Mutex in Python
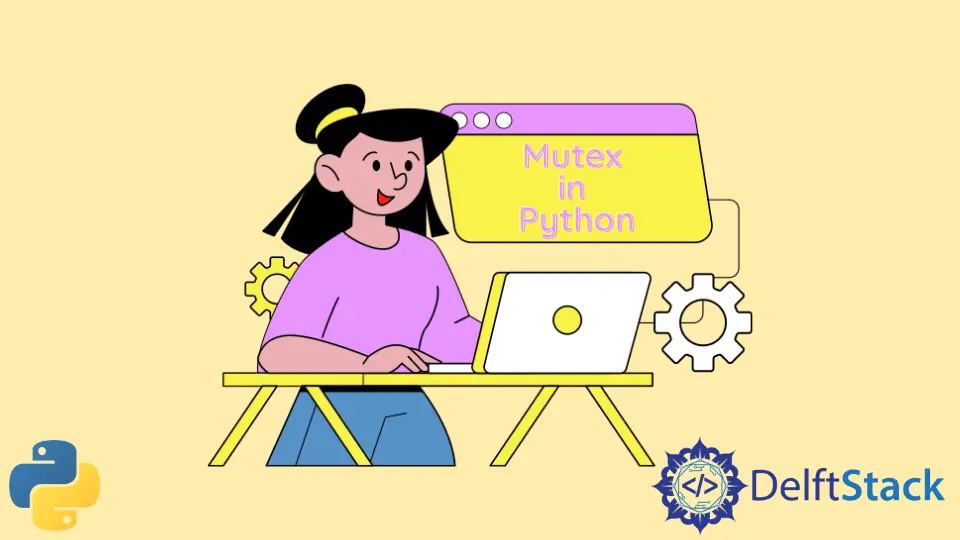
Mutex, short for mutual exclusion, is a crucial concept in concurrent programming. It helps manage access to shared resources in a multi-threaded environment, ensuring that only one thread can access a particular resource at a time.
In this tutorial, we will explore the use of mutex in Python, demonstrating how to implement it effectively. Whether you’re developing a multi-threaded application or just want to understand how to prevent race conditions, this guide will provide you with the knowledge you need. We’ll cover the basics of mutex, how to use it in Python, and provide clear examples to illustrate its functionality. Let’s dive in!
Understanding Mutex
Before we jump into the implementation, it’s essential to understand what a mutex is and why it’s necessary. In multi-threaded applications, threads often share resources. When two or more threads attempt to modify a shared resource simultaneously, it can lead to inconsistent or corrupted data. This is where a mutex comes into play. By locking a resource with a mutex, you can ensure that only one thread can access it at any given time, effectively preventing race conditions.
Mutexes are particularly important in applications that require high reliability and data integrity. In Python, the threading
module provides a simple way to implement mutexes using the Lock
class. Let’s take a look at how to use this class in practice.
Implementing Mutex in Python
To implement a mutex in Python, we will utilize the threading
module. The Lock
class is the primary tool for creating a mutex. Here’s a simple example that demonstrates how to use a mutex to protect a shared resource.
import threading
import time
# Shared resource
shared_counter = 0
# Create a mutex
mutex = threading.Lock()
def increment_counter():
global shared_counter
for _ in range(100000):
mutex.acquire()
shared_counter += 1
mutex.release()
def decrement_counter():
global shared_counter
for _ in range(100000):
mutex.acquire()
shared_counter -= 1
mutex.release()
# Create threads
thread1 = threading.Thread(target=increment_counter)
thread2 = threading.Thread(target=decrement_counter)
# Start threads
thread1.start()
thread2.start()
# Wait for threads to finish
thread1.join()
thread2.join()
print("Final counter value:", shared_counter)
Output:
Final counter value: 0
In this example, we have a shared resource, shared_counter
, which is incremented and decremented by two different threads. The Lock
object, mutex
, is used to ensure that only one thread can modify the counter at a time. When a thread wants to modify the counter, it first acquires the lock using mutex.acquire()
. Once the modification is complete, it releases the lock with mutex.release()
. This ensures that the counter remains consistent, and the final value is what we expect.
Using Context Managers for Mutex
While the previous example demonstrates the basic usage of mutexes, it is often more convenient to use context managers to handle locking and unlocking automatically. This approach reduces the chances of forgetting to release a lock and makes the code cleaner. Here’s how you can implement mutex using a context manager.
import threading
import time
# Shared resource
shared_counter = 0
# Create a mutex
mutex = threading.Lock()
def increment_counter():
global shared_counter
for _ in range(100000):
with mutex:
shared_counter += 1
def decrement_counter():
global shared_counter
for _ in range(100000):
with mutex:
shared_counter -= 1
# Create threads
thread1 = threading.Thread(target=increment_counter)
thread2 = threading.Thread(target=decrement_counter)
# Start threads
thread1.start()
thread2.start()
# Wait for threads to finish
thread1.join()
thread2.join()
print("Final counter value:", shared_counter)
Output:
Final counter value: 0
In this example, we utilize the with
statement to create a context manager for our mutex. When a thread enters the with mutex:
block, it automatically acquires the lock. Once the block is exited, the lock is released, even if an error occurs within the block. This pattern not only makes the code cleaner but also enhances reliability by ensuring that the lock is always released properly.
Conclusion
Mutexes are vital for managing access to shared resources in multi-threaded applications. By using the Lock
class from Python’s threading
module, you can easily implement mutexes to prevent race conditions and ensure data integrity. Whether you choose to use the basic locking mechanism or the more convenient context manager approach, understanding how to effectively use mutexes will significantly enhance the reliability of your applications. As you continue to develop multi-threaded programs, keep this tool in your toolkit to handle concurrency safely.
FAQ
-
What is a mutex in Python?
A mutex, or mutual exclusion, is a synchronization primitive that prevents multiple threads from accessing a shared resource simultaneously. -
How do I implement a mutex in Python?
You can implement a mutex in Python using theLock
class from thethreading
module. -
Why should I use a context manager for mutex?
Using a context manager simplifies the code and ensures that locks are released properly, even in the event of an error. -
Can I use mutex in single-threaded applications?
While mutexes are primarily used in multi-threaded applications, they can be used in single-threaded applications to maintain consistent coding practices. -
What happens if I forget to release a mutex?
If you forget to release a mutex, it can lead to deadlocks, where other threads are unable to proceed because they are waiting for the lock to be released.