How to Get and Increase the Maximum Recursion Depth in Python
-
Use the
getrecursionlimit()
Function to Get the Maximum Recursion Depth in Python -
Use the
setrecursionlimit()
Function to Set the Maximum Recursion Depth in Python
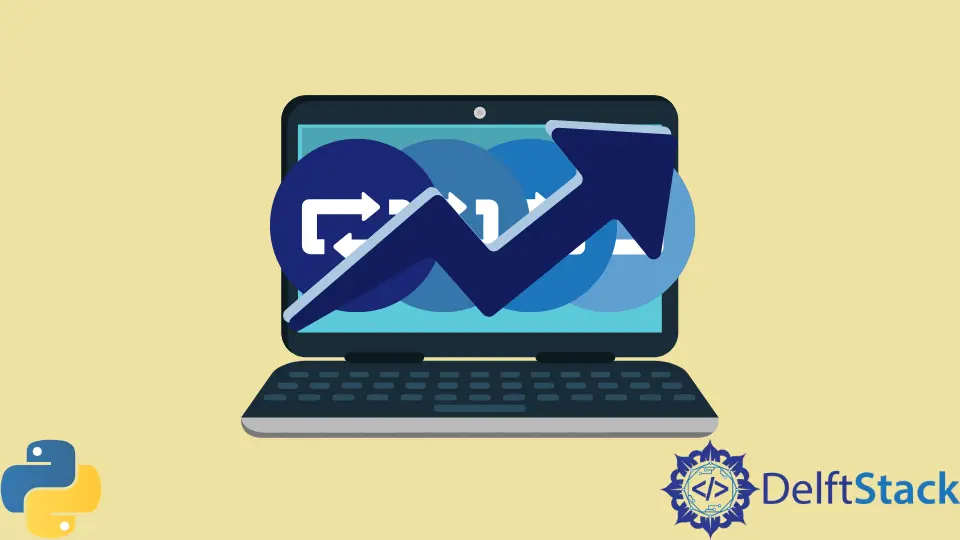
This article will introduce methods to get and increase the maximum recursion depth in Python with the getrecursionlimit()
and setrecursionlimit()
functions.
Use the getrecursionlimit()
Function to Get the Maximum Recursion Depth in Python
What is recursion? Recursion is the process of repetition.
In Python, we have some limits for recursive functions. It tells about how many times the function will repeat. We can use getrecursionlimit()
function to get the maximum recursion depth. The correct syntax is:
sys.getrecursionlimit()
This method accepts no parameters.
The program below shows how we can use this method to get the maximum recursion limit in Python.
import sys
print(sys.getrecursionlimit())
Output:
3000
Use the setrecursionlimit()
Function to Set the Maximum Recursion Depth in Python
If the recursion depth exceeds the default maximum recursion depth in Python, it will throw a RecursionError
. Let’s see the below example.
def Test(i):
if i > 0:
return Test(i - 1) + 1
else:
return 0
Test(5000)
Output:
Traceback (most recent call last):
File "C:/Test/test.py", line 7, in <module>
Test(5000)
File "C:/Test/test.py", line 3, in Test
return Test(i-1)+1
File "C:/Test/test.py", line 3, in Test
return Test(i-1)+1
File "C:/Test/test.py", line 3, in Test
return Test(i-1)+1
[Previous line repeated 1021 more times]
File "C:/Test/test.py", line 2, in Test
if i > 0:
RecursionError: maximum recursion depth exceeded in comparison
We need to increase the maximum recursion depth to solve the above issue. We use setrecursionlimit()
function to set the recursion limit. The correct syntax to use this function is as follows:
sys.setrecursionlimit(limit)
This method accepts one parameter only. The detail of its parameter is as follows:
Parameter | Description | |
---|---|---|
limit |
mandatory | It is the limit that we want to set. |
The program below shows how we can use this method to set the recursion limit in Python.
import sys
sys.setrecursionlimit(5000)
print(sys.getrecursionlimit())
Output:
5000