How to Implement Curl Commands Using Requests Module in Python
-
Install the
requests
Module in Python -
Different Curl Commands Using the
requests
Module in Python - Conclusion
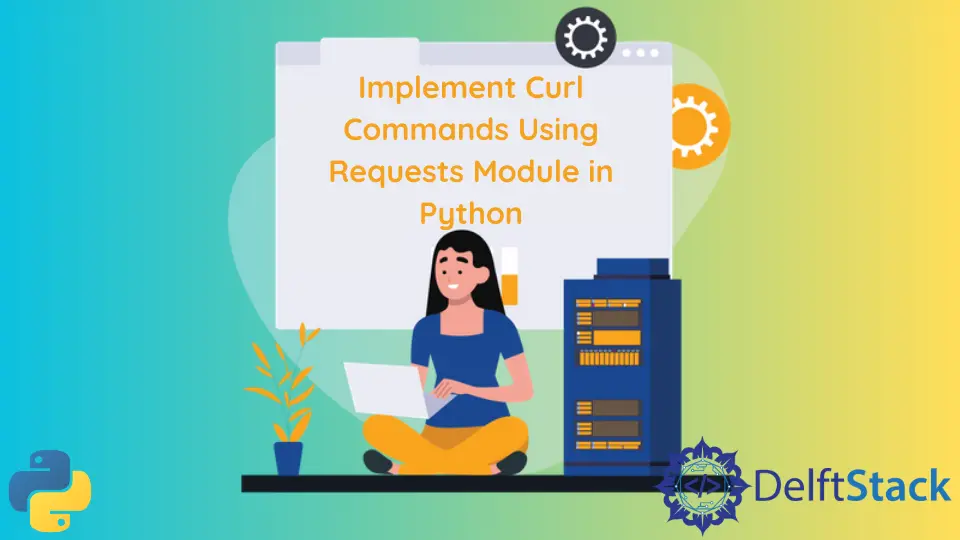
This article will discuss and implement different curl commands using the requests
module in Python.
Install the requests
Module in Python
Python provides us with the requests
module to execute curl commands. Install this in Python 3 using Pip3 by running the following command in the bash terminal.
pip3 install requests
And for previous versions of Python, install the Requests module using Pip by executing the following command.
pip install requests
The following section will implement all the curl methods in Python one by one.
This tutorial will send requests to the Dummy Rest API to execute the commands, a dummy API that responds to curl commands. The data returned by the curl commands contains a fake social media platform with different users, posts, albums, photos, etc.
Different Curl Commands Using the requests
Module in Python
Because we need to perform different operations while interacting with a server, there are specific curl commands for each task.
Implement the Get
Curl Command Using Requests Module in Python
We use the Get
curl method to request information from a server. The examples for this are any read-only operation like clicking on a web page, streaming videos, etc.
We can use the requests.get()
method which accepts the URL for the Get
method as its input argument and returns a Response
object of requests.Response
type.
Example:
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The response is:", output)
print("Data type of response is:", type(output))
Output:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
Each Response
object returned has specific attributes like response code, headers, content, etc. We can access it as follows.
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
Output:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:29:06 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Transfer-Encoding': 'chunked', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '998', 'X-Ratelimit-Reset': '1645368134', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'max-age=43200', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"116-jnDuMpjju89+9j7e0BqkdFsVRjs"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'HIT', 'Age': '2817', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=gqOambHdiHjqe%2BfpyIj5A5LYTGm%2BONF4uN9kXU9%2ByYgYx%2BbU1xmnrgw8JJk4u5am5SZn%2F1xyvb%2By4zB2zNkuT%2F1thH%2Bx8xr82N50ljyZwfBS3H6n46N%2B33UdXGh2La0Oa9%2FkhbLOzr2fYmzednU6"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21d4c3cfc06eec-BOM', 'Content-Encoding': 'gzip', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "userId": 1,\n "id": 2,\n "title": "qui est esse",\n "body": "est rerum tempore vitae\\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\\nqui aperiam non debitis possimus qui neque nisi nulla"\n}'
To print the text in the response, we can use the text
attribute of the Response
object.
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The text in the response is:")
print(output.text)
Output:
The text in the response is:
{
"userId": 1,
"id": 2,
"title": "qui est esse",
"body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla"
}
We can also print the response of the get()
method in JSON format using json()
.
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The json content in the response is:")
print(output.json())
Output:
The json content in the response is:
{'userId': 1, 'id': 2, 'title': 'qui est esse', 'body': 'est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla'}
Implement the Post
Curl Command Using the requests
Module in Python
We can use the Post
command to send data to a server, consider it as an insert operation in a set. Here, we don’t need to specify where the data is stored.
Examples of the Post
method include creating a user account on social media platforms, posting a question on StackOverflow, etc.
To implement, we can use the requests.post()
method.
The post()
method takes the URL for the new Request
object as its first input argument. We can pass an object such as a dictionary, a list of tuples, or a JSON object to the data parameter as the input argument.
We can send headers using the headers parameter, a JSON object using the JSON parameter, etc. The default value for the data parameter is None
.
After execution, the post()
method sends a post request to the server and returns a Response
object of the requests.Response
data type.
Example:
import requests as rq
data_object = {"userId": 111, "id": 123, "title": "Sample Title"}
api_link = "https://jsonplaceholder.typicode.com/posts/"
output = rq.post(api_link, data_object)
print("The response is:", output)
print("Data type of response is:", type(output))
Output:
The response is: <Response [201]>
Data type of response is: <class 'requests.models.Response'>
In the example, we created a new post record in the database at the server using the Post
curl command in Python.
In reality, the post is not being made on the server as we are using a dummy REST API. However, the server simulates a process and returns a status code and related data that shows that the post()
command has been executed.
Access different attributes of the Response
object returned by the post()
method as follows.
import requests as rq
data_object = {"userId": 111, "id": 123, "title": "Sample Title"}
api_link = "https://jsonplaceholder.typicode.com/posts/"
output = rq.post(api_link, data_object)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
Output:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:46:37 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Content-Length': '61', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645634849', 'Vary': 'Origin, X-HTTP-Method-Override, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'Access-Control-Expose-Headers': 'Location', 'Location': 'http://jsonplaceholder.typicode.com/posts//101', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"3d-uGbtVxfcg580jWo4cMrgwUKWTZA"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=dpGflkwdx9n%2B3elhuPKogSNjzW5A1yBpla29W5VLk6YLUzQG2i53oQXEXbF2lxPbN0qsq%2BqC%2BXSpKxAbWqN36I5sTONqyblOz%2BQ5j8mYIGGQeuWI7KiU03Vwp8BTiGwqeOBES6DOGuU22peaa78J"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21ee68cbd92eb6-SIN', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
201
The response URL is:
https://jsonplaceholder.typicode.com/posts/
The response content is:
b'{\n "userId": "111",\n "id": 101,\n "title": "Sample Title"\n}'
Implement the Put
Curl Command Using the Requests Module in Python
We also use the Put
method to send data to a server, but we specify where the data goes. Examples include updating profiles on social media platforms, posting answers to StackOverflow questions, etc.
To implement the Put
curl command, we can use the put()
method, similar to the post()
method.
After execution, it also returns a Response
object of requests.Response
data type.
import requests as rq
data_object = {"userId": 1, "id": 2, "title": "updated title", "body": "updated body"}
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link, data_object)
print("The response is:", output)
print("Data type of response is:", type(output))
Output:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
We updated a post using the put()
method. Again, the post is not updated on the server as we used a dummy REST API.
As shown below, we can print the different attributes of the Response
object returned by the put()
method.
import requests as rq
data_object = {"userId": 1, "id": 2, "title": "updated title", "body": "updated body"}
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link, data_object)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
Output:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:49:24 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Transfer-Encoding': 'chunked', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645634969', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"54-RFApzrEtwBFQ4y2DO/oLSTZrNnY"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=ad%2B%2FEAFK338xNieWtoBeSIPLc7VH015dF65QUBuE7AknX%2BKVHgzcoY7wCDpuExtug1w3zLMXq8mO%2FDVjAKEFyXe6RsgvvwEvtg8gP5elj7sDxXGDT6nXEqG7l6Q9XQ%2BY5J0SqzQIouNZ2gyo0J91"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21f27ed9ea563a-SIN', 'Content-Encoding': 'gzip', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "userId": "1",\n "id": 2,\n "title": "updated title",\n "body": "updated body"\n}'
Implement the Delete
Curl Command Using the requests
Module in Python
As the name suggests, we use the Delete
method to delete specified data from the server. Examples for the Delete
method include removing personal information from social media accounts, deleting an answer to a StackOverflow question, etc.
To implement the Delete
curl command, we use the requests.delete()
method.
The delete()
method takes the URL at which we have to perform the Delete
curl operation and other related data as input arguments. It also returns a Response
object of requests.Response
data type.
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link)
print("The response is:", output)
print("Data type of response is:", type(output))
Output:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
Print the different attributes of the Response
object returned using the code below.
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
Output:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:52:58 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Content-Length': '13', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645635209', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"d-GUEcOn+YmHSF2tizNHXIHPjfR5k"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=WCIIO1n%2FtlmR6PYwsKbNmScEPP8ypXyY0du2MWE9cYjkX3Yjl1CD4m4XgJ%2FAdDYrPQxfyc%2B4b%2F1dn%2FbimsZi9sMn0s%2FocQdFGCulOadr%2FjMmM%2FN10STq3z7v5LklANYT%2BOwW6nZg5gYNFj8BY1Z3"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21f7b75ec884e9-BOM', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "id": 2\n}'
Conclusion
This article has discussed curl commands and implemented them in Python using the requests
module. The methods provided in the Requests module are comparatively slower than the methods provided in the pycurl
module.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub