How to Call External Program in Python
-
Use the
os.system()
Function to Execute External System Commands in Python -
Use the
os.popen()
Function to Execute External System Commands in Python -
Use the
subprocess.Popen()
Function to Execute External System Commands in Python -
Use the
subprocess.call()
Function to Execute External System Commands in Python -
Use the
subprocess.run()
Function to Execute External System Commands in Python - Conclusion
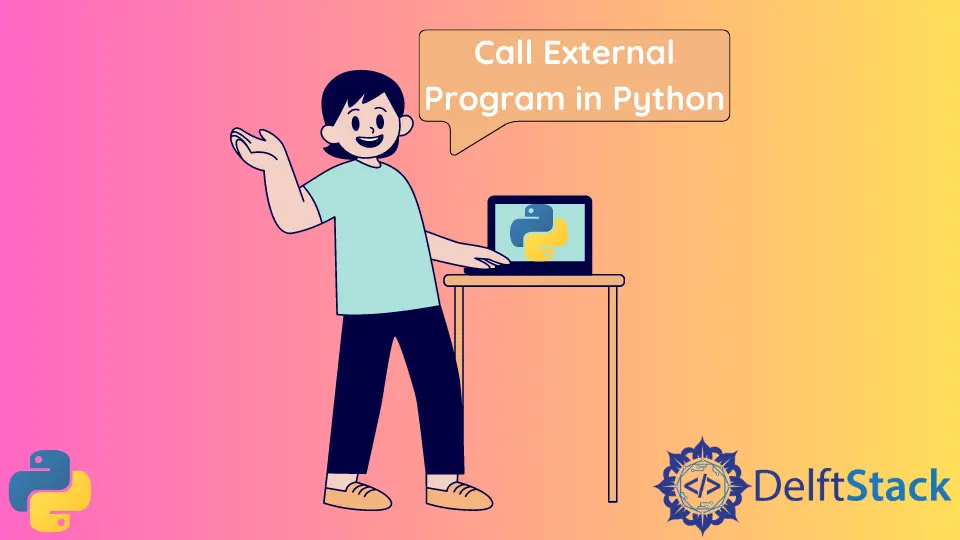
This comprehensive guide delves into various methods provided by Python to execute system commands, each with its unique features and use cases. Ranging from the straightforward os.system()
to the more sophisticated subprocess.run()
, Python offers a versatile toolkit for interacting with the system’s shell and external programs.
Understanding these methods is crucial for Python developers who need to extend their scripts’ capabilities beyond the Python environment, enabling them to leverage the full power of the underlying operating system.
Use the os.system()
Function to Execute External System Commands in Python
The os
module in Python can interact with the device’s OS and has functions available to execute shell commands. The system()
function implements a command passed to it as a string in a subshell.
It returns the exit status of the shell, which the OS returns after running the given command. It allows to run multiple commands at once and call external programs, not shell commands.
Example:
import os
# Command to echo 'Hello, World!'
command = "echo Hello, World!"
# Execute the command
return_code = os.system(command)
# Print the return code
print("Return code:", return_code)
In this simple script, we first import the os
module. We then define a string command
that holds our intended shell command.
Here, we’re using the echo
command, a standard command in most operating systems, to print Hello, World!
.
The os.system(command)
line executes this command. The output of the echo
command is displayed directly to the console.
After executing the command, os.system()
returns a code that represents the execution status, which we capture in return_code
and print. This return code helps in understanding if the command was executed successfully (indicated by 0
) or if there was an error.
Use the os.popen()
Function to Execute External System Commands in Python
Another function from the os
module to run external program commands is the popen()
function. This method opens a pipe related to the command.
It is similar to the os.system()
function, but it provides a file-type object that helps with input-output operations related to the command. We can specify the file mode for the file and the buff size as the parameter in the function.
Example:
import os
# Command to echo 'Hello, World!'
command = "echo Hello, World!"
# Open a pipe to the command
pipe = os.popen(command)
# Read the output of the command
output = pipe.read()
# Close the pipe
pipe.close()
# Print the output
print(output)
In our script, we start by importing the os
module, which is essential for using os.popen()
. We define the command
variable to hold the shell command that we wish to execute.
In this example, we use the echo
command to output the string Hello, World!
.
We then use os.popen(command)
to execute the command. This function opens a pipe to the command and returns a file-like object.
We read from this object using the read()
method, which captures the command’s output. After reading, it’s important to close the pipe using the close()
method to free up system resources.
Finally, we print the captured output.
Output:
Use the subprocess.Popen()
Function to Execute External System Commands in Python
The subprocess
module is similar to the os
module and has improved functions and methods for calling external programs. The Popen
is a class and not a method.
It is a little complicated to use this due to various parameters that need to be specified along with the required command. It combines all the 4 os.popen()
functions into one.
It also executes the external program as a separate process.
Example:
import subprocess
# Command to run
command = "echo Hello, World!"
# Run the command through the shell
process = subprocess.Popen(
command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, shell=True
)
# Capture the output and error
output, error = process.communicate()
# Decode and print the output
print(output.decode())
We first import the subprocess
module, essential for process creation and management in Python. We define our command, echo Hello, World!
, a simple shell command to display a message.
In the crucial step, we create a subprocess using subprocess.Popen
, passing our command as an argument and setting shell=True
to ensure the command is executed through the shell.
We then direct both the standard output and error to be captured via pipes, allowing us to manage the output within our Python script.
By calling process.communicate()
, we capture and subsequently decode the output from bytes to a string, enabling us to display the familiar Hello, World!
message in a Python-friendly format.
Output:
Use the subprocess.call()
Function to Execute External System Commands in Python
The call()
function is an improvement to the popen()
function.
However, unlike the popen()
function, it waits for the command to execute before providing the return code. We pass the command as an argument to the function.
Example:
import subprocess
# Define the command to run
command = "echo Hello, World!"
# Run the command through the shell
return_code = subprocess.call(command, shell=True)
# Print the return code
print("Return code:", return_code)
In our script, we first import the subprocess
module, providing us access to the subprocess.call()
function. We then define our command, a basic echo
command to output Hello, World!
.
This command is structured as a list where the first element is the command (echo
), and the subsequent elements are the arguments to this command.
We execute the command using subprocess.call(command)
. This function runs the command and waits until it is completed, then returns the exit code of the external program.
An exit code of 0
usually indicates success, while any non-zero value indicates an error.
Finally, we print the return code to the console. This is a simple but effective way to verify that our external command was executed successfully.
Output:
Use the subprocess.run()
Function to Execute External System Commands in Python
In Python v3.5 and above, the run()
function is more often used for running program commands. It returns a completed-process object after running the command, passed to the function as a parameter.
subprocess.run()
is designed for when you need to execute an external command, wait for it to complete, and then access its output, errors, and return code. It’s a higher-level interface than subprocess.Popen()
and is recommended for most use cases where you need to invoke a subprocess.
Example:
import subprocess
# Command to run
command = ["echo", "Hello, World!"]
# Run the command and capture the output
result = subprocess.run(command, capture_output=True, text=True)
# Print the output
print("Output:", result.stdout)
In our script, we first import the subprocess
module, which gives us access to the subprocess.run()
function. We then define the command as a list, where the first element is the executable (echo
), and the subsequent elements are its arguments.
We execute the command using subprocess.run()
, setting capture_output=True
to capture the output of the command and text=True
to treat input and output as strings rather than byte sequences. The function returns a CompletedProcess
object, which we store in result
.
Finally, we access the stdout
attribute of result
to print the captured output. Since we have set text=True
, result.stdout
is already a decoded string.
Output:
Conclusion
This exploration of Python’s capabilities to execute external system commands demonstrates the language’s flexibility and power in interacting with the operating system. From the basic os.system()
and os.popen()
methods to the more advanced subprocess
module’s Popen
, call
, and run
functions, Python provides a rich set of tools for various needs and complexities.
These functions enable Python scripts to communicate with external processes and the operating system, offering the ability to execute commands, capture outputs, and handle errors. The practical examples and detailed explanations provided aim to equip both novice and seasoned Python developers with the knowledge to effectively manage external processes in their applications.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn