The Modulo Operator (%) in Python
- Understanding the Modulo Operator
- Using the Modulo Operator in Conditional Statements
- Applying the Modulo Operator in Loops
- Conclusion
- FAQ
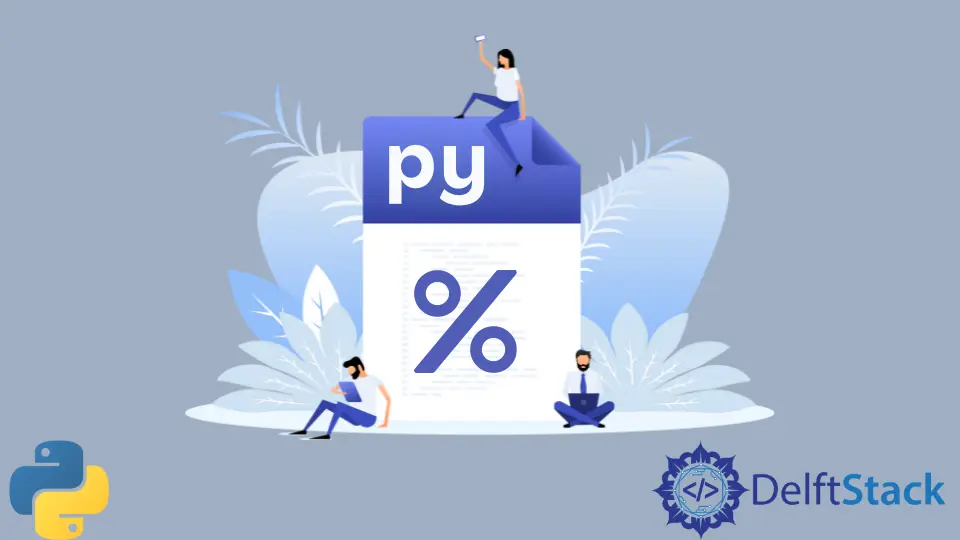
When diving into the world of programming, understanding operators is fundamental. One such operator that often sparks curiosity is the modulo operator, represented by the symbol %. This operator is not only a staple in Python but also plays a significant role in various programming languages. The modulo operator helps you determine the remainder of a division operation, which can be quite useful in numerous scenarios, such as checking for even or odd numbers, cycling through lists, or even implementing algorithms.
In this tutorial, we will explore the modulo operator in Python, providing clear examples and explanations to help you grasp its functionality and applications. Whether you’re a beginner or looking to refresh your knowledge, this guide will illuminate the nuances of the modulo operator in Python.
Understanding the Modulo Operator
The modulo operator (%) is used to find the remainder of a division between two numbers. For instance, if you divide 10 by 3, the quotient is 3 and the remainder is 1. Therefore, using the modulo operator, we can express this as:
result = 10 % 3
print(result)
Output:
1
In this example, 10 % 3
evaluates to 1. The modulo operator is particularly useful in scenarios where you need to determine whether a number is divisible by another. If the result of the modulo operation is 0, it indicates that the first number is divisible by the second.
The modulo operator can also be applied to negative numbers. For instance, -10 % 3
yields a positive result:
result = -10 % 3
print(result)
Output:
2
This behavior of the modulo operator can sometimes be confusing, but it adheres to the mathematical definition that the result should always have the same sign as the divisor.
Using the Modulo Operator in Conditional Statements
One of the most common applications of the modulo operator is in conditional statements. You can easily check if a number is even or odd using the modulo operator. If a number modulo 2 equals 0, it’s even; otherwise, it’s odd. Here’s how you can implement this:
number = 15
if number % 2 == 0:
print(f"{number} is even.")
else:
print(f"{number} is odd.")
Output:
15 is odd.
In this code snippet, we check if the variable number
is even or odd. The condition number % 2 == 0
evaluates to false since 15 is not divisible by 2, leading to the output that 15 is odd. This simple yet effective use of the modulo operator allows you to create more complex conditions in your programs.
You can also extend this concept to check for divisibility by other numbers. For example, if you want to determine if a number is divisible by 5, you can modify the condition accordingly:
number = 25
if number % 5 == 0:
print(f"{number} is divisible by 5.")
else:
print(f"{number} is not divisible by 5.")
Output:
25 is divisible by 5.
Here, the code checks for divisibility by 5, demonstrating the versatility of the modulo operator in various conditions.
Applying the Modulo Operator in Loops
Another powerful application of the modulo operator is within loops, where it can be used to cycle through elements. This is particularly useful when you want to repeat an action after a certain number of iterations. Let’s see how we can use the modulo operator to print a message every three iterations in a loop:
for i in range(10):
if i % 3 == 0:
print(f"Iteration {i}: Hello!")
Output:
Iteration 0: Hello!
Iteration 3: Hello!
Iteration 6: Hello!
Iteration 9: Hello!
In this example, the loop runs from 0 to 9. The condition i % 3 == 0
checks if the current iteration number i
is divisible by 3. If it is, it prints a message. This technique allows you to create repetitive patterns in your code easily, making the modulo operator a valuable tool in your programming toolkit.
You can also use this approach to manage tasks, such as executing a function every few iterations or creating a cycling effect in animations. The possibilities are vast, and understanding how to leverage the modulo operator can enhance your programming skills significantly.
Conclusion
The modulo operator (%) in Python is a powerful tool that enables you to perform various operations involving remainders. From checking if numbers are even or odd to cycling through elements in loops, the applications are extensive. Understanding how to use this operator effectively will not only improve your coding skills but also enhance your ability to solve complex problems. As you continue your programming journey, keep the modulo operator in mind as a versatile ally in your coding arsenal.
FAQ
-
What is the purpose of the modulo operator in Python?
The modulo operator is used to find the remainder of a division operation between two numbers. -
How do you check if a number is even or odd using the modulo operator?
You can check if a number is even by using the conditionnumber % 2 == 0
. If true, the number is even; otherwise, it is odd. -
Can the modulo operator be used with negative numbers?
Yes, the modulo operator can be applied to negative numbers, and the result will have the same sign as the divisor. -
How can I use the modulo operator in loops?
You can use the modulo operator in loops to perform actions at regular intervals, such as executing a function every few iterations. -
Is the modulo operator applicable in other programming languages?
Yes, the modulo operator is a common feature in many programming languages, including Java, C++, and JavaScript.