How to Call Java From Python
-
Call Java From Python Using
Pyjnius
-
Call Java From Python Using
Javabridge
-
Call Java From Python Using
JPype
-
Call Java From Python Using
Py4J
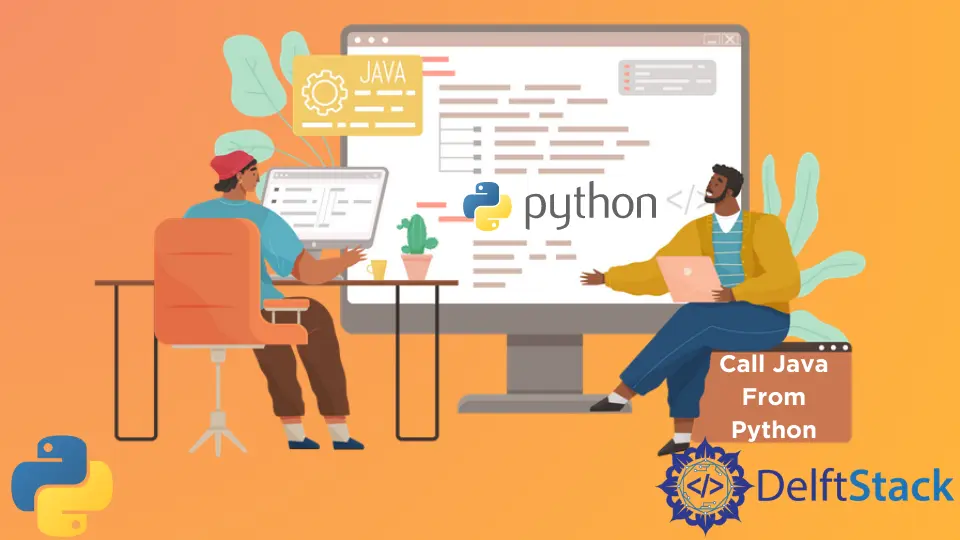
In our previous article, we tackled how to use Jython
to call Java from Python, which you can read more in this link.
However, there are multiple ways to call Java from Python. Therefore, this article will discuss several ways to call Java from Python.
Call Java From Python Using Pyjnius
Pyjnius
uses reflection to provision Java classes into the Python runtime and is based on the Java native interface.
Prerequisites:
-
Have Java installed, preferably OpenJDK 11.
-
Have
javac
installedapt-get install openjdk-11-jdk-headless
-
Install the
pyjnius
modulepip install pyjnius==1.4.1
Our example code snippet below creates a reference to the Java class System
and then calls the out.println static
method with the input Java in Python Success
.
from jnius import autoclass
autoclass("java.lang.System").out.println("Java in Python Success")
Let’s run this using the following command.
python main.py
Output:
Java in Python Success
Call Java From Python Using Javabridge
The Javabridge
library loads a Java class, org.cellprofiler.javabridge.CPython
, that we can use to execute Python code.
We can use the class within Java code called from the Python interpreter or within Java to run Python embedded in Java.
Install Javabridge
with the following command.
pip install javabridge
Maintaining references to Python objects may be desirable while running multiple scripts. The following routines, for instance, allow a Java caller to refer to a Python value (a base type or an object) using a token that we may swap out at any point for the actual value.
The Java code manages the lifetime of the reference.
import os
import javabridge
javaclasspath = (
[
os.path.realpath(
os.path.join(os.path.dirname(__file__), "..", "build", "classes")
)
]
+ [os.path.realpath(os.path.join(os.path.dirname(__file__), "..", "cfg"))]
+ [
os.path.realpath(
os.path.join(os.path.dirname(__file__), "..", "lib", name + ".jar")
)
for name in ["hamcrest-core-1.1", "logback-core-0.9.28", "slf4j-api-1.6.1"]
]
+ javabridge.JARS
)
javabridge.start_vm(class_path=[os.pathsep.join(javaclasspath)], run_headless=True)
try:
print
javabridge.run_script(
'java.lang.String.format("Hello, %s!", greetee);', dict(greetee="world")
)
finally:
javabridge.kill_vm()
Call Java From Python Using JPype
JPype
is a Python module that offers complete Java access from Python. This shared memory-based solution provides access to the whole CPython
and Java libraries while achieving good computation speed.
This method also enables the usage of Java threading and Java interface implementation in Python, as well as direct memory access between the two machines.
Install JPype
with the following command.
pip install jpype1
Once installed, you can now start importing the jpype
module.
import jpype as jp
jp.startJVM(jp.getDefaultJVMPath(), "-ea")
jp.java.lang.System.out.println("hello world")
jp.shutdownJVM()
Call Java From Python Using Py4J
With Py4J
, Java objects in a Java Virtual Machine can be dynamically accessed by Python applications running in a Python interpreter.
The method is invoked as though the Java objects were present in the Python interpreter, and Java collections are accessible via standard Python collection methods. Py4J
also makes it possible for Java programs to call Python objects back.
To install Py4J
, run the following snippet.
pip install py4j
#or
easy_install py4j
Here is a short snippet of what you can do with Py4J
.
from py4j.java_gateway import JavaGateway
gateway = JavaGateway() # connect to the JVM
java_object = gateway.jvm.mypackage.MyClass() # invoke constructor
other_object = java_object.doThat()
other_object.doThis(1, "abc")
gateway.jvm.java.lang.System.out.println("Hello World!")
Unlike Jython
, a portion of Py4J
runs in the Python Virtual Machine (VM), keeping it updated constantly with the latest version of Python and allowing you to use libraries that Jython
does not support properly (e.g., lxml
). Therefore, you should call the Java VM where the other portion is running.
Python communicates through sockets instead of JNI, and Py4J
has its protocol (to optimize certain cases, manage memory, etc.).
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn