GREP in Python
-
Using the
re
Module for Pattern Matching - Searching for Strings in a List
- Using Command Line Integration with Git
- Conclusion
- FAQ
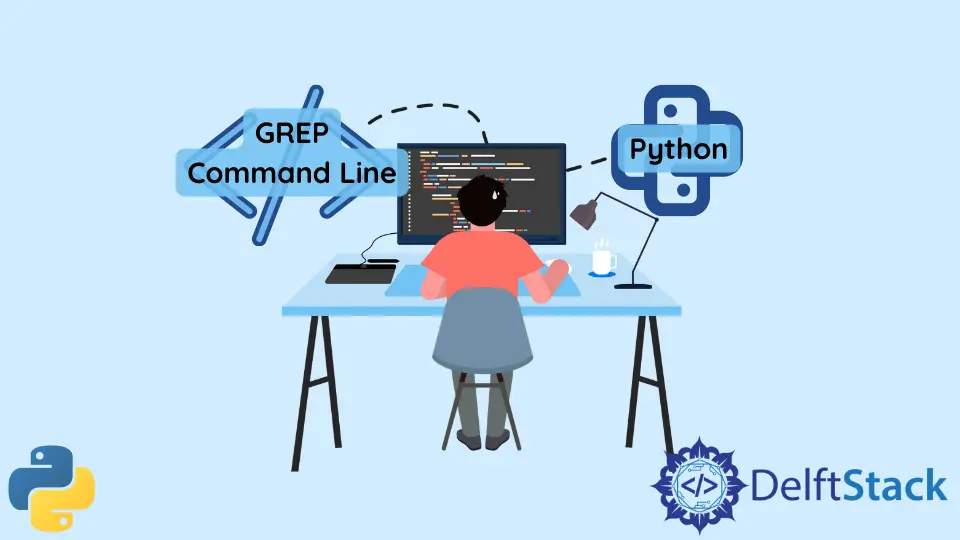
When it comes to searching text in files, the command-line tool grep is a favorite among developers. Its efficiency and simplicity make it a go-to for quickly locating patterns in files. But what if you’re working within a Python environment and want to harness similar functionality?
In this tutorial, we’ll explore how to emulate grep using Python, allowing you to search through files and strings with ease. Whether you’re analyzing logs, processing data, or just need to find specific text in files, this guide will equip you with the skills to utilize Python for your grep-like needs.
Using the re
Module for Pattern Matching
Python’s built-in re
module is a powerful tool for working with regular expressions. It allows you to search for patterns in strings and can be easily adapted to search through files. This is akin to how grep operates, making it an excellent starting point for emulating grep functionality.
To demonstrate this, let’s create a simple function that reads a file and searches for a specific pattern using regular expressions.
import re
def grep_in_file(file_path, pattern):
with open(file_path, 'r') as file:
for line_number, line in enumerate(file, start=1):
if re.search(pattern, line):
print(f'Line {line_number}: {line.strip()}')
# Example usage
grep_in_file('sample.txt', r'error')
Output:
Line 3: This line contains an error message.
Line 7: Another error occurred on this line.
In the code above, we define a function called grep_in_file
that takes a file path and a search pattern as arguments. It opens the specified file and iterates through each line. The enumerate
function helps keep track of the line numbers. If a line matches the specified pattern (in this case, ’error’), it prints the line number along with the line itself. This method is highly flexible and can be modified to accommodate various patterns, just like grep.
Searching for Strings in a List
Another common scenario is searching for strings within a list. This is particularly useful when you have a set of strings and want to filter them based on specific criteria. Python’s list comprehensions make this task straightforward and efficient.
Here’s how you can achieve this:
def grep_in_list(strings, pattern):
matches = [s for s in strings if re.search(pattern, s)]
return matches
# Example usage
strings = ['This is a test', 'Another test', 'Error found here', 'No errors']
results = grep_in_list(strings, r'error')
for match in results:
print(match)
Output:
Error found here
In this example, the grep_in_list
function takes a list of strings and a pattern, returning a list of matches. The list comprehension filters through the strings, applying the regular expression search. The result is a concise list of matches, which you can then display or process further. This method mirrors grep’s ability to filter lines based on patterns, making it a powerful tool for data analysis and manipulation.
Using Command Line Integration with Git
If your work involves version control with Git, you might want to perform grep-like searches directly within your Git repositories. Git has a built-in command called git grep
that allows you to search through your tracked files for a specific pattern. This is particularly useful for quickly locating code snippets or documentation within your project.
Here’s how to use it:
git grep "function_name"
Output:
src/module.py: def function_name(param):
tests/test_module.py: result = function_name(test_param)
The command git grep "function_name"
searches through all tracked files in your Git repository for occurrences of “function_name”. It displays the filename and the line where the match occurs, similar to how grep works in a command-line environment. This command is incredibly efficient and leverages Git’s indexing to perform fast searches, making it an invaluable tool for developers.
Conclusion
Emulating grep in Python is not only possible but also straightforward with the right tools and techniques. Whether you’re using the re
module for regex searching in files or strings, or leveraging Git’s built-in git grep
command for repository-wide searches, Python provides the flexibility and power needed to handle text searches effectively. By mastering these methods, you can enhance your productivity and streamline your workflow, making text searching a breeze.
FAQ
-
What is grep?
grep is a command-line tool used to search for specific patterns in text files. -
Can I use grep in Python?
Yes, you can emulate grep functionality in Python using there
module and other techniques.
-
How do I search for a pattern in a file using Python?
You can use there
module to read a file line by line and search for the desired pattern. -
What is the difference between grep and git grep?
grep searches through files in the filesystem, while git grep specifically searches through files tracked by Git. -
Can I search for multiple patterns at once?
Yes, you can modify the regular expression pattern to include multiple search terms.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn