Command-Line Arguments in Python
-
Use the
sys
Module to Process Command Line Arguments in Python -
Use the
getopt
Module to Process Command Line Arguments in Python -
Use the
argparse
Module to Process Command Line Arguments in Python
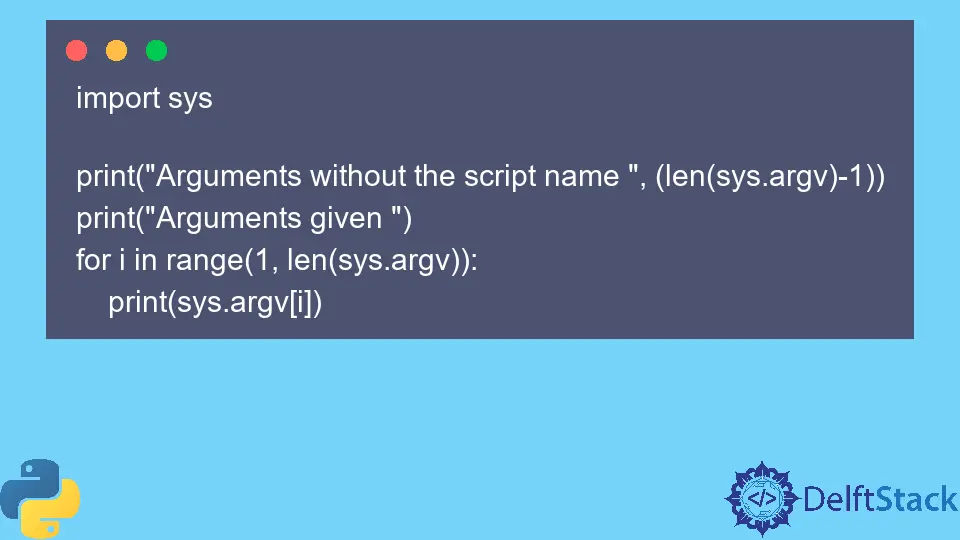
Command-line arguments are those values that are supplied in the command line window while running the file. They are accommodating because it allows us to pass the values while executing the program.
In Python, different modules can be used for processing command-line arguments, which are discussed below.
Use the sys
Module to Process Command Line Arguments in Python
sys.argv
is used to store the arguments supplied in the command line in a list-type structure. The name of the file is considered as the first argument.
For example,
import sys
print("Arguments without the script name ", (len(sys.argv) - 1))
print("Arguments given ")
for i in range(1, len(sys.argv)):
print(sys.argv[i])
In the above example, we pass some arguments at runtime and display the total number of arguments and their values.
Use the getopt
Module to Process Command Line Arguments in Python
The getopt
module also processes command line arguments. It allows us to validate the values given. We can provide values with the argument along with option strings that the script can recognize. This module works with the sys module for better processing.
The getopt()
constructor is used to initialize the object, which parses the arguments. It returns a getopt.error
when the option is not recognized from the argument list.
We use it in the code below.
import getopt
import sys
lst = sys.argv[1:]
options = "abc:"
long_options = ["A_Message", "B_Message", "C_Message"]
try:
arguments, values = getopt.getopt(lst, options, long_options)
for currentArgument, currentValue in arguments:
if currentArgument in ("-a", "--A_Message"):
print("Message A received")
elif currentArgument in ("-b", "--B_Message"):
print("Message B received")
elif currentArgument in ("-c", "--C_Message"):
print("Message C received")
except getopt.error as err:
print("Error")
Use the argparse
Module to Process Command Line Arguments in Python
The argparse module is by far the most convenient module to work with command-line arguments. This module offers a lot of flexibility since it can handle positional arguments, optional arguments and also specify their type.
We can put some default values in case some argument is missing and even display a help message.
The ArgumentParser()
function creates a parser object. The parse.args()
function reads the arguments from the command-line.
For example,
import argparse
parser = argparse.ArgumentParser(description="Which Message?")
parser.add_argument("a")
parser.add_argument("b")
args = parser.parse_args()
print(args.a, args.b)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn