How to Implement Timeout in Python Requests
- Implement Timeout for a URL in Python
- Implement Timeout on Multiple URLs Using the Requests Module in Python
-
Set
None
Inside Timeout Parameter to Implement Timeout in Python Requests - Implement Timeout for an Alternative to Python Requests
- Conclusion
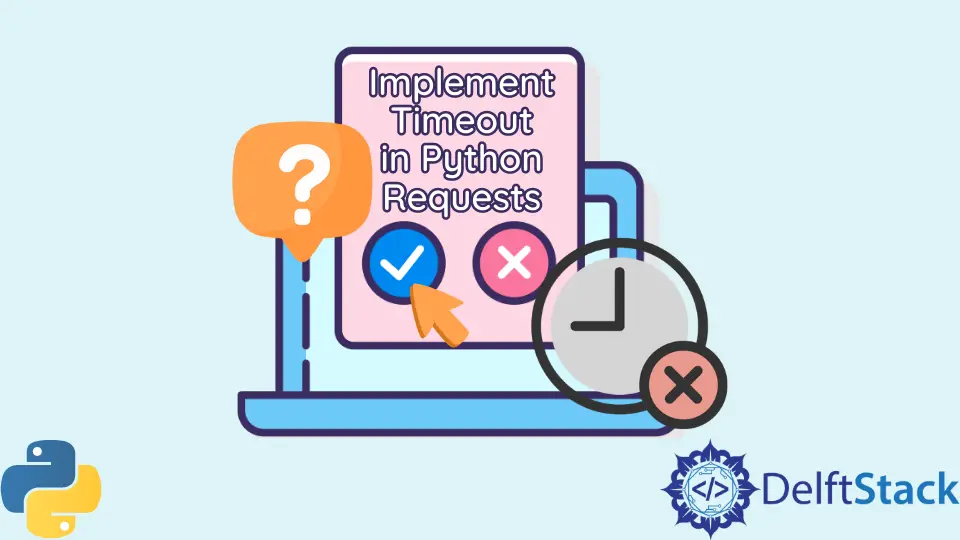
This article addresses how to implement timeouts in Python requests.
Timeouts must put a timer in request.get()
while fetching data from a connection. If a connection takes more time than the required threshold inside a timeout parameter, request.get()
gets timed out.
The examples below explain different methods of implementing timeout for Python requests using the syntax requests.get()
.
Implement Timeout for a URL in Python
This program demonstrates the method to fetch a response from an URL using GET
and implementing timeout inside it.
-
Import the Python library package
requests
. -
Define a
try
block. -
Inside the
try
block, declare a variablereq
to store requests from the required URL and set the timeout parameter. -
After the timeout parameter is set, print
req
to view the response. -
Inside the
except
block, set an exception if the program does not receive any response and print the message.
The try-except
block is an exception handling block that lets the program execute a function and sets an alternative if an exception arises.
Here, the program connects to the URL https://www.google.com
and executes the GET
command. The GET
command fetches a response from the connection, which is usually <Response [200]>
for successful connections.
The syntax timeout=(1)
tells the program to timeout connection after 1 second if no response is received.
Example:
import requests
try:
req = requests.request("GET", "https://www.google.com", timeout=(1))
print(req)
except requests.ReadTimeout:
print("READ TIME OUT")
Output:
"C:\Users\Win 10\main.py"
<Response [200]>
Process finished with exit code 0
Implement Timeout on Multiple URLs Using the Requests Module in Python
This example demonstrates the method to fetch the response from multiple URLs simultaneously. In this program, along with fetching the response, the timeout parameter will be implemented using a tuple; for example, (x,y)
is a tuple.
A timeout can be set as a tuple in reading and connecting, specified separately inside the program.
A timeout=(1,3)
indicates a connect timer of 1 second and a read timer of 3 seconds.
It must be noted that the timeout given will be applied to all the URLs. If different URLs need different timeouts, the program should contain the request.get()
function for the number of times various timeouts are there.
-
Import the library packages
requests
andurllib3
.urllib3
handles exceptions that arise from internet firewalls when the program tries to connect to domains the firewall does not recognize.The syntax below disables the warnings which arise when the program connects to an insecure website.
urllib3.disable_warnings(urllib3.exceptions.InsecureRequestWarning)
-
Inside the
try
block, initialize the variablewebsites
to store multiple domains. -
Run a
for
loopw
for the number of objects present inside the variablewebsite
. -
Initialize variable
r
to storerequest.get()
response and specify timeout(3,3)
. Verify checks for the website TLS certificate.It is given a
false
value to avoid exception throws. -
Print variable
r
. -
Inside the
except
block, store the timeout exception insidee
and print it.Example:
import requests as requests import urllib3 urllib3.disable_warnings(urllib3.exceptions.InsecureRequestWarning) try: websites = ["https://github.com", "https://www.google.com", "https://1337xto.to"] for w in websites: r = requests.get(w, verify=False, timeout=(3, 3)) print(r) except requests.exceptions.Timeout as e: print(e)
The program tries to connect to three URLs. After successfully connecting to the first two, the program prints the response.
In the third URL, the program throws a timeout exception as the URL takes more than 6 seconds to return a response.
Output:
"C:\Users\Win 10\main.py"
<Response [200]>
<Response [200]>
HTTPSConnectionPool(host='1337xto.to', port=443): Max retries exceeded with url: / (Caused by ConnectTimeoutError(<urllib3.connection.HTTPSConnection object at 0x0000022B3A202940>, 'Connection to 1337xto.to timed out. (connect timeout=3)'))
Process finished with exit code 0
Set None
Inside Timeout Parameter to Implement Timeout in Python Requests
In a particular scenario where the connection is made to a very slow website, and the response time takes more than it usually takes, timeouts are set to None
.
Example:
import requests
try:
req = requests.request("GET", "https://1337xto.to", timeout=None)
print(req)
except requests.ReadTimeout:
print("READ TIME OUT")
Implement Timeout for an Alternative to Python Requests
There are many alternatives for Python requests’ request.get()
timeout, though most are redundant or unsupported. A third-party library package eventlet
is used to execute timeout in this example.
- Import Python library packages -
requests
andeventlet
. monkey_patch
patches the standardeventlet
library with its green equivalents.- Inside
try
block, seteventlet.Timeout
for desired seconds. - Initialize variable
req
to store URL response usingrequest.get()
syntax. - Inside the
except
block, print timeout message.
Example:
import requests
import eventlet
eventlet.monkey_patch()
try:
with eventlet.Timeout(10):
req = requests.get(
"http://ipv4.download.thinkbroadband.com/1GB.zip", verify=False
)
print(req)
except:
print("timeout")
Output:
"C:\Users\Win 10\curl.py"
timeout
Process finished with exit code 0
Conclusion
Implementing timeout in request.get()
is explained with three example codes of Python requests, and another example is given to demonstrate timeout in the eventlet
.
After going through this article, the reader will be able to implement a timeout in Python requests.