在 Python 中使用 requests 模块实现 Curl 命令
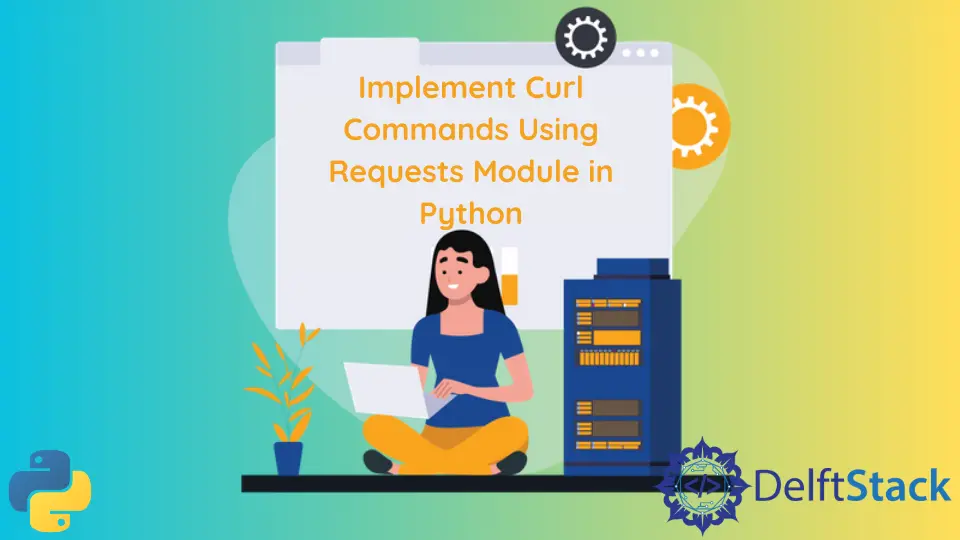
本文将讨论并使用 Python 中的 requests
模块实现不同的 curl 命令。
在 Python 中安装 requests
模块
Python 为我们提供了 requests
模块来执行 curl 命令。通过在 bash 终端中运行以下命令,使用 Pip3 在 Python 3 中安装它。
pip3 install requests
对于以前版本的 Python,请通过执行以下命令使用 Pip 安装 Requests 模块。
pip install requests
下一节将一一实现 Python 中的所有 curl 方法。
本教程将向 Dummy Rest API 发送请求以执行命令,这是一个响应 curl 命令的虚拟 API。curl 命令返回的数据包含一个虚假的社交媒体平台,其中包含不同的用户、帖子、相册、照片等。
在 Python 中使用 requests
模块的不同 Curl 命令
因为我们需要在与服务器交互时执行不同的操作,所以每个任务都有特定的 curl 命令。
在 Python 中使用 Requests 模块实现 Get
Curl 命令
我们使用 Get
curl 方法从服务器请求信息。这方面的示例是任何只读操作,例如单击网页、流式传输视频等。
我们可以使用 requests.get()
方法,它接受 Get
方法的 URL 作为其输入参数,并返回 requests.Response
类型的 Response
对象。
例子:
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The response is:", output)
print("Data type of response is:", type(output))
输出:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
每个返回的 Response
对象都有特定的属性,如响应代码、标题、内容等。我们可以按如下方式访问它。
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
输出:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:29:06 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Transfer-Encoding': 'chunked', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '998', 'X-Ratelimit-Reset': '1645368134', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'max-age=43200', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"116-jnDuMpjju89+9j7e0BqkdFsVRjs"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'HIT', 'Age': '2817', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=gqOambHdiHjqe%2BfpyIj5A5LYTGm%2BONF4uN9kXU9%2ByYgYx%2BbU1xmnrgw8JJk4u5am5SZn%2F1xyvb%2By4zB2zNkuT%2F1thH%2Bx8xr82N50ljyZwfBS3H6n46N%2B33UdXGh2La0Oa9%2FkhbLOzr2fYmzednU6"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21d4c3cfc06eec-BOM', 'Content-Encoding': 'gzip', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "userId": 1,\n "id": 2,\n "title": "qui est esse",\n "body": "est rerum tempore vitae\\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\\nqui aperiam non debitis possimus qui neque nisi nulla"\n}'
要打印响应中的文本,我们可以使用 Response
对象的 text
属性。
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The text in the response is:")
print(output.text)
输出:
The text in the response is:
{
"userId": 1,
"id": 2,
"title": "qui est esse",
"body": "est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla"
}
我们还可以使用 json()
以 JSON 格式打印 get()
方法的响应。
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.get(api_link)
print("The json content in the response is:")
print(output.json())
输出:
The json content in the response is:
{'userId': 1, 'id': 2, 'title': 'qui est esse', 'body': 'est rerum tempore vitae\nsequi sint nihil reprehenderit dolor beatae ea dolores neque\nfugiat blanditiis voluptate porro vel nihil molestiae ut reiciendis\nqui aperiam non debitis possimus qui neque nisi nulla'}
在 Python 中使用 requests
模块实现 Post
Curl 命令
我们可以使用 Post
命令将数据发送到服务器,将其视为集合中的插入操作。在这里,我们不需要指定数据的存储位置。
Post
方法的示例包括在社交媒体平台上创建用户帐户、在 StackOverflow 上发布问题等。
为了实现,我们可以使用 requests.post()
方法。
post()
方法将新 Request
对象的 URL 作为其第一个输入参数。我们可以将诸如字典、元组列表或 JSON 对象之类的对象作为输入参数传递给 data 参数。
我们可以使用 headers 参数发送标头,使用 JSON 参数发送 JSON 对象等。data 参数的默认值为 None
。
执行后,post()
方法向服务器发送一个 post 请求,并返回一个 requests.Response
数据类型的 Response
对象。
例子:
import requests as rq
data_object = {"userId": 111, "id": 123, "title": "Sample Title"}
api_link = "https://jsonplaceholder.typicode.com/posts/"
output = rq.post(api_link, data_object)
print("The response is:", output)
print("Data type of response is:", type(output))
输出:
The response is: <Response [201]>
Data type of response is: <class 'requests.models.Response'>
在示例中,我们使用 Python 中的 Post
curl 命令在服务器的数据库中创建了一条新的帖子记录。
实际上,由于我们使用的是虚拟 REST API,因此不会在服务器上发布帖子。但是,服务器会模拟一个进程并返回一个状态码和相关数据,表明 post()
命令已被执行。
访问 post()
方法返回的 Response
对象的不同属性,如下所示。
import requests as rq
data_object = {"userId": 111, "id": 123, "title": "Sample Title"}
api_link = "https://jsonplaceholder.typicode.com/posts/"
output = rq.post(api_link, data_object)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
输出:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:46:37 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Content-Length': '61', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645634849', 'Vary': 'Origin, X-HTTP-Method-Override, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'Access-Control-Expose-Headers': 'Location', 'Location': 'http://jsonplaceholder.typicode.com/posts//101', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"3d-uGbtVxfcg580jWo4cMrgwUKWTZA"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=dpGflkwdx9n%2B3elhuPKogSNjzW5A1yBpla29W5VLk6YLUzQG2i53oQXEXbF2lxPbN0qsq%2BqC%2BXSpKxAbWqN36I5sTONqyblOz%2BQ5j8mYIGGQeuWI7KiU03Vwp8BTiGwqeOBES6DOGuU22peaa78J"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21ee68cbd92eb6-SIN', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
201
The response URL is:
https://jsonplaceholder.typicode.com/posts/
The response content is:
b'{\n "userId": "111",\n "id": 101,\n "title": "Sample Title"\n}'
在 Python 中使用 Requests 模块实现 Put
Curl 命令
我们还使用 Put
方法将数据发送到服务器,但我们指定数据的去向。示例包括更新社交媒体平台上的个人资料、发布 StackOverflow 问题的答案等。
要实现 Put
curl 命令,我们可以使用 put()
方法,类似于 post()
方法。
执行后,它还返回一个 requests.Response
数据类型的 Response
对象。
import requests as rq
data_object = {"userId": 1, "id": 2, "title": "updated title", "body": "updated body"}
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link, data_object)
print("The response is:", output)
print("Data type of response is:", type(output))
输出:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
我们使用 put()
方法更新了一个 post。同样,由于我们使用了一个虚拟 REST API,该帖子没有在服务器上更新。
如下所示,我们可以打印 put()
方法返回的 Response
对象的不同属性。
import requests as rq
data_object = {"userId": 1, "id": 2, "title": "updated title", "body": "updated body"}
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link, data_object)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
输出:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:49:24 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Transfer-Encoding': 'chunked', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645634969', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"54-RFApzrEtwBFQ4y2DO/oLSTZrNnY"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=ad%2B%2FEAFK338xNieWtoBeSIPLc7VH015dF65QUBuE7AknX%2BKVHgzcoY7wCDpuExtug1w3zLMXq8mO%2FDVjAKEFyXe6RsgvvwEvtg8gP5elj7sDxXGDT6nXEqG7l6Q9XQ%2BY5J0SqzQIouNZ2gyo0J91"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21f27ed9ea563a-SIN', 'Content-Encoding': 'gzip', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "userId": "1",\n "id": 2,\n "title": "updated title",\n "body": "updated body"\n}'
在 Python 中使用 requests
模块实现 Delete
Curl 命令
顾名思义,我们使用 Delete
方法从服务器中删除指定的数据。Delete
方法的示例包括从社交媒体帐户中删除个人信息、删除 StackOverflow 问题的答案等。
为了实现 Delete
curl 命令,我们使用 requests.delete()
方法。
delete()
方法将我们必须执行 Delete
curl 操作的 URL 和其他相关数据作为输入参数。它还返回一个 requests.Response
数据类型的 Response
对象。
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link)
print("The response is:", output)
print("Data type of response is:", type(output))
输出:
The response is: <Response [200]>
Data type of response is: <class 'requests.models.Response'>
使用下面的代码打印返回的 Response
对象的不同属性。
import requests as rq
api_link = "https://jsonplaceholder.typicode.com/posts/2"
output = rq.put(api_link)
print("The header in the response is:")
print(output.headers)
print("The response code is:")
print(output.status_code)
print("The response url is:")
print(output.url)
print("The response content is:")
print(output.content)
输出:
The header in the response is:
{'Date': 'Wed, 23 Feb 2022 16:52:58 GMT', 'Content-Type': 'application/json; charset=utf-8', 'Content-Length': '13', 'Connection': 'keep-alive', 'X-Powered-By': 'Express', 'X-Ratelimit-Limit': '1000', 'X-Ratelimit-Remaining': '999', 'X-Ratelimit-Reset': '1645635209', 'Vary': 'Origin, Accept-Encoding', 'Access-Control-Allow-Credentials': 'true', 'Cache-Control': 'no-cache', 'Pragma': 'no-cache', 'Expires': '-1', 'X-Content-Type-Options': 'nosniff', 'Etag': 'W/"d-GUEcOn+YmHSF2tizNHXIHPjfR5k"', 'Via': '1.1 vegur', 'CF-Cache-Status': 'DYNAMIC', 'Expect-CT': 'max-age=604800, report-uri="https://report-uri.cloudflare.com/cdn-cgi/beacon/expect-ct"', 'Report-To': '{"endpoints":[{"url":"https:\\/\\/a.nel.cloudflare.com\\/report\\/v3?s=WCIIO1n%2FtlmR6PYwsKbNmScEPP8ypXyY0du2MWE9cYjkX3Yjl1CD4m4XgJ%2FAdDYrPQxfyc%2B4b%2F1dn%2FbimsZi9sMn0s%2FocQdFGCulOadr%2FjMmM%2FN10STq3z7v5LklANYT%2BOwW6nZg5gYNFj8BY1Z3"}],"group":"cf-nel","max_age":604800}', 'NEL': '{"success_fraction":0,"report_to":"cf-nel","max_age":604800}', 'Server': 'cloudflare', 'CF-RAY': '6e21f7b75ec884e9-BOM', 'alt-svc': 'h3=":443"; ma=86400, h3-29=":443"; ma=86400'}
The response code is:
200
The response URL is:
https://jsonplaceholder.typicode.com/posts/2
The response content is:
b'{\n "id": 2\n}'
结论
本文讨论了 curl 命令并使用 requests
模块在 Python 中实现了它们。Requests 模块中提供的方法比 pycurl
模块中提供的方法要慢。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub