How to Clear Console in Python
- Method 1: Using os.system for Windows
- Method 2: Using os.system for Unix/Linux
- Method 3: Using ANSI Escape Sequences
- Method 4: Using a Custom Function
- Conclusion
- FAQ
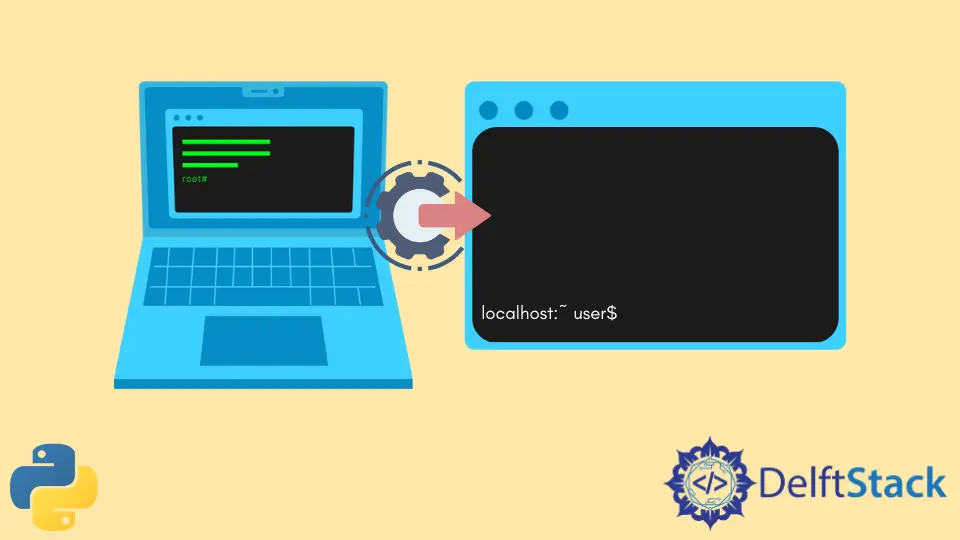
When working with Python, especially during development and debugging, you might find yourself needing to clear the console for better readability. A cluttered console can be distracting and hinder your workflow. Whether you’re running scripts in an IDE, terminal, or command prompt, knowing how to clear the console can enhance your coding experience.
In this article, we will explore various methods to clear the console in Python, providing clear examples and explanations. By the end, you’ll be equipped with the knowledge to keep your console clean and your focus sharp.
Method 1: Using os.system for Windows
One of the easiest ways to clear the console in Python on Windows is by using the os
module. The os.system()
function allows you to execute shell commands directly from your Python script. To clear the console, you can use the cls
command, which is specific to Windows.
Here’s how you can do it:
import os
os.system('cls')
Output:
<console cleared>
This code snippet imports the os
module, which provides a way of using operating system-dependent functionality. The os.system('cls')
command then executes the cls
command in the command prompt, effectively clearing the console. This method is straightforward and works seamlessly in a Windows environment.
However, it’s essential to remember that this method is specific to Windows. If you try to use it in a Unix-like environment, you will not see the desired effect. Therefore, it’s crucial to choose the right command based on your operating system.
Method 2: Using os.system for Unix/Linux
If you are working in a Unix-like environment, such as Linux or macOS, clearing the console is just as easy, but the command differs. Instead of cls
, you will use the clear
command. Here’s how you can implement this in Python:
import os
os.system('clear')
Output:
<console cleared>
Similar to the previous method, this code snippet uses the os
module to execute the clear
command. When you run this code, it will clear all previous output in the terminal, giving you a fresh slate to work with. This method is efficient and widely used among developers who prefer working in Unix-like environments.
Again, it’s crucial to note that this command is specific to Unix-based systems. Attempting to use it on Windows will not yield the desired results. Always ensure you are using the appropriate command for your operating system to avoid confusion.
Method 3: Using ANSI Escape Sequences
Another method to clear the console in Python is by using ANSI escape sequences. This approach can be particularly useful if you want a solution that works across different operating systems, including Windows, Linux, and macOS. Here’s how to do it:
import sys
def clear_console():
sys.stdout.write("\033[2J\033[H")
sys.stdout.flush()
clear_console()
Output:
<console cleared>
In this code, we define a function called clear_console()
. The sys.stdout.write()
method sends the ANSI escape sequences to the console. The sequence \033[2J
clears the screen, and \033[H
moves the cursor back to the top-left corner of the console. The sys.stdout.flush()
method ensures that the output is immediately visible in the console.
This method is advantageous because it does not rely on the operating system’s specific commands, making it more versatile. It’s a great option if you are developing cross-platform applications and want to maintain consistency in your console output.
Method 4: Using a Custom Function
If you prefer a more customizable approach, you can create a function that checks the operating system and clears the console accordingly. This method combines the previous approaches and provides a unified solution for all environments. Here’s an example:
import os
import platform
def clear_console():
if platform.system() == "Windows":
os.system('cls')
else:
os.system('clear')
clear_console()
Output:
<console cleared>
In this code, we import the platform
module to determine the operating system. The clear_console()
function checks if the system is Windows and executes the appropriate command (cls
for Windows and clear
for Unix-like systems). This method is efficient and ensures that your code remains clean and adaptable to different environments.
By using this custom function, you can easily integrate console-clearing functionality into your larger Python applications without worrying about compatibility issues.
Conclusion
Clearing the console in Python is a simple yet effective way to enhance your coding experience. Whether you’re using the os
module, ANSI escape sequences, or creating a custom function, there are various methods to achieve a clean console. By incorporating these techniques into your workflow, you can maintain focus and clarity while developing your Python applications. Remember to choose the method that best fits your operating system and coding style, and enjoy a more organized coding environment.
FAQ
-
How do I clear the console in Python on Windows?
You can use theos.system('cls')
command to clear the console in Python on Windows. -
What command should I use to clear the console in Unix/Linux?
Use theos.system('clear')
command to clear the console in Unix/Linux environments. -
Can I use ANSI escape sequences to clear the console?
Yes, you can use ANSI escape sequences like\033[2J\033[H
to clear the console across different operating systems. -
Is there a universal method to clear the console in Python?
Yes, you can create a custom function that checks the operating system and uses the appropriate command to clear the console. -
Why is it important to clear the console while coding?
Clearing the console helps improve readability and focus, making it easier to debug and develop your Python applications.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn