Template Matching Using OpenCV in Python
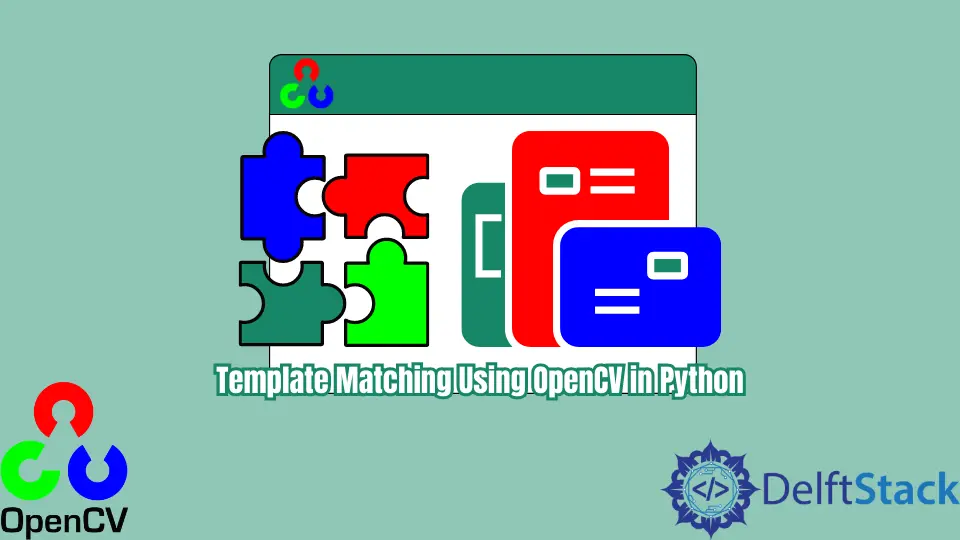
The opencv
library in Python implements many techniques and algorithms. We can perform various Computer Vision and AI tasks using this library.
One such feature of this library is template matching.
Let us discuss this technique in detail. Template matching refers to checking whether a small portion of an image is part of another image or not.
This small portion (also called template) is moved horizontally and vertically over the image to find the most likely position of the template. Template matching using opencv
has many real-life applications, especially object detection.
This tutorial will demonstrate how to perform template matching using opencv
in Python.
Use the matchTemplate()
Function to Perform Template Matching Using OpenCV in Python
The matchTemplate()
function can be used to find the position of a given template in an image. We can pass both images to this function, which will slide the template in both directions to find the best matching location.
Each location’s pixel density is calculated, and the one with the highest density (bright pixel) is the most probable match. The matchTemplate()
uses several methods and is specified using the method
parameter.
There are six in total: TM_CCOEFF
, TM_CCOEFF_NORMED
, TM_CCORR
, TM_CCORR_NORMED
, TM_SQDIFF
, and TM_SQDIFF_NORMED
.
Note that the TM_CCORR
is not considered a good choice due to its inaccurate results. Another thing to remember is that for the TM_SQDIFF
and TM_SQDIFF_NORMED
methods, we select the minimum value.
This will be clear with the examples below.
Now that we have discussed this function in detail let us observe a working example. The template and the full image are displayed below, followed by the code and result.
Image:
Template:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
temp = cv2.imread("temp.png", 0)
width, height = temp.shape[::-1]
result = cv2.matchTemplate(img, temp, cv2.TM_CCOEFF)
val_min, val_max, min_loc, max_loc = cv2.minMaxLoc(result)
t_left = max_loc
b_right = (t_left[0] + width, t_left[1] + height)
cv2.rectangle(i, t_left, b_right, 255, 2)
cv2.imshow("Output", i)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
Let us now discuss the code in detail. We start by reading the required images.
We convert this image to grayscale using the cvtColor()
function to convert images to required color spaces. Black and white images provide the best result.
We pass the template and the image to the matchTemplate()
function along with the TM_CCOEFF
method.
This returns a numpy
array. We need a set of values from this array to find the coordinates.
The minMaxLoc()
function finds an array’s minimum and maximum values and their indexes. We use the maximum value’s position to calculate the best match coordinates.
We use the rectangle()
function to draw a rectangle over the matched portion. The image is then displayed with the result.
The waitKey()
and destroyAllWindows()
functions prevent the output window from closing automatically and wait for some user input.
As discussed, we can use the other discussed methods within the matchTemplate()
function similarly. The only difference is associated with the TM_SQDIFF
and TM_SQDIFF_NORMED
methods.
We can use them by making a slight tweak in our code. See the following example.
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
temp = cv2.imread("temp.png", 0)
width, height = temp.shape[::-1]
result = cv2.matchTemplate(img, temp, cv2.TM_SQDIFF)
val_min, val_max, min_loc, max_loc = cv2.minMaxLoc(result)
t_left = min_loc
b_right = (t_left[0] + width, t_left[1] + height)
cv2.rectangle(i, t_left, b_right, 255, 2)
cv2.imshow("Output", i)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
The above example shows that we use the minimum value index to calculate the template coordinates. The remaining code remains the same.
Conclusion
This tutorial demonstrated the template matching technique using opencv
in Python. We discussed the basics of template matching and the function in the opencv
library that implements this technique.
The several methods associated with the function were also discussed. We saw a live working example of this technique using this and several other functions.
It was discussed in detail by highlighting the purpose of every line.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn