How to Decode Base-64 Data in Python
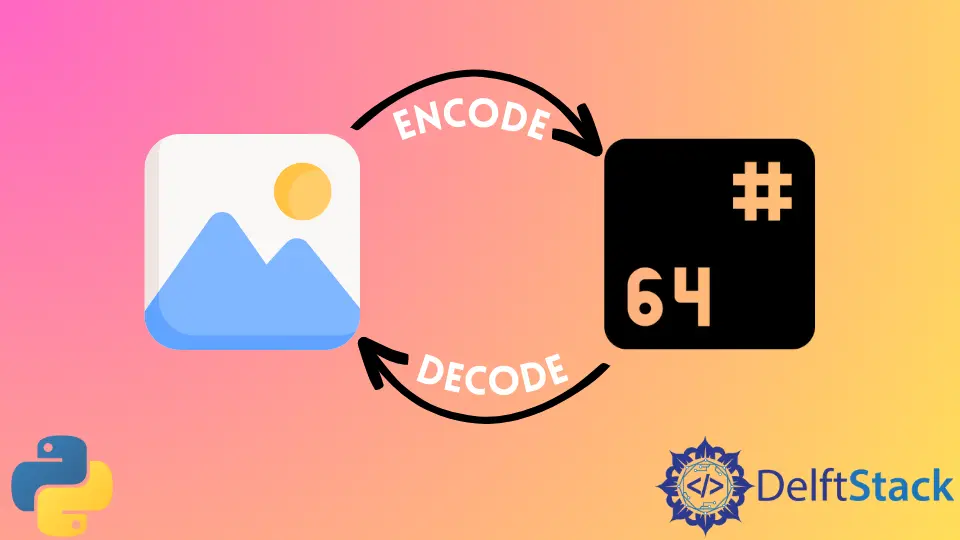
This article aims at explaining how to decode Base64. We will look at the codes on decoding Base64 data, then venture towards Base64 image decoding.
Demonstrate Decoding Base64 Data in Python
Base64 decoding is a five-step process, which is as follows.
-
Take the base64 formatted string and convert it to its corresponding decimal form, as per the base64 decoding conversion chart.
-
Convert those decimal numbers to 6-bit binary.
-
Once converted, those 6-bit binary needs to be segregated into an 8-bit binary representation format.
-
These 8-bit binary numbers then need to get converted into their decimal form again.
-
Lastly, those decimal digits need to be converted to their corresponding base64 encoded letters.
Consider the example below.
Suppose we have a Base64 encoded string U3Vubnk=
, which must go through Base64 decoding. The first step requires conversion of it into its decimal form.
20 55 21 46 27 39 36=
These decimal characters need to get converted to their 6-bit binary form.
010100, 110111, 010101, 101110, 011011, 100111, 100100, 00
Segregating it into 8-bit:
01010011, 01110101, 01101110, 01101110, 01111001, 00000000
The last group is appended with 4 extra zeroes to make it 8-bit. It does not affect the result. Now, these 8-bit binaries need to get converted to their decimal format.
83 117 110 110 121 0
Lastly, we will convert these decimal digits to their corresponding letters using the standard conversion chart.
S u n n y
Decode Base64 Data Manually in Python
These are the steps to follow to decode Base64 manually in Python.
-
Import Base64 library package. This library package aids in encoding-decoding Base64 numbers in Python.
-
Declare a string variable
input_string
and put the encoded value ‘U3Vubnk=’ as input in it. -
The variable,
base64_converted_string
, takes the value ‘U3Vubnk=’ and turns it into its ASCII values. -
In the 4th step, we use the variable
decode
to convert ASCII values into their Base64 value. The syntax below converts the ASCII values to their Base64 values.base64.b64decode(base64_converted_string)
-
In the final step, the variable
decimal_converted_string
is used to decode the Base64 values into their corresponding ASCII characters.Syntax:
variable_name.decode("ascii")
In the program below, decode
is the variable that stores the Base64 decoded value. We need to print the variable decimal_converted_string
to see the decoded result.
Code:
import base64
input_string = "U3Vubnk="
base64_converted_string = input_string.encode("ascii")
decode = base64.b64decode(base64_converted_string)
decimal_converted_string = decode.decode("ascii")
print(decimal_converted_string)
Output:
Sunny
Decode Base64 Data Using UTF-8 in Python
The UTF-8 method is a more straightforward approach to decoding Base64 using python. Let’s see how this works.
-
We first need to import the Base64 library package like the above program.
-
The variable
input_string
is used to store the value that needs to get decoded. -
We use the variable
decoded_value
to convert the input string to its corresponding binary array values. The method used to interpret this string is UTF-8, which is very similar to ASCII. -
The string is interpreted into its 8-bit binary values. Other interpreting formats can be chosen as well.
-
To see the result, we print the variable
decoded_value
.
Code:
import base64
input_string = "U3Vubnk="
decoded_value = base64.b64decode(input_string).decode("utf-8")
print(decoded_value)
Output:
Sunny
Decode an Image Using Base64 in Python
The last segment will discuss practical uses of Base64 decodings, such as Base64 image encoding and decoding. We will look at how images are encoded into their Base64 codes using Python and how it is decoded back, as well.
We have an image named cheers.jpg
which will be encoded.
Encode an Image Using Base64 in Python
Import the Base64 library package. The syntax with open("cheers.jpg", "rb")
is a Base64 function that stores the image’s bytes in an object named photo2code
that we created.
The Base64 coded values of the image are stored in the variable coded_str
. The syntax photo2code.read()
reads the byte values of the image, and the function base64.b64encode(objectname.read()
converts the byte values into Base64 coded values.
Finally, coded_str
is printed to see the Base64 output.
Code:
import base64
with open("cheers.jpg", "rb") as photo2code:
coded_str = base64.b64encode(photo2code.read())
print(coded_str)
Here, the output is a long ciphertext. You can execute the above code to produce the result on your machine.
Decode a Base64 Code to Its Image Form in Python
Decoding an image file requires two main steps:
-
Reading the Base64 data, and
-
Decoding and writing that data into an image file.
Reading the Base64 Data
A writable .bin file is created to store the Base64 values for this step.
The syntax with open('file_name, "wb") as file:
creates a writable ("wb"
) .bin
file, in which we store the Base64 values. The file.write(coded_str)
syntax simply writes those Base64 values into that .bin file.
Here, the variable coded_str
is used in the above program. The above program can be used to recreate the coded_str
variable, but a .txt file can also be used.
All it needs is to put the file having Base64 values in the syntax, with open('(filename.extension)', "wb") as file:
, and the file will be loaded in the program. It should be made sure that the file is in the same directory where the python.txt file is stored, or the system won’t interact with it.
Decode Base64 Values and Write Into an Image File
Here, we will use the values inside the .bin file to decode. Variable decoder
is created that loads the .bin file through the syntax decoder = open('pic_encoding.bin', 'rb')
.
The file is loaded as a readable entity because we won’t be writing anything in this file anymore. Then the contents of the decoder are read by a variable using the syntax, read_b64 = decoder.read()
.
The variable final_decoder
is used to create a new writable .jpeg file where we will be storing our decoded Base64 values.
Finally, we decode and write the contents into a new image file.
Let’s recall the steps initiated so far to make everything crystal clear.
- We have Base64 values in the
coded_str
variable. - These values are written in the .bin file
pic_encoding.bin
. - Then, the data is loaded from the .bin file into the variable decode.
- We use variable
read_64
to read encoded values stored in the decoder variable. - Finally, we create an empty image file
pic_decoded_back.jpeg
and variablefinal_decoder
that will act as a funnel to transfer decoded data into the image file.
When all the above steps are initiated, we decode the binary values and write those values in the image file using the syntax,
final_decoder.write(base64.b64decode((read_b64)))
The Base64 function took the values from the variable read_b64
and transferred them into the image file through the variable final_decoder
.
The program will generate a new image file in the same directory where the python.txt file is present if appropriately executed.
Code:
import base64
# writing the base64 values in a writable file
with open("pic_encoding.bin", "wb") as file:
file.write(coded_str)
# decoding
decoder = open("pic_encoding.bin", "rb")
read_b64 = decoder.read()
final_decoder = open("pic_decoded_back.jpeg", "wb")
final_decoder.write(base64.b64decode((read_b64)))