Natural Log in Python
- Natural Log in Python
- NumPy Method for Natural Log in Python
- Graphical Representation of Natural Log in Python
- Math Method for Natural Log in Python
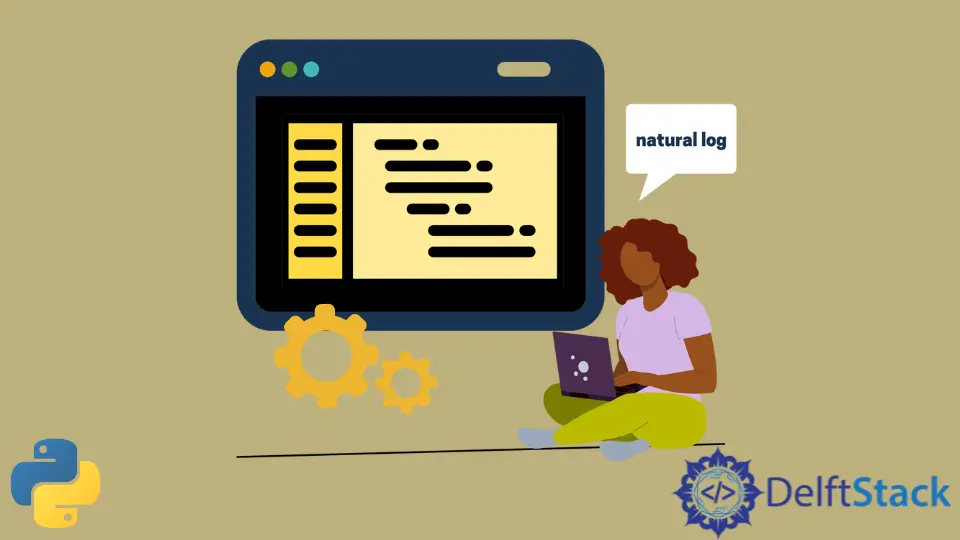
In this article, we’ll look at how to use Python to calculate the natural log of any number. You’ll learn the natural logarithm and how to compute it in Python using the NumPy library.
Natural Log in Python
It is the logarithm to the base of the irrational and transcendental number e, approximately equal to 2.718281828459. It is represented by ln()
.
There are two methods of getting a natural log in Python. One is by using NumPy, and the other is the math method.
Let’s discuss using NumPy to get a natural log in Python.
NumPy Method for Natural Log in Python
NumPy is still the most critical Python module for computer science. It is a Python library that includes multidimensional array objects and derived objects (such as masked arrays and matrices).
It has various routines for fast array operations, such as mathematical, logical, shape manipulation, sorting, selecting, I/O, discrete Fourier transforms, introductory linear algebra, basic statistical operations, random simulation, etc.
NumPy method also contributes to finding the natural log of any number or value in Python programming. The users can find the natural log x where x is any number that the users give with the help of the np.log()
function.
With the help of this function, we can find the log of a list or an array in Python.
As shown below, let’s go through an example and use NumPy to find the natural log.
# python
import numpy as np
internal_array = [1, 3, 5, 2, 8]
print("Input array : ", internal_array)
external_array = np.log(internal_array)
print("Output array : ", external_array)
print("\nnp.log(2**4) : ", np.log(2 ** 4))
print("np.log(3**9) : ", np.log(3 * 9))
Output:
From the above example program, a random array is given. We must find the natural log of this array.
We use the NumPy function of the log, which takes every element of the array and then gives it as output in the form of an output array.
Graphical Representation of Natural Log in Python
We can also find the graphical representation of the array element in this program. We first find the log of the array elements and then represent a graph of these values.
# python
import numpy as np
import matplotlib.pyplot as plt
internal_array = [1, 4.5, 4.1, 6.1, 8.1, 2.4]
external_array = np.log(internal_array)
print("out_array : ", external_array)
plt.plot(internal_array, internal_array, color="blue", marker="*")
plt.plot(external_array, internal_array, color="red", marker="o")
plt.title("numpy.log()")
plt.xlabel("external_array")
plt.ylabel("internal_array")
plt.show()
Output:
Math Method for Natural Log in Python
We can compute the natural log of an array or list in Python by utilizing the importing math method.
In this technique, we first import math into the software and then use the natural log method to find the log of values. It is a quick and straightforward method to find the log in python programming.
Let’s go through an example in which we will use the math method to get the natural log of a number in Python.
# python
import math
NUM = 23
natural_log = math.log(NUM)
print("natural log of the", NUM, "is", natural_log)
Output:
From the above example, we can quickly get the log of any number using math.log()
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn