage module installed, it’s time to see how it works in practice. To use coverage, you typically run your tests through the coverage command. Here’s a simple example to illustrate how to run your tests with coverage.
Assuming you have a test file named test_sample.py
, you would execute the following command:
coverage run -m unittest test_sample
Output:
...
In this command, coverage run
tells the coverage module to run the specified Python module, which in this case is the unittest framework applied to test_sample.py
. The module collects data on which parts of your code are executed during the test run.
After running your tests, you can generate a coverage report to see the results. Use the following command to create an HTML report:
coverage html
Output:
HTML report generated in directory htmlcov
This command generates an HTML report in a directory called htmlcov
. You can open the index.html
file in your web browser to view the detailed coverage report. This report will show you which lines of code were executed during testing, providing a clear visual representation of your code coverage.
The basic usage of the coverage module is quite intuitive. By running your tests with coverage, you can quickly identify areas of your code that need additional testing. This process is essential for maintaining high-quality software and ensuring that all critical paths in your application are tested.
Interpreting Coverage Reports
After generating your coverage report, the next step is to interpret the results effectively. The coverage report provides several metrics, including line coverage, branch coverage, and more. Let’s break down how to read these metrics and what they mean for your code.
When you open the HTML report, you will see a summary of the coverage metrics at the top. Here’s what you should look for:
- Statements: This shows the total number of executable statements in your code and how many of them were executed during testing.
- Branches: This metric indicates how many branches (like
if
statements) were executed. It’s crucial for understanding conditional paths in your code. - Functions: This shows how many functions were called during your tests, giving you insight into the overall execution flow.
For example, a report might indicate:
Name Stmts Miss Cover Branch BrMiss BrCover
-------------------------------------------------------------
my_module.py 100 10 90% 50 5 90%
In this case, my_module.py
has 100 statements, with 10 missed, resulting in a coverage of 90%. This means that 90% of your code was executed during the tests, which is a good coverage percentage. However, the goal should be to minimize the missed statements and branches.
Interpreting the coverage report is crucial for identifying untested areas of your code. If you find that certain branches or statements are consistently missed, you should consider writing additional tests to cover those scenarios. This practice not only enhances the reliability of your code but also helps in preventing future bugs.
Advanced Coverage Features
The coverage module offers several advanced features that can help you gain deeper insights into your code. One of these features is the ability to exclude specific lines or files from coverage reporting. This can be particularly useful for code that is difficult to test or for third-party libraries.
To exclude lines from coverage, you can use special comments in your code. For example:
def my_function():
# pragma: no cover
return "This line will be excluded from coverage"
By adding # pragma: no cover
, you instruct the coverage tool to ignore this line when calculating coverage metrics. This can help ensure that your coverage percentage accurately reflects the parts of your code that are truly testable.
Another advanced feature is the ability to combine coverage data from multiple test runs. This is especially useful in large projects where tests are run in different environments or configurations. You can use the following command to combine coverage data:
coverage combine
Output:
Combining coverage data
This command merges the coverage data files from different runs into a single report, allowing you to get a comprehensive view of your coverage across all tests.
By leveraging these advanced features, you can fine-tune your coverage reporting to better reflect the quality of your code. This flexibility makes the coverage module a powerful tool in your testing arsenal.
Conclusion
In summary, the coverage module in Python is an invaluable resource for developers looking to ensure their code is well-tested and reliable. By measuring code coverage, you can identify untested areas, improve your testing strategies, and ultimately enhance the quality of your applications. From installation to advanced features, this guide has provided a comprehensive overview of how to use the coverage module effectively. As you continue to develop and test your Python applications, remember that thorough testing is key to delivering high-quality software.
FAQ
-
What is the coverage module in Python?
The coverage module is a tool used to measure code coverage in Python programs, indicating which parts of the code are executed during testing. -
How do I install the coverage module?
You can install the coverage module using pip by running the commandpip install coverage
in your terminal. -
How do I generate a coverage report?
To generate a coverage report, run your tests with the commandcoverage run -m unittest test_sample
, followed bycoverage html
to create an HTML report.
-
What do the coverage metrics mean?
Coverage metrics indicate the percentage of code executed during tests, including statements, branches, and functions, helping you identify untested areas. -
Can I exclude certain lines from coverage?
Yes, you can exclude lines from coverage by adding# pragma: no cover
comments in your code.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn