How to Fix Inverse of Matrix in Python
-
Use the
numpy.linalg.inv()
Function to Find the Inverse of a Matrix in Python -
Use the
numpy.matrix
Class to Find the Inverse of a Matrix in Python -
Use the
scipy.linalg.inv()
Function to Find the Inverse of a Matrix in Python - Create a User-Defined Function to Find the Inverse of a Matrix in Python
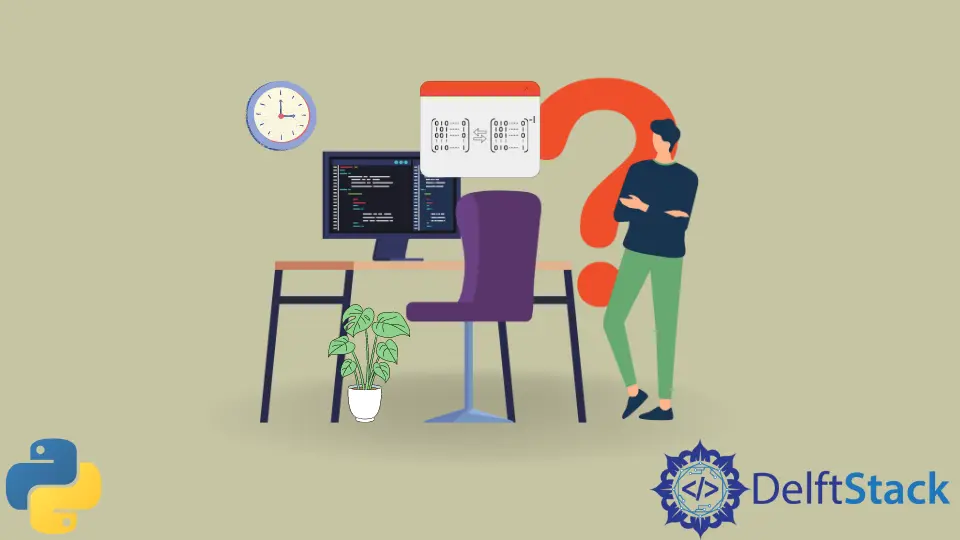
A matrix is a two-dimensional array with every element of the same size. We can represent matrices using numpy
arrays or nested lists.
For a non-singular matrix whose determinant is not zero, there is a unique matrix that yields an identity matrix when multiplied with the original. This unique matrix is called the inverse of the original matrix.
This tutorial will demonstrate how to inverse a matrix in Python using several methods.
Use the numpy.linalg.inv()
Function to Find the Inverse of a Matrix in Python
The numpy
module has different functionalities to create and manipulate arrays in Python. The numpy.linalg
submodule implements different linear algebra algorithms and functions.
We can use the numpy.linalg.inv()
function from this module to compute the inverse of a given matrix. This function raises an error if the inverse of a matrix is not possible, which can be because the matrix is singular.
Therefore, using this function in a try
and except
block is recommended. If the matrix is singular, an error will be raised, and the code in the except
block will be executed.
Code Snippet:
import numpy as np
try:
m = np.array([[4, 3], [8, 5]])
print(np.linalg.inv(m))
except:
print("Singular Matrix, Inverse not possible.")
Output:
[[-1.25 0.75]
[ 2. -1. ]]
Use the numpy.matrix
Class to Find the Inverse of a Matrix in Python
For a long time, the numpy.matrix
class was used to represent matrices in Python. This is the same as using a normal two-dimensional array for matrix representation.
A numpy.matrix
object has the attribute numpy.matrix.I
computed the inverse of the given matrix. It also raises an error if a singular matrix is used.
Code Snippet:
import numpy as np
try:
m = np.matrix([[4, 3], [8, 5]])
print(m.I)
except:
print("Singular Matrix, Inverse not possible.")
Output:
[[-1.25 0.75]
[ 2. -1. ]]
Although both the methods work the same internally, using the numpy.matrix
class is discouraged. This is because it has been deprecated and ambiguous while working with numpy
arrays.
Use the scipy.linalg.inv()
Function to Find the Inverse of a Matrix in Python
We can use the scipy
module to perform different scientific calculations using its functionalities. It works well with numpy
arrays as well.
The scipy.linalg.inv()
can also return the inverse of a given square matrix in Python. It works the same way as the numpy.linalg.inv()
function.
Code Snippet:
import numpy as np
from scipy import linalg
try:
m = np.matrix([[4, 3], [8, 5]])
print(linalg.inv(m))
except:
print("Singular Matrix, Inverse not possible.")
Output:
[[-1.25 0.75]
[ 2. -1. ]]
Create a User-Defined Function to Find the Inverse of a Matrix in Python
We can implement the mathematical logic for calculating an inverse matrix in Python. For this, we will use a series of user-defined functions.
We will create different functions to return the determinants, transpose, and matrix determinants. These functions will be used in a function that will return the final inverse.
This method works when we represent a matrix as a list of lists in Python.
Code Snippet:
def return_transpose(mat):
return map(list, zip(*mat))
def return_matrix_minor(mat, i, j):
return [row[:j] + row[j + 1 :] for row in (mat[:i] + mat[i + 1 :])]
def return_determinant(mat):
if len(mat) == 2:
return mat[0][0] * mat[1][1] - mat[0][1] * mat[1][0]
determinant = 0
for c in range(len(m)):
determinant += (
((-1) ** c) * m[0][c] * return_determinant(return_matrix_minor(m, 0, c))
)
return determinant
def inverse_matrix(m):
determinant = return_determinant(m)
if len(m) == 2:
return [
[m[1][1] / determinant, -1 * m[0][1] / determinant],
[-1 * m[1][0] / determinant, m[0][0] / determinant],
]
cfs = []
for r in range(len(m)):
cfRow = []
for c in range(len(m)):
minor = return_matrix_minor(m, r, c)
cfRow.append(((-1) ** (r + c)) * return_determinant(minor))
cfs.append(cfRow)
cfs = return_transpose(cfs)
for r in range(len(cfs)):
for c in range(len(cfs)):
cfs[r][c] = cfs[r][c] / determinant
return cfs
m = [[4, 3], [8, 5]]
print(inverse_matrix(m))
Output:
[[-1.25, 0.75], [2.0, -1.0]]
The above example returns a nested list that represents the given matrix’s inverse.
To wrap up, we discussed several methods to find the inverse of a matrix in Python. The numpy
and scipy
modules have the linalg.inv()
function that computes the inverse of a matrix.
We can also use the numpy.matrix
class to find the inverse of a matrix. Finally, we discussed a series of user-defined functions that compute the inverse by implementing the arithmetical logic.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn