Collatz Sequence in Python
- Programming Logic Behind Collatz Sequence
-
Collatz Sequence in Python Using
if-else
-
Collatz Sequence in Python Using
while
Loop and Exception Handling - Collatz Sequence in Python Using Recursion
-
Collatz Sequence in Python Using
while
Loop - Conclusion
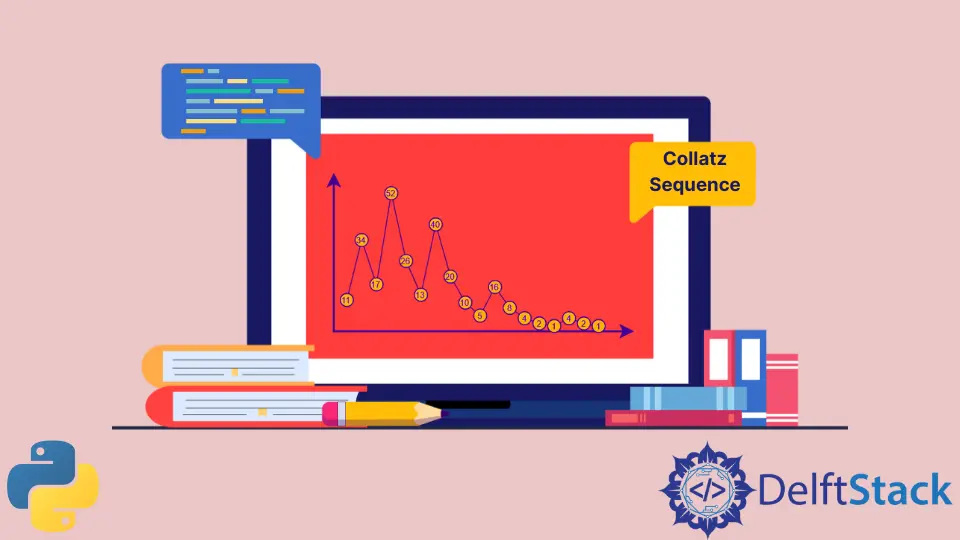
Collatz sequence is a mathematical sequence of numbers that ends up as 1. It is said that when a number undergoes a specific set of operations, the last digit left must be 1.
This article will explain how to write programs that find the collatz sequence in Python on any given number.
Programming Logic Behind Collatz Sequence
Four operations need to be followed to find a given number’s collatz sequence in Python.
- Checking if the number is even or odd.
- For an even number, the program divides the number
n
by 2 and prints it. - For an odd number, the program multiples
n
with 3, then adds 1 to it and prints it. - The program checks if the number
n
has reduced to 1; if not, it runs again.
For a given number 5, the collatz sequence will be - 16, 8, 4, 2, 1.
The following sections will explain four ways to create a program that displays collatz sequences in Python.
Collatz Sequence in Python Using if-else
The program below shows a simple representation to find a collatz sequence in Python. A method collatz_seq
is created with a single parameter numb
.
The logic used in this program uses an if-else
statement inside a while
loop. At first, the program runs a while
loop inside the method collatz_seq
to check if the value inside variable numb
is 1 or not.
If the variable numb
is not 1, then the program runs a second check to find if the given number is even or odd using an if-else
statement. Inside the if
statement, the program checks if the number divided by 2 leaves any remainder.
if numb % 2 == 0:
pass
If the number is even, the program divides it by 2 and prints it. In case the number is odd, the program shifts to the elif
statement where numb % 2 == 1
is true
.
The variable numb
is multiplied by 3 and added to 1. Lastly, the number is printed.
if numb % 2 == 0:
pass
elif numb % 2 == 1:
numb = (numb * 3) + 1
print(numb)
This sequence of loops keeps on running the operations of checking, updating, and printing on the number until the value of numb
is reduced to 1
and when the value of numb
is found as 1
, the while
loop exits.
Lastly, the method collatz_seq
is called, and 5
is passed for the argument’s value.
def collatz_seq(numb):
while numb != 1:
if numb % 2 == 0:
numb = numb // 2
print(numb)
elif numb % 2 == 1:
numb = (numb * 3) + 1
print(numb)
collatz_seq(5)
It shows the collatz sequence in Python for an input = 5
is:
16
8
4
2
1
Collatz Sequence in Python Using while
Loop and Exception Handling
The previous example demonstrated a program that prints a given number’s collatz sequence in Python. But the program has lots of loose ends that can lead to crashes.
For instance, the program crashes if a string is provided for the value of numb
. Similarly, the program crashes if a negative integer is provided as input.
A program must have a failsafe procedure to filter entered data to solve it.
In this program to find the collatz sequence in Python, the library package sys
is imported. The sys
system function exits programs when a condition is met.
A method collatz_seq
is created with a parameter numb
just like the last program.
import sys
def collatz_seq(numb):
An if-elif
statement checks if the number is even or odd. If the number is even, the number gets divided by 2, and the new number is stored inside the variable value
.
If the number is odd, the variable value
is stored with a value multiplied and added by 3 and 1, respectively.
if numb % 2 == 0:
value = numb // 2
elif numb % 2 != 0:
value = (3 * numb) + 1
Unlike the last program, the updated result is stored inside the value
variable. After the if-elif
block, two while
loops are created.
The first while
loop checks if the value of the variable value
is 1 and then prints and exits the loop if the case is found true
. If value
is not 1
, then the second while
loop prints the value
and copies it inside the variable numb
.
while value == 1:
print(value)
sys.exit()
while value != 1:
print(value)
n = value
Lastly, the method collatz_seq
is called recursively with the argument numb
. The method will keep calling itself until the value
is reduced to 1
, then the program exits using the sys.exit()
function.
return collatz_seq(numb)
Outside the method collatz_seq
, a variable number
is created that takes user input. An exception handling block is added to catch invalid inputs.
Inside the try
block, the variable number
is taken as an integer input. An if
statement is used to check if the number is negative or not.
If found true
, the polarity of the number is changed, and a suitable message is printed. Lastly, the method collatz_seq
is called.
print('Enter a number: ')
try:
number = int(input())
if number < 0:
number = abs(number)
print("A negative number entered")
collatz_seq(number)
Inside the except
block, a ValueError
is raised for inputs other than an integer, along with an error message.
except ValueError:
print('You must enter an integer type.')
The complete code is given below.
import sys
def collatz_seq(numb):
if numb % 2 == 0: # Remainder = 0, Checks for even number
value = numb // 2
elif numb % 2 != 0: # Remainder = 1, Checks for odd number
value = (numb * 3) + 1
while value == 1: # If value is 1
print(value)
sys.exit() # Program exits
while value != 1: # The loop runs until the value is not 1.
print(value)
numb = value # Value of value is copied to numb
return collatz_seq(numb)
print("Enter a number: ") # Input statement
try:
number = int(input()) # Typecast as Integer Input
if number < 0:
number = abs(number)
print("A negative number entered")
collatz_seq(number)
except ValueError:
print("You must enter an integer type.")
Output:
Enter a number:
10
5
16
8
4
2
1
For an invalid input:
Enter a number:
j
You must enter an integer type.
Collatz Sequence in Python Using Recursion
This program finds the collatz sequence in Python with a different method call. The with
block is created outside the collatz
method to let the program keep taking inputs for a given number of times.
The collatz
method checks if the number is even or odd and performs operations on it like the previous program. Outside the method, the program takes two inputs; the first takes a range of for
loop.
Inside a try
block, the second input is provided. The while
loop keeps calling method collatz
until the value of numb
is reduced to 1
.
Inside the while
loop, the value of numb
is passed and returned repeatedly.
def collatz_seq(numb):
if numb % 2 == 0: # even
print(numb // 2)
return numb // 2
elif numb % 2 != 0: # odd
value = 3 * numb + 1
print(value)
return value
c = int(input("Enter count: "))
for c in range(c):
try:
n = input("Enter number: ")
while n != 1:
n = collatz_seq(int(n))
except ValueError:
print("Value Error. Please enter integer.")
Output:
Enter count: 2
Enter number: 5
16
8
4
2
1
Enter number: 6
3
10
5
16
8
4
2
1
Collatz Sequence in Python Using while
Loop
So far, we have learned how to create a program that prints the collatz sequence in Python for a given number. In this program, the number of iterations also calculated that the program took to reduce the result to 1
.
A method solve
is created with a parameter numb
. Inside an if
statement, the program returns 0
if the parameter is given a value = 0
.
A new variable, length
, is created, which will be used to count the number of iterations.
A variable length
is created and assigned 1
to it. A while
loop is created with exit condition numb != 1
. This keeps the loop running until the value of numb
is 1
.
Inside the while
loop, the operations on numb
are executed by a single statement. The numb
gets divided by 2
if it is even, else (3 * numb + 1)
.
The operations are performed in a single statement so that the value of length
is updated with each iteration.
numb = (numb / 2) if numb % 2 == 0 else (3 * numb + 1)
The current value of numb
and length
is printed, and then length
is incremented by 1
. The program checks for even or odd numbers, updates numb
, and prints it.
Then the length
is incremented for the next operation.
In the last step, the program increments an extra 1
to length
. This extra number is subtracted, and the final count of length
is returned.
Lastly, method solve
is called and passed an argument 10
, and the result is printed.
def solve(numb):
if numb == 0:
return 0
length = 1
while numb != 1:
numb = (numb / 2) if numb % 2 == 0 else (3 * numb + 1)
print("Count = ", length, "Value = ", numb)
length += 1
return length - 1
print("Total iterations = ", solve(10))
Output:
Count = 1 Value = 5.0
Count = 2 Value = 16.0
Count = 3 Value = 8.0
Count = 4 Value = 4.0
Count = 5 Value = 2.0
Count = 6 Value = 1.0
Total iterations = 6
Conclusion
This article provides various examples of creating programs that display the collatz sequence in Python. After going through the article, the reader can create a collatz sequence in Python in multiple ways.