Async in Python
- Concept of Asynchronous Programming in Python
-
Implementing the
async
Keyword Using theasyncio
Module in Python
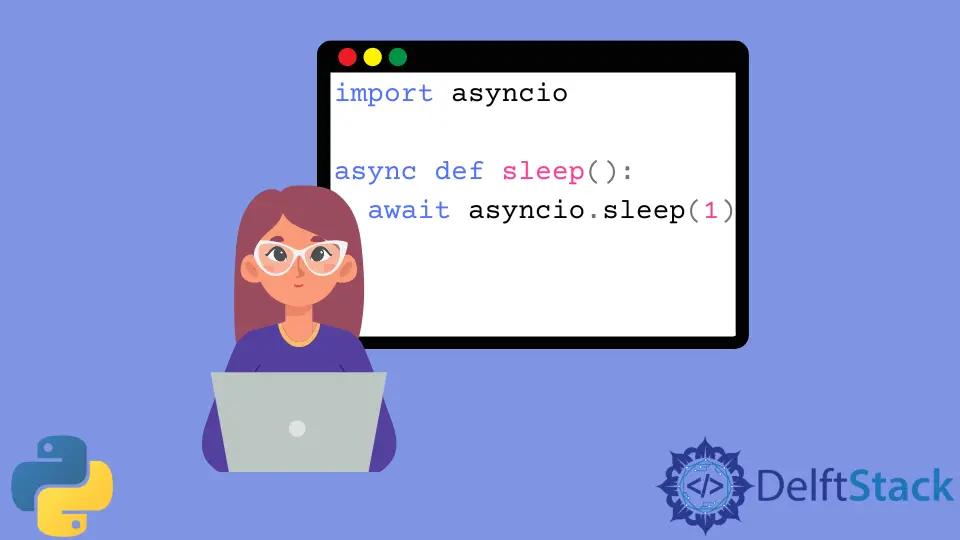
Asynchronous programming is a useful aspect of programming and can be implemented in Python with the help of the async IO
module.
This tutorial discusses the async IO
and how to implement it in Python.
Concept of Asynchronous Programming in Python
Asynchronous programming is a type of parallel programming that allows the designated part of the work to run separately from the primary thread where the application runs.
We utilize the asyncio
package as the foundation for several asynchronous frameworks in Python.
The asyncio
package in Python provides a much-needed foundation and a bunch of high-level APIs that help with the tasks of the coroutines.
Asynchronous programming has been strongly developed since its inclusion in Python programming with the version Python 3.5
. It has become the core for the popularity of some languages like Node.JS
as it relies on Asynchronous programming.
Implementing the async
Keyword Using the asyncio
Module in Python
In Python, the asyncio
module introduces two new keywords, async
and await
.
We will take an example code and provide its solution. Afterward, we will attempt the same problem by taking the Asynchronous programming approach through the asyncio
module.
Below is a snippet with the general approach.
import time
def sleep():
print(f"Time: {time.time() - begin:.2f}")
time.sleep(1)
def addn(name, numbers):
total = 0
for number in numbers:
print(f"Task {name}: Computing {total}+{number}")
sleep()
total += number
print(f"Task {name}: Sum = {total}\n")
begin = time.time()
tasks = [
addn("X", [1, 2]),
addn("Y", [1, 2, 3]),
]
termi = time.time()
print(f"Time: {termi-begin:.2f} sec")
Output:
Task X: Computing 0+1
Time: 0.00
Task X: Computing 1+2
Time: 1.00
Task X: Sum = 3
Task Y: Computing 0+1
Time: 2.00
Task Y: Computing 1+2
Time: 3.00
Task Y: Computing 3+3
Time: 4.00
Task Y: Sum = 6
Time: 5.02 sec
The general approach takes a total time of 5.02
seconds.
Now, let us use the asynchronous programming approach with the help of the asyncio
module in the following code block.
import asyncio
import time
async def sleep():
print(f"Time: {time.time() - begin:.2f}")
await asyncio.sleep(1)
async def addn(name, numbers):
total = 0
for number in numbers:
print(f"Task {name}: Computing {total}+{number}")
await sleep()
total += number
print(f"Task {name}: Sum = {total}\n")
begin = time.time()
loop = asyncio.get_event_loop()
tasks = [
loop.create_task(addn("X", [1, 2])),
loop.create_task(addn("Y", [1, 2, 3])),
]
loop.run_until_complete(asyncio.wait(tasks))
loop.close()
termi = time.time()
print(f"Time: {termi-begin:.2f} sec")
Output:
Task X: Computing 0+1
Time: 0.00
Task Y: Computing 0+1
Time: 0.00
Task X: Computing 1+2
Time: 1.00
Task Y: Computing 1+2
Time: 1.00
Task X: Sum = 3
Task Y: Computing 3+3
Time: 2.00
Task Y: Sum = 6
Time: 3.00 sec
We tweak the sleep()
function in the code when we add asyncio
to it.
Asynchronous programming takes the total time taken down to 3.0
seconds, as seen from the output above. This shows the need and the utility of asynchronous programming.
We have successfully implemented asynchronous programming on a simple Python code with the help of the asyncio
module.
Why do we need to utilize the asyncio
module when asynchronous programming can be implemented by utilizing the classic approach of Threads?
The answer would be that Python’s global interpreter lock limits the performance and the use of threads for instructions that do not utilize the interpreted code. There are no such issues with the asyncio
package as an external service is utilized.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn