How to Calculate Cross Correlation in Python
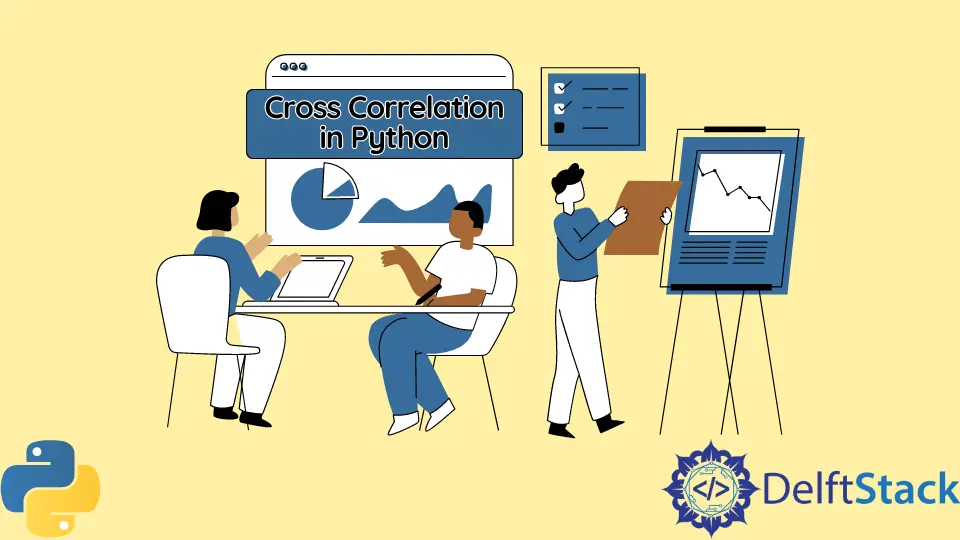
Cross-correlation is an essential signal processing method to analyze the similarity between two signals with different lags. Not only can you get an idea of how well the two signals match, but you also get the point of time or an index where they are the most similar.
This article will discuss multiple ways to process cross-correlation in Python.
Cross-Correlation in Python
We can use Python alone to compute the cross-correlation of the two signals. We can use the formula below and translate it into a Python script.
Formula:
R_{fg}(l) = \sum_{n=0}^{N} f(n)g(n+l)
Example Code:
sig1 = [1, 2, 3, 2, 1, 2, 3]
sig2 = [1, 2, 3]
# Pre-allocate correlation array
corr = (len(sig1) - len(sig2) + 1) * [0]
# Go through lag components one-by-one
for l in range(len(corr)):
corr[l] = sum([sig1[i + l] * sig2[i] for i in range(len(sig2))])
print(corr)
Output:
[14, 14, 10, 10, 14]
Now, let’s go through multiple Python packages that use cross-correlation as a function.
Use NumPy
Module
The standard Python module for numerical computing is called NumPy
. It is not surprising that NumPy
has a built-in cross-correlation technique. If we don’t have NumPy
installed, we can install it with the command below:
pip install numpy
Example Code:
import numpy as np
sig1 = [1, 2, 3, 2, 1, 2, 3]
sig2 = [1, 2, 3]
corr = np.correlate(a=sig1, v=sig2)
print(corr)
Output:
[14 14 10 10 14]
Use SciPy
Module
When NumPy
fails, SciPy
is the main package to consider. It includes practical techniques for numerous engineering and scientific disciplines.
But first, we must import the cross-correlation-related signal processing software. Then, the signal is automatically padded at the start and finish by the SciPy
cross-correlation.
As a result, compared to our pure Python code and the NumPy
module, it provides a more extensive signal response for cross-correlation. Therefore, we deleted these padding components to make the outcome equivalent in our test case.
If we don’t have SciPy
installed, we can install it with the command below:
pip install scipy
Example Code:
import scipy.signal
sig1 = [1, 2, 3, 2, 1, 2, 3]
sig2 = [1, 2, 3]
corr = scipy.signal.correlate(sig1, sig2)
# Removes padded Correlations
corr = corr[(len(sig1) - len(sig2) - 1) : len(corr) - ((len(sig1) - len(sig2) - 1))]
print(corr)
Output:
[14 10 10]
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn