Python 中的异步编程
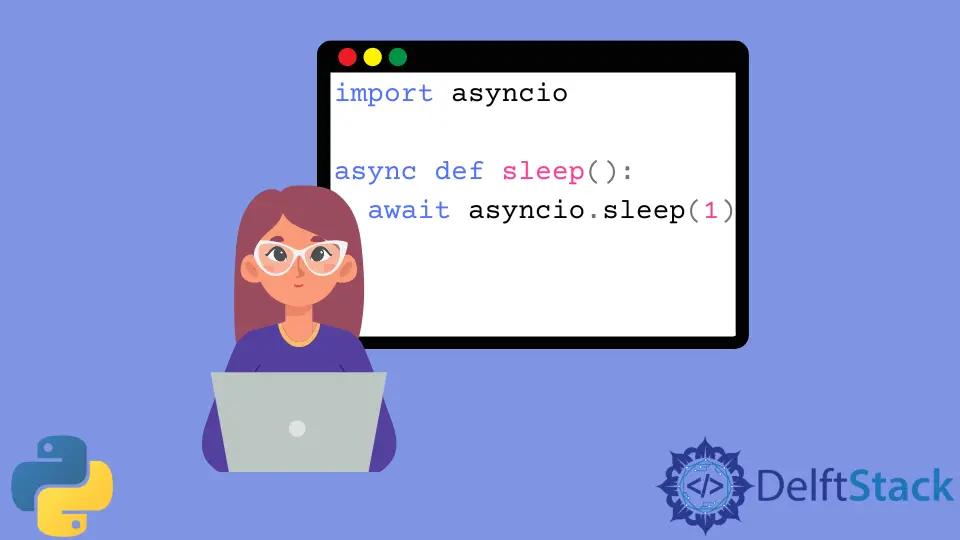
异步编程是编程的一个有用方面,可以借助 async IO
模块在 Python 中实现。本教程讨论异步 IO
以及如何在 Python 中实现它。
Python 中异步编程的概念
异步编程是一种并行编程,它允许工作的指定部分与运行应用程序的主线程分开运行。
我们利用 asyncio
包作为 Python 中几个异步框架的基础。
Python 中的 asyncio
包提供了一个急需的基础和一堆有助于协程任务的高级 API。
自从以 Python 3.5
版本包含在 Python 编程中以来,异步编程得到了强大的发展。由于它依赖于异步编程,因此它已成为诸如 Node.JS
等一些语言流行的核心。
在 Python 中使用 asyncio
模块实现 async
关键字
在 Python 中,asyncio
模块引入了两个新关键字,async
和 await
。
我们将举一个示例代码并提供其解决方案。之后,我们将通过 asyncio
模块采用异步编程方法来尝试相同的问题。
下面是一个带有一般方法的片段。
import time
def sleep():
print(f"Time: {time.time() - begin:.2f}")
time.sleep(1)
def addn(name, numbers):
total = 0
for number in numbers:
print(f"Task {name}: Computing {total}+{number}")
sleep()
total += number
print(f"Task {name}: Sum = {total}\n")
begin = time.time()
tasks = [
addn("X", [1, 2]),
addn("Y", [1, 2, 3]),
]
termi = time.time()
print(f"Time: {termi-begin:.2f} sec")
输出:
Task X: Computing 0+1
Time: 0.00
Task X: Computing 1+2
Time: 1.00
Task X: Sum = 3
Task Y: Computing 0+1
Time: 2.00
Task Y: Computing 1+2
Time: 3.00
Task Y: Computing 3+3
Time: 4.00
Task Y: Sum = 6
Time: 5.02 sec
一般的方法需要 5.02
秒的总时间。
现在,让我们在下面的代码块中借助 asyncio
模块使用异步编程方法。
import asyncio
import time
async def sleep():
print(f"Time: {time.time() - begin:.2f}")
await asyncio.sleep(1)
async def addn(name, numbers):
total = 0
for number in numbers:
print(f"Task {name}: Computing {total}+{number}")
await sleep()
total += number
print(f"Task {name}: Sum = {total}\n")
begin = time.time()
loop = asyncio.get_event_loop()
tasks = [
loop.create_task(addn("X", [1, 2])),
loop.create_task(addn("Y", [1, 2, 3])),
]
loop.run_until_complete(asyncio.wait(tasks))
loop.close()
termi = time.time()
print(f"Time: {termi-begin:.2f} sec")
输出:
Task X: Computing 0+1
Time: 0.00
Task Y: Computing 0+1
Time: 0.00
Task X: Computing 1+2
Time: 1.00
Task Y: Computing 1+2
Time: 1.00
Task X: Sum = 3
Task Y: Computing 3+3
Time: 2.00
Task Y: Sum = 6
Time: 3.00 sec
当我们添加 asyncio
时,我们在代码中调整 sleep()
函数。
从上面的输出中可以看出,异步编程将总时间缩短到 3.0
秒。这表明了异步编程的必要性和实用性。
在 asyncio
模块的帮助下,我们已经成功地在一个简单的 Python 代码上实现了异步编程。
当异步编程可以通过使用线程的经典方法实现时,为什么我们需要使用 asyncio
模块?
答案将是 Python 的全局解释器锁限制了线程的性能和使用不使用解释代码的指令。asyncio
包没有这样的问题,因为使用了外部服务。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn