How to Copy Text to Clipboard in Python
-
Copy Text to Clipboard in Python Using the
pyperclip
Module -
Copy Text to Clipboard in Python Using the
pyperclip3
Module -
Copy Text to Clipboard in Python Using the
clipboard
Module -
Copy Text to Clipboard in Python Using the
xerox
Module -
Copy Text to Clipboard in Python Using the
tkinter
Module - Conclusion
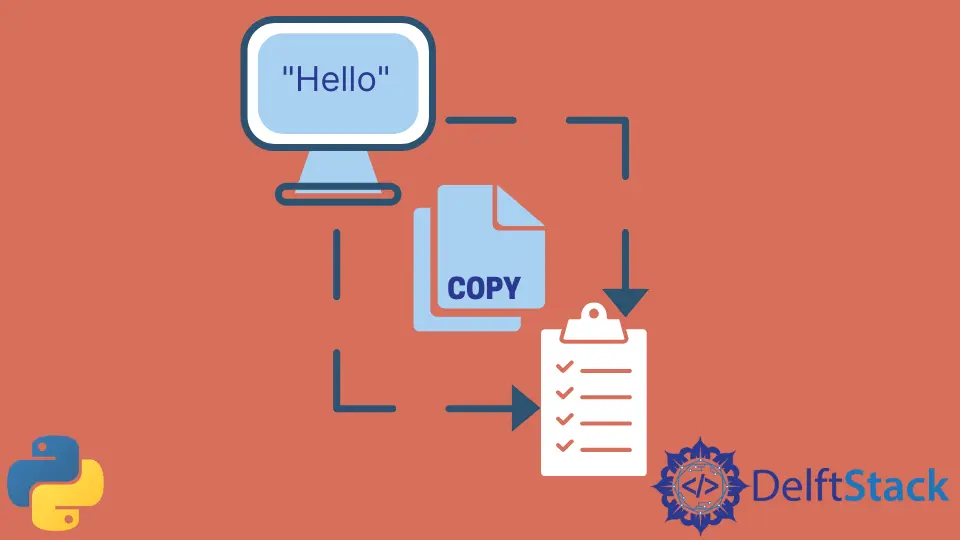
The clipboard is a temporary storage area provided by the operating system, allowing users to cut, copy, and paste content across applications. In Python, various modules provide convenient ways to interact with the clipboard and copy text.
This article discusses four distinct modules—pyperclip
, pyperclip3
, clipboard
, and xerox
—as well as the tkinter
module for graphical user interfaces.
Copy Text to Clipboard in Python Using the pyperclip
Module
The pyperclip
module is a versatile library that enables seamless cross-platform clipboard interactions in Python. It works on various operating systems, including Windows, Linux, and macOS.
The module introduces two key functions: copy()
and paste()
. The copy()
function is used to copy text to the clipboard, while paste()
retrieves the current content of the clipboard.
To use the pyperclip
module, you first need to install it using the pip
command. Here’s how you can install pyperclip
in Python.
pip install pyperclip
Once installed, you can import the module and use the copy()
function to place text on the clipboard:
import pyperclip as pc
# Text to copy to clipboard
text_to_copy = "Hello, Clipboard!"
# Copy text to the clipboard
pc.copy(text_to_copy)
# Retrieve the copied text from the clipboard
pasted_text = pc.paste()
# Print the pasted text and its data type
print("Pasted Text:", pasted_text)
print("Data Type:", type(pasted_text))
In the example, we start by importing the pyperclip
module using the alias pc
. We then define a variable text_to_copy
containing the string we want to copy to the clipboard.
The copy()
function is called with this text, placing it on the clipboard.
Next, we use the paste()
function to retrieve the text from the clipboard and store it in the variable pasted_text
. Finally, we print the pasted text and its data type using the print()
function.
Code Output:
This output confirms that the text was successfully copied to the clipboard and then retrieved, with the data type being a string.
By using the pyperclip
module, you can easily incorporate clipboard functionality into your Python applications, making it convenient to interact with and manipulate text content.
Copy Text to Clipboard in Python Using the pyperclip3
Module
In scenarios where you need to work with different data types and prefer text representation in bytes, the pyperclip3
module provides a solution. This module is an extension of the well-known pyperclip
library but specifically converts all data types into bytes.
This becomes particularly useful when dealing with non-string data. Like pyperclip
, it supports cross-platform clipboard interactions, making it versatile for different operating systems.
Before using the pyperclip3
module, it needs to be installed. You can do this by running the following command:
pip install pyperclip3
After installation, the module can be imported and utilized similarly to pyperclip
.
import pyperclip3 as pc
# Non-string data to be copied to the clipboard
data_to_copy = 987.65
# Copy data to the clipboard
pc.copy(str(data_to_copy)) # Convert to string before copying
# Retrieve the copied data from the clipboard, decode, and convert it to float
pasted_data = float(pc.paste().decode("utf-8"))
# Print the pasted data and its data type
print("Pasted Data:", pasted_data)
print("Data Type:", type(pasted_data))
In this example, we begin by importing the pyperclip3
module using the alias pc
. The variable data_to_copy
is assigned the value of a floating-point number (987.65
) as an example of non-string data.
However, since the pyperclip3
module converts non-string data to bytes during clipboard operations, we first convert the numeric value to a string using str(data_to_copy)
before being copied using pc.copy()
.
The subsequent step involves retrieving the data from the clipboard using the pc.paste()
function. As the data is returned as bytes, we need to decode it from bytes to a string using .decode('utf-8')
.
Finally, the decoded string, representing the original numeric value, is converted to a float using float()
. We then print the pasted data and its data type using the print()
function.
Code Output:
Here, the non-string data (987.65
) is successfully copied to the clipboard, and upon retrieval, it is treated as a string. This is a notable behavior of pyperclip3
, as it ensures compatibility with a wider range of data types by converting them into bytes during the clipboard interactions.
Copy Text to Clipboard in Python Using the clipboard
Module
In Python, when simplicity is key, the clipboard
module comes into play. It offers a straightforward and efficient solution for copying and pasting text to and from the clipboard.
The clipboard
module is a lightweight library designed for minimalists, providing only two functions: copy()
and paste()
. These functions serve the fundamental purpose of copying text to the clipboard and retrieving text from it.
While it may lack the versatility of some other modules, its simplicity can be advantageous for straightforward clipboard operations.
To use the clipboard
module, you first need to install it using the following command:
pip install clipboard
After installation, import the module and use the copy()
function to copy text to the clipboard:
import clipboard as c
# Text to be copied to the clipboard
text_to_copy = "Python Clipboard Magic"
# Copy text to the clipboard
c.copy(text_to_copy)
# Retrieve the copied text from the clipboard
pasted_text = c.paste()
# Print the pasted text
print("Pasted Text:", pasted_text)
In this example, we begin by importing the clipboard
module using the alias c
. The variable text_to_copy
contains the string we want to copy to the clipboard. The copy()
function is then called with this text, placing it on the clipboard.
Next, the paste()
function is used to retrieve the text from the clipboard and store it in the variable pasted_text
. Finally, we print the pasted text using the print()
function.
Code Output:
This output confirms that the text Python Clipboard Magic
was successfully copied to the clipboard and then retrieved. The clipboard
module, with its simplicity, is ideal for scenarios where basic clipboard functionality is all that’s needed.
Copy Text to Clipboard in Python Using the xerox
Module
When the goal is to keep things straightforward and platform-agnostic, the xerox
module is another solution for clipboard operations in Python.
The xerox
module is purpose-built for Python, aiming to simplify the process of copying and pasting through the clipboard. One of its notable features is its cross-platform support, making it compatible with Windows, Linux, and macOS.
To use its capabilities, you need to install it first:
pip install xerox
With xerox
, copying and pasting text becomes a seamless task, and it operates well with various data types.
The xerox
module keeps it concise with easy-to-use functions. Here’s how you can copy text to the clipboard:
import xerox
# Text to be copied to the clipboard
text_to_copy = "Python Xerox Example"
# Copy text to the clipboard
xerox.copy(text_to_copy)
# Retrieve the copied text from the clipboard
pasted_text = xerox.paste()
# Print the pasted text
print("Pasted Text:", pasted_text)
Starting with importing the xerox
module, we define the text_to_copy
variable containing the string to be copied. The copy()
function then transfers this text to the clipboard.
Subsequently, the paste()
function retrieves the text from the clipboard, storing it in the pasted_text
variable. Finally, we use print()
to display the pasted text.
Code Output:
This output confirms that the text Python Xerox Example
was successfully copied to the clipboard using the xerox
module and then retrieved. With its simplicity and cross-platform support, xerox
proves to be a handy tool for clipboard operations in Python.
Copy Text to Clipboard in Python Using the tkinter
Module
For those working on graphical user interfaces (GUIs) in Python, the tkinter
module offers a built-in solution for clipboard operations. It provides a set of tools for building graphical interfaces, making it a versatile choice for applications with GUI components.
Among its functionalities is the ability to interact with the clipboard through the tkinter.Tk()
class.
To copy text to the clipboard using tkinter
, we utilize the Tk
class to create a hidden main window. This main window’s clipboard is then manipulated using the clipboard_clear()
and clipboard_append()
methods.
By doing so, we can easily copy text to the system clipboard.
Code Example:
import tkinter as tk
# Text to be copied to the clipboard
text_to_copy = "Clipboard with Tkinter"
# Create a hidden main window
root = tk.Tk()
root.withdraw()
# Clear the clipboard and append the text
root.clipboard_clear()
root.clipboard_append(text_to_copy)
root.update()
# Print a message indicating success
print("Text successfully copied to the clipboard.")
In this example, we start by importing the tkinter
module with the alias tk
. We define the text_to_copy
variable containing the string we want to copy.
Next, we create a hidden main window using tk.Tk()
and hide it with withdraw()
.
We then clear the clipboard using clipboard_clear()
to ensure it contains no previous content. The clipboard_append()
method is used to append our desired text to the clipboard, and update()
ensures the clipboard is updated.
The script concludes by printing a message indicating that the text has been successfully copied to the clipboard.
Code Output:
This output confirms that the text Clipboard with Tkinter
has been successfully copied to the system clipboard using the tkinter
module. Integrating this approach can be beneficial when working on applications with graphical interfaces, where tkinter
is already in use for creating GUI components.
Conclusion
Copying text to the clipboard in Python is a straightforward task, thanks to various modules catering to different needs. Whether you opt for the versatile pyperclip
, the minimalist clipboard
, the platform-agnostic xerox
, or leverage tkinter
for GUI applications, these methods empower you to enhance the user experience by enabling seamless data transfer between your Python programs and external applications.
Choose the method that best suits your application’s requirements and enjoy the convenience of clipboard interactions in Python.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn