Python 中的 GREP
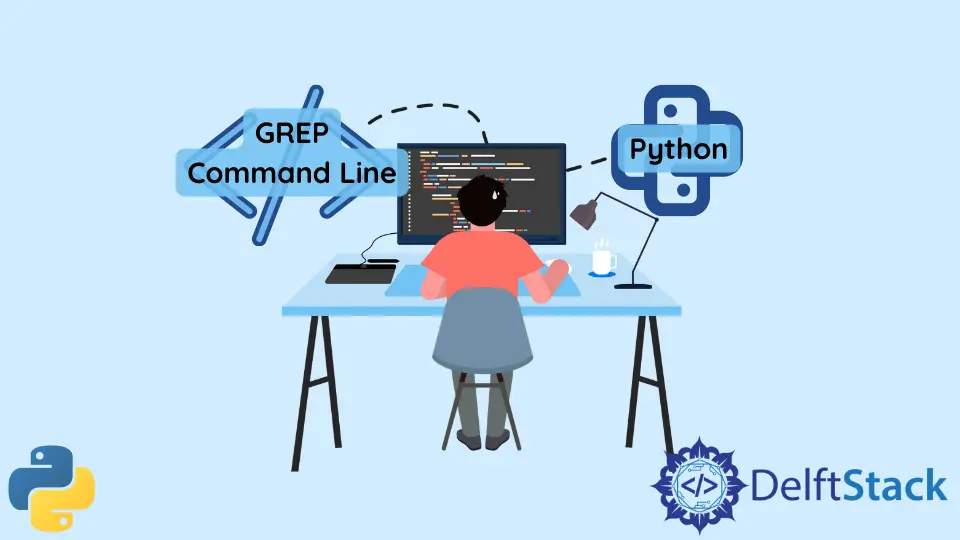
GREP 是一个有趣的命令行功能,它使我们可以使用正则表达式在纯文本文件中搜索特定行。
正则表达式在 Python 中使用率很高,可用于检查字符串是否与模式匹配。
Python 中的 re
模块允许我们处理正则表达式。在以下代码中,我们将尝试在 Python 中实现 GREP,并在文件中搜索某些特定模式。
with open("sample.txt", "r") as file:
for line in file:
if re.search(pattern, line):
print(line)
我们以读取模式打开所需的文件,并逐行遍历文件。然后,我们使用 re.search()
函数在每一行中搜索模式。如果找到了图案,则将打印该行。
还有另一种简洁的方法可以在 Python 命令行中实现。此方法将在终端中运行文件时在命令行中指定正则表达式和要搜索的文件。这使我们能够在 Python 中正确复制 GREP。
以下代码实现了这一点。
import re
import sys
with open(sys.argv[2], "r") as file:
for line in file:
if re.search(sys.argv[1], line):
print(line)
sys
模块提供了 argv()
函数,该函数返回命令行中提供的所有参数的数组。
我们可以将该文件另存为 grep.py
并从终端运行此 Python 脚本,并通过以下方式指定必要的参数。
python grep.py 'RE' 'file-name'
如果要使用多个参数,则可以使用 glob
模块。
glob
模块使我们能够找到与目录中的模式匹配的文件的路径。
可以在下面看到它在 Python 中复制 GREP 的用途。
import re
import sys
import glob
for arg in sys.argv[2:]:
for file in glob.iglob(arg):
for line in open(file, "r"):
if re.search(sys.argv[1], line):
print(
line,
)
iglob()
函数创建一个对象,该对象返回目录中的文件,该文件将传递给该函数。
下面显示了另一种简洁的实现 GREP 的方法。
import re
import sys
map(sys.stdout.write, (l for l in sys.stdin if re.search(sys.argv[1], l)))
这种方式更精确,内存效率更高,我们可以直接在终端中运行这些行。
python -c "import re,sys;map(sys.stdout.write,(l for l in sys.stdin if re.search(sys.argv[1],l)))" "RE"
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn