Method Overloading in Python
- Method Overloading in Python
- Advantages Of Method Overloading in Python
- Method Overloading in Python Using Different Data Types in the Same Method
- Method Overloading in Python Using Multiple Dispatch Decorators
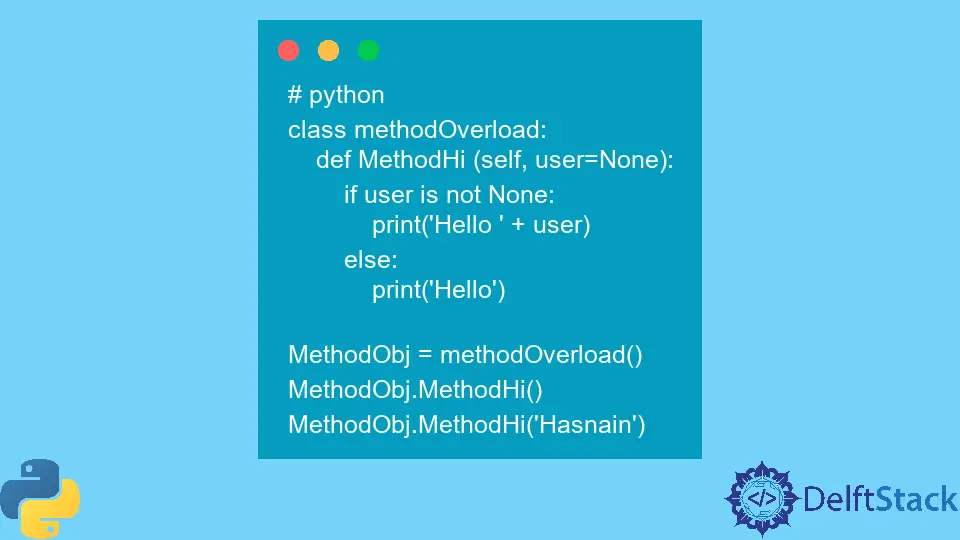
This tutorial will introduce method overloading in Python and its advantages with examples.
Method Overloading in Python
Method overloading plays a critical role in Python. Methods sometimes take in zero parameters, and sometimes they take one or more parameters.
When we call the same method in different ways, it is known as method overloading. Python does not support the overloading method by default like other languages.
Two or more methods cannot have the same names in Python because which method overloading allows us to make the same operator with different meanings. Let’s discuss method overloading in detail.
If two or more methods in the same class take distinct parameters, they might have the same name. The characteristic of method overloading allows the same operator to have several interpretations.
Overloading refers to a method or operator that may perform many functions under the same name.
Now, let’s discuss method overloading with a basic example in which we perform two different things using the same parameter.
Example:
# python
class methodOverload:
def MethodHi(self, user=None):
if user is not None:
print("Hello " + user)
else:
print("Hello")
MethodObj = methodOverload()
MethodObj.MethodHi()
MethodObj.MethodHi("Hasnain")
Output:
As you can see in this example, we created a class methodOverload
in which we defined the method MethodHi
that will greet the user with and without a name. After that class, we created an object instance using the class and called it with and without a parameter.
This type of loading of functions with different parameters is known as method overloading. Now, let’s discuss the advantages of using method overloading in our Python programs.
Advantages Of Method Overloading in Python
There are many advantages of using method overloading in Python programs. Some of them are as follows:
- Method overloading reduces the program’s complexity, function, or method. It makes it simple for the user to use it without parameters, with a single parameter, or with multiple parameters.
- Method overloading improves code quality, makes it efficient, and covers most of the use cases to make our application successful.
- Method overloading increases the reusability of the program and makes it easily accessible.
Now, let’s go through some examples of method overloading, which we will cover different aspects of method overloading. When we make the same functions and want to work them differently, we can use method overloading.
Method Overloading in Python Using Different Data Types in the Same Method
In our first example, we will make a class addition
and use different data types to perform two tasks with the same method. The program checks when the data type is an integer, then the answer will be the addition of numbers.
If the data type is a string, the answer will be the concatenation of strings. We will use a for
loop that passes through the arguments and checks them all.
If they are integers, they are added. And if they are strings, they are combined, as shown below.
Example:
# python
def addition(datatype, *args):
if datatype == "int":
result = 0
for x in args:
result = result + x
print(result)
if datatype == "str":
result = []
for x in args:
result.append(x)
print(result[0], result[1])
addition("int", 7, 11)
addition("str", "Hello", "python")
Output:
In the above example, when we provided integers as a data type with numbers to add, we received the addition of numbers. But when we gave string as a data type and passed strings, the same method concatenated the strings and displayed the result.
Method Overloading in Python Using Multiple Dispatch Decorators
Now, let’s go through another example that will be a bit tricky. In this example, we will use the efficient way to perform method overloading.
We need to install the Multiple Dispatch Decorators using the following command.
pip install multipledispatch
After installation, we will import it into our program. It dispatches three integers and three floats and displays the results below.
Example:
# python
from multipledispatch import dispatch
@dispatch(int, int, int)
def multiply(oneInt, twoInt, threeInt):
ans = oneInt * twoInt * threeInt
print(ans)
@dispatch(float, float, float)
def multiply(oneInt, twoInt, threeInt):
ans = oneInt * twoInt * threeInt
print(ans)
multiply(3, 7, 8)
multiply(3.6, 5.9, 9.9)
Output:
As you can see in the above example, we can use multiple dispatch decorators to send different data types and get results from them using the same method for all data types that we want to use.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn