How to Examine Items in a Python Queue
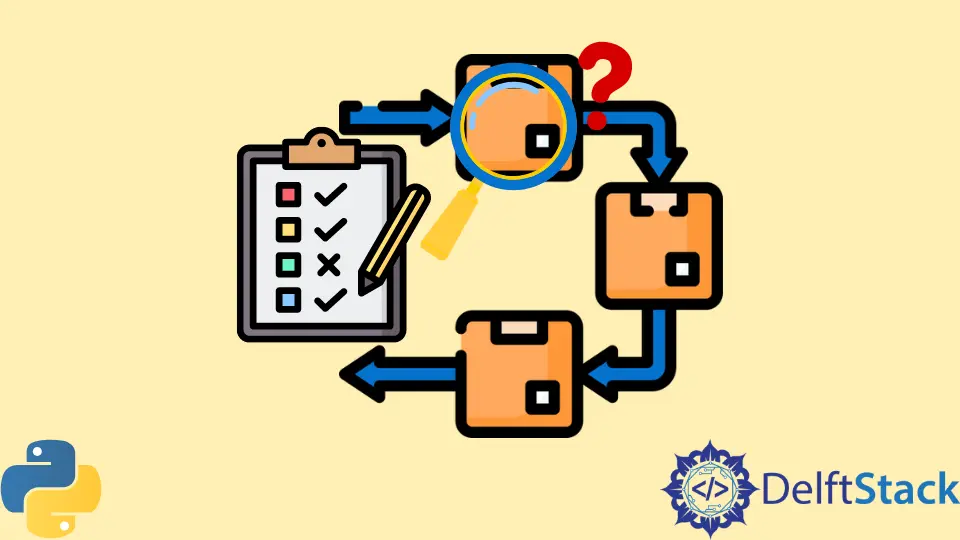
In Python, a built-in list data structure is called a module that implements multi-procedure and multi-consumer queues. In this article, we will learn Python’s built-in function queue. A queue is a data structure established on the first in and first out (FIFO)
rule when inserting and removing elements, whereas in stacks, as the name suggests, follow the Last in and First Out (LIFO)
rule.
A queue has two kinds of operations:
The procedure in which items are added to the end of the queue in an operation is called enqueue
and when the items removed from the beginning of the queue are called dequeue
operation. A queue in Python is usually fulfilled using the in-built list, Queue
from the queue
module, or deque from the collections
module.
There are two open ends in the queue
: front and rear ends. They are used for different purposes to work with queue, rear
is the end that inserts items, and front
is used as the point of removal. Though the front and rear ends are treated as pointers, when the queue
is empty, both front and rear pointers are NULL. Furthermore, we cannot dequeue an empty queue, and we need at least one item to be available in the queue when we need to dequeue.
In the code example below, we will see how we can insert items in the queue and delete those items one by one from the queue.
Queue in Python
In the code sample below, we will learn how to add and delete items from the queue item list. We will first create a list of the vegetables, and then we will add the names of the vegetables one by one. Once all items are added using the append()
function, we will delete each item one by one using the pop()
function. Furthermore, we can see that the first item inserted in the list has been deleted first, which has confirmed that the queue works as the first in and first out
(FIFO) methodology.
import queue
vegetables = []
vegetables.append("Broccoli")
vegetables.append("Potato")
vegetables.append("Carrot")
vegetables.append("Spinach")
first_item = vegetables.pop(0)
print(first_item)
Second_item = vegetables.pop(0)
print(Second_item)
print(vegetables)
Output:
Broccoli
Potato
['Carrot', 'Spinach']
Abdul is a software engineer with an architect background and a passion for full-stack web development with eight years of professional experience in analysis, design, development, implementation, performance tuning, and implementation of business applications.
LinkedIn