How to Clear Variables in IPython
-
Use
%reset
to Clear All Variables in IPython - Requires User Confirmation -
Use
%reset -f
to Clear All Variables in IPython - No User Confirmation -
Use
del
to Clear a Specific Variable in IPython -
Use
magic('reset -sf')
to Clear Variables Before Script Runs -
Use
%reset_selective
to Clear Variables Before Script Runs - Conclusion
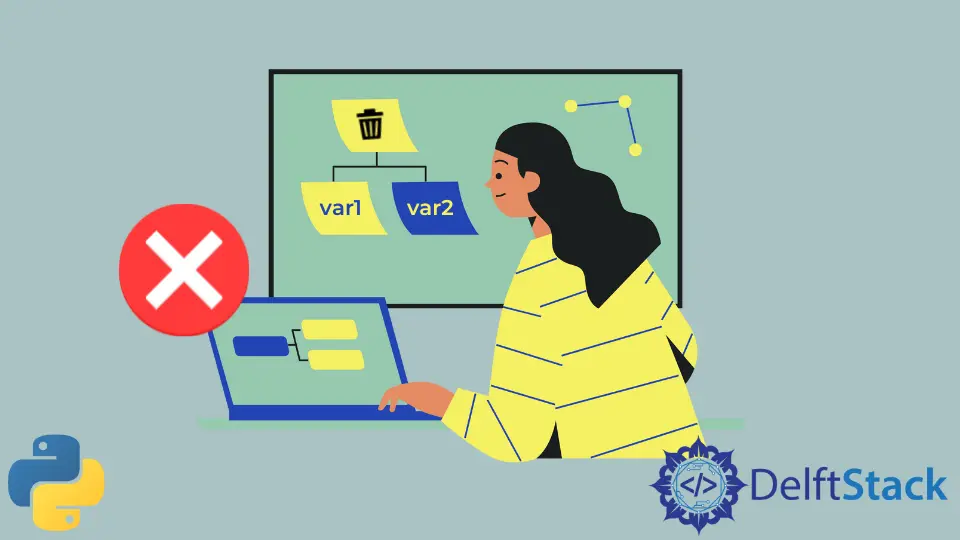
In the dynamic and interactive world of Python programming, particularly within IPython and Jupyter Notebook environments, efficiently managing the workspace is crucial for maintaining a seamless flow of work.
This article dives into the various methods available for clearing variables in these environments, ranging from complete workspace resets to selective variable deletion.
Use %reset
to Clear All Variables in IPython - Requires User Confirmation
The simplest way to clear all variables in IPython is by executing %reset
in IPython console. This command pops up a confirmation prompt before deleting the variables.
This functionality is particularly useful in scenarios where you need to clear the workspace of accumulated variables but do not wish to restart the entire Python session.
The below example illustrates this:
# Assume we are in an IPython or Jupyter Notebook environment
# Define a variable
number = 42
# Print the variable
print("Variable before reset:")
print("number:", number)
# Applying %reset
# This will prompt for confirmation in an interactive environment
%reset
# Attempt to print the variable after the reset
try:
print("\nVariable after reset:")
print("number:", number)
except NameError as e:
print("Error:", e)
In this example, we start by defining a single variable number
and print its value. We then execute the %reset
command.
In an interactive environment, this will prompt the user to confirm the action. This ensures that the user consciously decides to clear the workspace, preventing accidental data loss.
After the reset, we try to print the variable number
again, but this results in a NameError
since number
has been cleared from the namespace.
Output:
Use %reset -f
to Clear All Variables in IPython - No User Confirmation
We can also use %reset -f
to clear all variables in IPython. The difference with %reset
is that %reset -f
requires no user confirmation. The below example illustrates this:
# Assume we are in an IPython or Jupyter Notebook environment
# Define a variable
number = 42
# Print the variable
print("Variable before reset:")
print("number:", number)
# Force reset the workspace
%reset -f
# Attempt to print the variable after the reset
try:
print("\nVariable after reset:")
print("number:", number)
except NameError as e:
print("Error:", e)
In this example, we begin by defining a variable number
and displaying its value. We then apply %reset -f
to forcibly reset the workspace.
Unlike the standard %reset
command, %reset -f
does not ask for user confirmation, making the process faster and more straightforward.
After applying the reset, when we try to print number
again, we encounter a NameError
, which indicates that the variable has been successfully cleared from the namespace.
Output:
Use del
to Clear a Specific Variable in IPython
If we want to clear any specific variable, we may use del variable_name
to achieve that. The del
statement in Python is used for variable deletion.
It’s a way to remove one or more objects from the Python namespace, helping in managing memory and reducing potential confusion or errors in code. This feature is particularly useful in scenarios involving large datasets or when working with a limited memory environment.
The below example illustrates this:
# Assume we are in an IPython or Jupyter Notebook environment
# Define multiple variables
a = 5
b = 10
c = 15
d = 20
# Print the variables
print("Variables before deletion:")
print("a:", a, "b:", b, "c:", c, "d:", d)
# Delete multiple variables b and c
del b, c
# Attempt to print the variables after deletion
print("Variables after deletion:")
print("a:", a)
try:
print("b:", b)
except NameError as e:
print("Error:", e)
try:
print("c:", c)
except NameError as e:
print("Error:", e)
print("d:", d)
In this script, a
, b
, c
, and d
are initially defined and printed, confirming their presence in the namespace. We then use the del
statement to simultaneously remove b
and c
.
Attempting to print b
and c
after their deletion results in NameError
s, indicating that these variables have been successfully removed. Variables a
and d
are unaffected by the deletion and are printed normally.
Output:
Use magic('reset -sf')
to Clear Variables Before Script Runs
The manual deletion effort adds extra overhead and requires it to be done every time. A better approach will be to add a few code lines in the script we are running if we need to ensure that all the variables from memory are cleared before running the script.
The below example illustrates this:
from IPython import get_ipython
# Define a variable
a = 10
print("Variable before reset:", a)
# Perform the reset
get_ipython().magic("reset -sf")
# Attempt to print the variable after reset
try:
print("Variable after reset:", a)
except NameError as e:
print("Error:", e)
In this code, we start by defining a variable a
and printing its value, confirming its existence in the workspace. We then use get_ipython().magic('reset -sf')
to reset the workspace.
This command clears all the variables, so when we attempt to print a
again, it results in a NameError
. This behavior confirms that the variable a
, along with any other defined variables or functions, has been successfully cleared from the current workspace.
Output:
Use %reset_selective
to Clear Variables Before Script Runs
In Python’s interactive environments like IPython and Jupyter Notebooks, efficiently managing the workspace is key for productivity. While the %reset
command is widely known for clearing everything, its lesser-known cousin, %reset_selective
, offers a more refined approach: it allows for selectively clearing specific variables.
The %reset_selective
magic command is tailored for scenarios where you want to delete specific variables or functions from the namespace without affecting others. This can be immensely useful in interactive data analysis or when working with large datasets, where you might need to free up memory by clearing certain variables without disrupting your entire workspace.
The below example illustrates this:
# Assume we are in an IPython or Jupyter Notebook environment
# Define some variables
a = 10
b = 20
temp_var = 30
print("Variables before reset_selective:")
print("a:", a, "b:", b, "temp_var:", temp_var)
# Use reset_selective to delete variables that match the pattern 'temp_*'
%reset_selective -f temp_*
# Attempt to print the variables after reset_selective
print("\nVariables after reset_selective:")
print("a:", a)
print("b:", b)
try:
print("temp_var:", temp_var)
except NameError as e:
print("Error:", e)
In this example, we define three variables: a
, b
, and temp_var
. We then use the %reset_selective
command with the -f
flag to immediately delete any variable whose name starts with temp_
.
After this operation, we attempt to print all the variables. We expect temp_var
to be undefined, leading to a NameError
, while a
and b
should remain unaffected.
Output:
Conclusion
The exploration of various methods to clear variables in IPython and Jupyter Notebook environments is an essential aspect of efficient Python programming. From the broad-stroke approach of %reset
and %reset -f
, which clear the entire workspace, to the precision of del
and %reset_selective
, these tools provide programmers with the flexibility to manage their coding environment effectively.
The additional method of using magic('reset -sf')
for script-level clearing further enhances this flexibility. These techniques are indispensable in scenarios that demand frequent resetting of the workspace, such as iterative testing or data analysis.
They not only help in avoiding clutter and confusion but also play a vital role in memory management, ensuring a smooth and efficient workflow. By mastering these commands, Python programmers can significantly enhance their productivity and maintain a clear, organized workspace conducive to successful coding endeavors.