How to Calculate the Average Word Length in a Sentence in Python
-
Use
split()
,sum()
, andlen()
to Calculate the Average Word Length in a Sentence in Python -
Use
split()
,sum()
,map()
, andlen()
to Calculate the Average Word Length in a Sentence in Python -
Use
split()
,len()
, andjoin()
to Calculate the Average Word Length in a Sentence in Python -
Use
len()
andreplace()
to Calculate the Average Word Length in a Sentence in Python
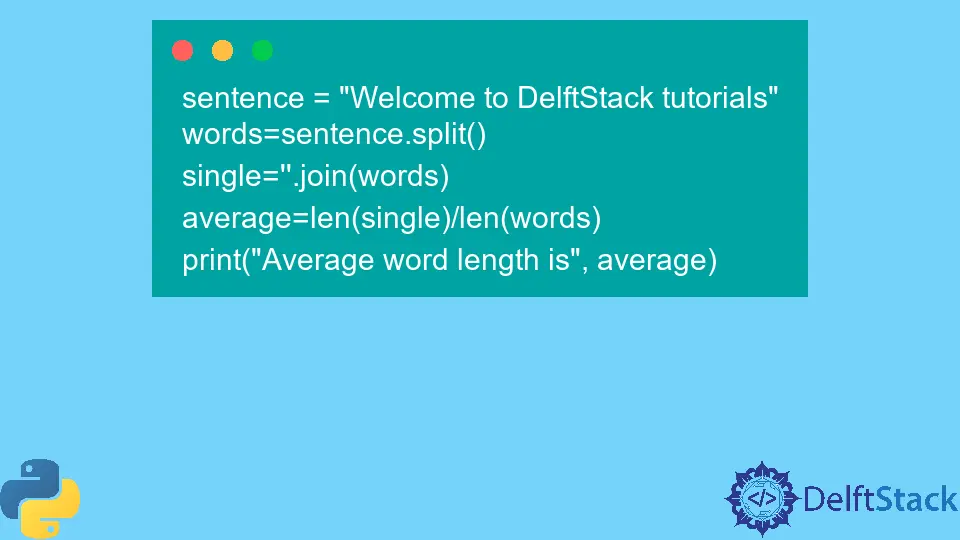
While working with strings in Python, sometimes you may need to know the average word length in a sentence. The average word length of a sentence is equal to the sum of the length of all characters to the sum of all words.
This tutorial will teach you to calculate the average word length in a sentence in Python.
Use split()
, sum()
, and len()
to Calculate the Average Word Length in a Sentence in Python
Calculating each word in a sentence and finding the average word length is impossible. But you can split it into a list and apply the function.
The split()
method in Python splits a string into a list where each word is a list item.
After splitting the string into a list, use the len()
function to get the average word length. The len()
function provides the number of items in a list.
The following example calculates the average word length in a given sentence.
sentence = "Welcome to DelftStack tutorials"
words = sentence.split()
avg = sum(len(word) for word in words) / len(words)
print("Average word length is", avg)
Output:
Average word length is 7.0
The sum()
function gets the sum of the length of all characters.
Use split()
, sum()
, map()
, and len()
to Calculate the Average Word Length in a Sentence in Python
This method is the same as above, but here we use the map()
function to get the length of the characters. The map()
function lets you apply a specified function to all items of an iterable.
As you can see, it replaces the for
loop in the first method.
sentence = "Welcome to DelftStack tutorials"
words = sentence.split()
average = sum(map(len, words)) / len(words)
print("Average word length is", average)
Output:
Average word length is 7.0
Use split()
, len()
, and join()
to Calculate the Average Word Length in a Sentence in Python
The join()
method joins all items in an iterable (list, tuple, string) into one string. In this method, we use split()
, len()
, and join()
to compute the average word length in a sentence.
sentence = "Welcome to DelftStack tutorials"
words = sentence.split()
single = "".join(words)
average = len(single) / len(words)
print("Average word length is", average)
Here, we split the sentence into a list and saved it in a variable, words
. Next, we use the join()
method to join the items in a list with an empty string.
The result is WelcometoDelftStacktutorials
, which is saved in a variable single
. Then we calculate the average word length by dividing the length of single
by the length of words
.
Output:
Average word length is 7.0
Use len()
and replace()
to Calculate the Average Word Length in a Sentence in Python
The replace()
method in Python replaces a specified text/character with a new text/character.
In this case, we replace the whitespaces with an empty string. And we compute the number of characters in a sentence using the len()
function.
Next, we divide the result by the number of items in a list object, giving the average word length in a sentence.
sentence = "Welcome to DelftStack tutorials"
average = len(sentence.replace(" ", "")) / len(sentence.split())
print("Average word length is", average)
Output:
Average word length is 7.0
Now you should know how to calculate a sentence’s average word length using the split()
command. The logic behind all its methods is quite similar.
You have also learned to use len()
and replace()
to determine the average word length. We hope you enjoy learning this simple Python program.