How to Execute Python Script via Crontab
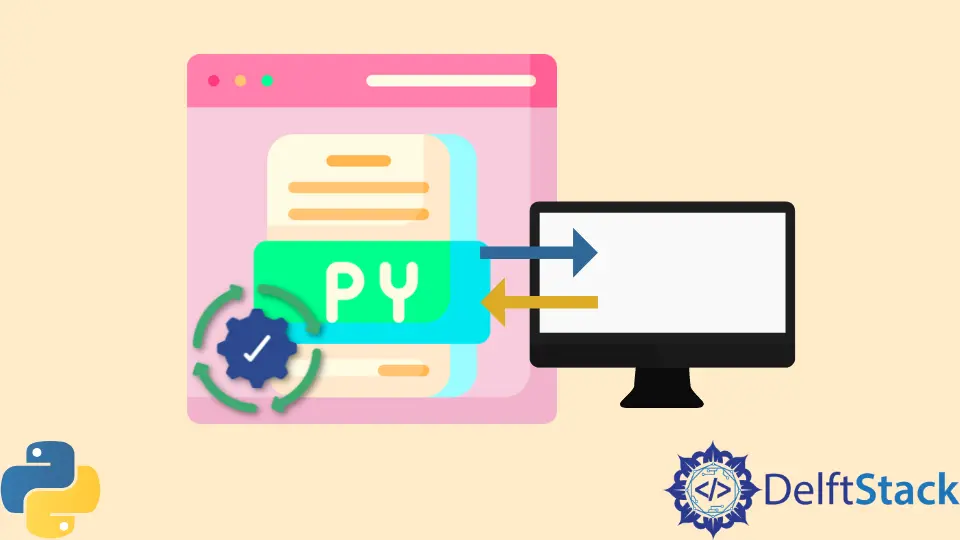
More often than not, we find ourselves in situations where processes need to be automated for efficiency and our sanity. It could be anything from having a regular email sent to employees to having a license for some application renewed every three months.
Crontab is derived from the Cron table. Cron is an automation utility offered by the Unix, Solaris, and Linux operating systems that helps with automation.
In this article, we will be exploring Crontab and how we can use it to run a Python script.
Crontab in Linux/Unix/Solaris
Cron is a daemon that runs when a system boots from the /etc/init.d
scripts. If required, we can also start/restart or stop the daemon using the init
script or via crond
, which is essentially a command service found in Linux and its other flavors.
A Crontab or Cron table is a file containing a myriad of commands in the form of a list. These commands or cron entries are set to be run at specific times.
The commands in this file, commonly known as cronjobsCrontabs
, are typically used for managing, maintaining, or administering a system. They can also be used for other tasks that are required regularly, for example, backups.
Each user can have a Crontab of their own.
Each Crontab file has five fields that specify the minutes, hours, day of the month (date), month, day, and lastly, all of this is followed by the command that is supposed to be executed at the previously specified time.
Some tips for efficiently maintaining a Crontab are:
- Insert a space to separate each field.
- Insert a comma to separate multiple values.
- Insert a hyphen (
-
) to designate a range of values. - Insert an asterisk (
*
) to include all possible values for the field. - Use a hash (
#
) at the start of a line to add comments or insert blank lines in the file.
Cron Job or Cron Schedule in Linux/Unix/Solaris
A Cron Job or Cron Schedule is a particular set of instructions or commands that specify the time and day to execute the commands. There can be multiple execution commands inside a cron table.
Execute Python Scripts via Crontab
A Python script can be executed with the help of a Crontab by following these simple steps:
-
Find or define the Python script you wish to execute.
-
Execute the command
crontab -e
in the terminal you want to work with. -
Now, you need to press
i
to enter into the edit mode. -
Input the scheduled command wherein you can specify the path of the Python script you wish to execute.
-
You can press the escape button to exit the edit mode.
-
Use the command
:wq
to create and write a Crontab.
Examples of Crontab
Here are some examples of what a Crontab would look like for various scenarios:
-
Removing some temp files daily at a particular time from a specific location or path in the computer:
30 18 * * * rm /home/someuser/tmp/*
-
Some commands to be executed every 7th day:
0 0 */7 * * shell_command_here
-
Executing a script in the system every minute:
* * * * * cd/Users/name/Automation && /usr/bin/python testing_crontabs.py
Let’s now create a script of our own and have it executed via Crontab. Following is the Python script we will be using for this article:
Example Code:
#! /usr/bin/python3
import sys
from datetime import datetime
def main(args):
result = 1
for arg in args[1:]:
result *= int(arg)
print(f"Result: {result} on: {datetime.now()}.")
if __name__ == "__main__":
main(sys.argv)
Output:
Now, we execute the command sudo chmod +x main.py
in the terminal of our choice to make the file executable.
Now, execute crontab -e
in the terminal, and when asked to select an editor from the options provided, we choose nano for this article and add the following cron command:
*/2 * * * * /home/$(USER)/myscript.py 1 2 3 4 5 >> /home/$(USER)/outputfile.txt
We need to replace USER
with the username for our specific machine. Save and exit from the editor, and our Crontab is now ready and working.
How do we know? According to our Crontab, the script should run every two minutes.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn