Python의 뮤텍스
Ishaan Shrivastava
2022년1월22일
Python
Python Mutex
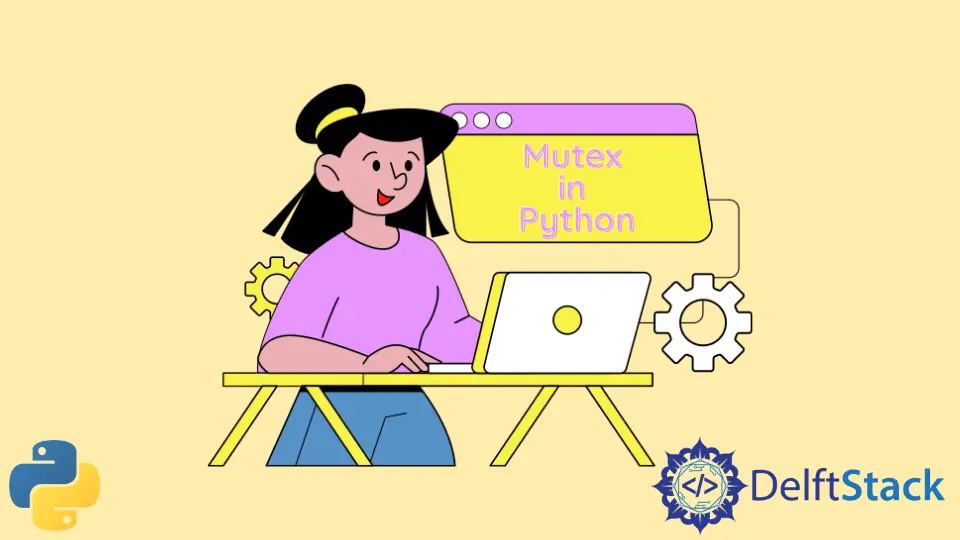
Mutex는 상호 배제를 의미합니다. 이는 주어진 특정 시간에 하나의 스레드만 특정 리소스를 사용할 수 있음을 의미합니다. 한 프로그램에 다중 스레드가 있는 경우 상호 배제는 스레드가 특정 리소스를 동시에 사용하도록 제한합니다. 다른 스레드를 잠그고 임계 영역으로의 진입을 제한합니다.
이 튜토리얼은 파이썬에서 뮤텍스의 사용을 보여줄 것입니다.
파이썬에서 뮤텍스를 구현하기 위해 threading
모듈의 lock()
함수를 사용하여 스레드를 잠글 수 있습니다. 두 번째 스레드가 첫 번째 스레드보다 먼저 끝나려고 하면 첫 번째 스레드가 끝날 때까지 기다립니다. 이를 보장하기 위해 두 번째 스레드를 잠근 다음 첫 번째 스레드가 완료될 때까지 기다리게 합니다. 그리고 첫 번째 스레드가 완료되면 두 번째 스레드의 잠금을 해제합니다.
아래 주어진 코드를 참조하십시오.
import threading
import time
import random
mutex = threading.Lock()
class thread_one(threading.Thread):
def run(self):
global mutex
print("The first thread is now sleeping")
time.sleep(random.randint(1, 5))
print("First thread is finished")
mutex.release()
class thread_two(threading.Thread):
def run(self):
global mutex
print("The second thread is now sleeping")
time.sleep(random.randint(1, 5))
mutex.acquire()
print("Second thread is finished")
mutex.acquire()
t1 = thread_one()
t2 = thread_two()
t1.start()
t2.start()
출력:
The first thread is now sleeping
The second thread is now sleeping
First thread is finished
Second thread is finished
이 코드에서 두 번째 스레드는 첫 번째 스레드가 완료될 때까지 해제되지 않습니다. 두 번째 스레드는 잠금의 첫 번째 스레드를 기다립니다. global
키워드는 두 스레드가 모두 사용하기 때문에 코드에서 사용됩니다. 스레드가 대기하는 동안에는 아직 완료되지 않았기 때문에 print
문은 acquire
문 바로 뒤에 옵니다.
따라서 스레드를 잠그는 것이 매우 중요합니다. 그렇지 않으면 두 스레드가 동일한 리소스를 동시에 공유하는 경우 응용 프로그램이 충돌할 수 있습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다