How to Calculate and Display a Convex Hull in Python
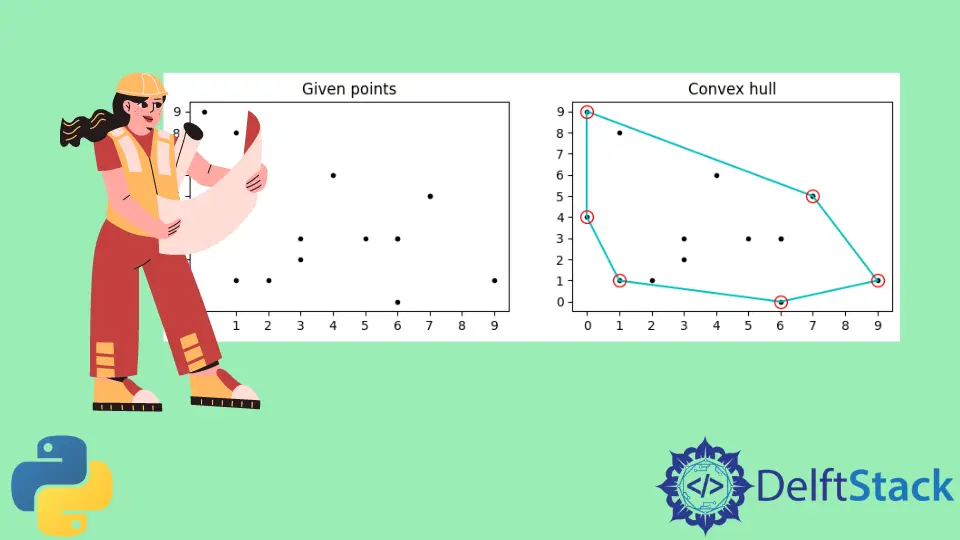
A convex object is an object that has no interior angles greater than 180 degrees. Hull is the exterior part of the object.
Thus, the Convex Hull means the boundary around the shape of the convex object. This tutorial will teach you to calculate and display a Convex Hull of a random set of points in Python.
Calculate and Show a Convex Hull in Python
A convex hull of a set of points is the boundary of the smallest convex polygon that consists of all points in a set.
Let’s see an example of a convex hull of a set of points.
Given these points:
The convex hull is:
The following is a simple implementation of displaying a convex hull of random points in Python.
Importing the required modules:
from scipy.spatial import ConvexHull
import matplotlib.pyplot as plt
import numpy as np
Using random points in 2-D:
points = np.random.randint(0, 10, size=(15, 2))
For a convex hull, we have:
hull = ConvexHull(points)
Now, let’s plot the points and convex hull.
fig, (ax1, ax2) = plt.subplots(ncols=2, figsize=(10, 3))
for ax in (ax1, ax2):
ax.plot(points[:, 0], points[:, 1], ".", color="k")
if ax == ax1:
ax.set_title("Given points")
else:
ax.set_title("Convex hull")
for simplex in hull.simplices:
ax.plot(points[simplex, 0], points[simplex, 1], "c")
ax.plot(
points[hull.vertices, 0],
points[hull.vertices, 1],
"o",
mec="r",
color="none",
lw=1,
markersize=10,
)
ax.set_xticks(range(10))
ax.set_yticks(range(10))
plt.show()
Output:
Now you should know generating a set of random points and display a convex hull in Python. We hope you find this tutorial helpful.