Global Variables and How to Change From a Function in Python
-
Use
Global Variables
and Change Them From a Function in Python -
Create the
Global
Variable in Python -
Change the Value of
Global
Variable From a Function in Python -
the
Global
Keyword in Python - the Multiple Functions and a Global Variable
-
a Function That Has a Variable With the Same Name as a
Global
Variable - Conclusion
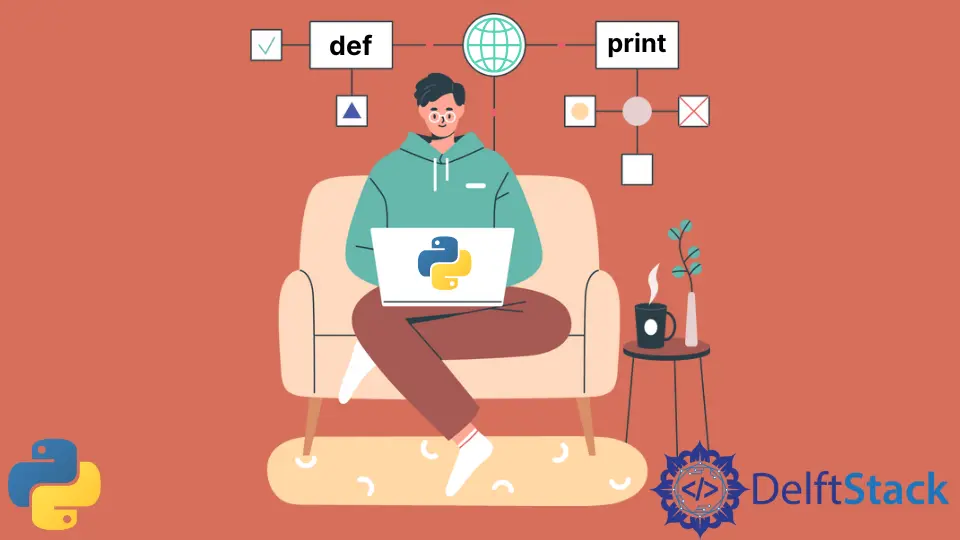
Global
variables in Python are those variables that have a global scope. In other words, their scope is not limited to any specific function or block of the source code.
Use Global Variables
and Change Them From a Function in Python
First, declare the variable x
.
def avengers():
x = 10
print("Gibberish")
avengers()
print(x)
Output:
Gibberish
NameError: name 'x' is not defined
The variable x
is inside the function avengers
. This means that the scope of this variable is limited to this function only.
That is why we get an error if we try to access this variable outside this function.
Create the Global
Variable in Python
Move the variable x
declaration outside the function.
x = 10
def avengers():
print("Gibberish")
avengers()
print(x)
Output:
Gibberish
10
The variable x
is defined outside the function, and thus, we can use it anywhere in our program.
Also, it is a global
variable. Declaring a variable in the global scope creates a global
variable in Python.
We can also access the global
variable x
from the avengers
function.
x = 10
def avengers():
print("From avengers:", x)
avengers()
print("Outside avengers:", x)
Output:
From avengers: 10
Outside avengers: 10
Change the Value of Global
Variable From a Function in Python
This code has a global
variable x
with 10
. Then, inside the function change
, we add 12
to this variable x
.
A print statement inside the function should print the updated value of x
.
x = 10
def change():
x = x + 12
print(x)
change()
Output:
UnboundLocalError: local variable 'x' referenced before assignment
In python, a function can only access and print a global variable
. We need to tell the function referring for any assignment or change to the global variable
.
If we do not specify this, the function thinks that assignments and changes are made to the local variable itself. Thus, we get this error.
Use the global
keyword to change a global variable
value from inside a Python function.
the Global
Keyword in Python
Python gives you a keyword named global
to modify a variable outside its scope. Use it when you have to change the value of a variable or make any assignments.
Let us try fixing the above code using the global
keyword.
x = 10
def change():
global x
x = x + 12
print(x)
change()
Output:
22
See how we specify x
as global using the global
keyword in the third line.
Now, let us see the value of variable x
when printing it outside the function.
x = 10
def change():
global x
x = x + 12
print(x)
change()
print(x)
Output:
22
22
Since the function has updated x
from 10
to 22
, we will get the updated value as output even when accessing the variable outside the local scope.
var = "A"
def chocolate():
print("From chocolate:", var)
def cake():
global var
var = var * 2
print("From cake:", var)
chocolate()
cake()
print("From main:", var)
Output:
From chocolate: A
From cake: AA
From main: AA
The best inference you can draw from this output is - order matters. The chocolate
function uses the initial value of var
and not the modified value.
This is because the function cake
that modifies the value of var
is called after the function chocolate
. If we call the cake
function first, the chocolate
function will also use the updated value of var
.
var = "A"
def chocolate():
print("From chocolate:", var)
def cake():
global var
var = var * 2
print("From cake:", var)
cake()
chocolate()
print("From main:", var)
Output:
From cake: AA
From chocolate: AA
From main: AA
This brings us to some rules that you must follow while using Python’s global
keyword.
- By default, a variable inside a function is local, and a variable outside a function is
global
. Don’t use this keyword for variables outside a function. - Using the
global
keyword outside a function in Python does not impact the code in any way. - The main use of the
global
keyword is to do assignments or changes in Python. Thus, we do not need it for simply accessing or printing the variable.
the Multiple Functions and a Global Variable
Here, we have a global variable s
with the value 1
. See how the function college
uses the global
keyword to modify the value of s
.
s = 1
def college():
global s
s = s + 5
print("College students: ", s)
return s
def school():
s = college()
s = s + 10
print("School students: ", s)
return s
college()
school()
print("Global students: ", s)
Output:
College students: 6
College students: 11
School students: 21
Global students: 11
First, we call the function college
. This function modifies the global variable s
and changes it to 6
.
We get the output as 6
from the first print statement. Then, we call the function school
.
We again call the function school
inside the function college
. This time, the function college
also modifies the value of variable s
.
It takes the previous value of 6
and then updates it to 11
. So, the final value of the global variable now becomes 11
.
Then, the function school
modifies it, but this will not be updated in the global variable. It uses the updated value of s
and prints the value after adding 10
.
It does not use the global
keyword. Hence, the output 21
. Now you can see why the output of the last statement is 11
.
This is nothing but the updated value of the global variable s
.
a Function That Has a Variable With the Same Name as a Global
Variable
There is a possibility that we have a function that has a variable declared inside it with the same name as a global
variable.
An inevitable question that arises here is - which variable will the function use? Local or Global? Let us see.
# the global variable
a = 5
# function
def music():
# variable inside function with same name as global variable
a = 10
print("Music:", a)
music()
print(a)
Output:
Music: 10
5
There is a global
variable a
in this code, whose value is 5
. The function music
also has a variable named a
.
The value of this variable is 10
. When we access the value of the variable a
inside the function, we get the value of the variable local to this function, which is 10
.
When we access the value of a
from outside this function, we get the output as 5
.
This implies that if the local
variable is present with the same name as the global
variable in a specific scope, it has more priority than the global variable.
Conclusion
This tutorial taught the basics of global
variables in Python. We saw how they are different from local
variables and how we create them in Python.