__all__ in Python
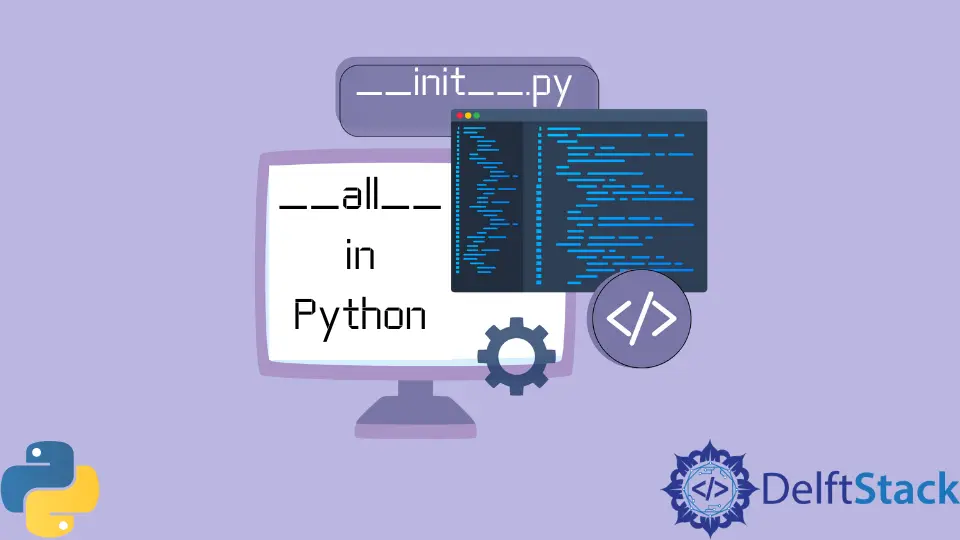
In the world of Python programming, understanding how to manage namespaces and control what gets imported from a module is crucial. One of the key features that help with this is the __all__
variable.
This tutorial dives into the concept of __all__
in Python, explaining its purpose and how it can be used effectively in your projects. Whether you are a beginner or an experienced developer, grasping the nuances of __all__
can enhance your code’s readability and maintainability. From controlling module exports to managing large codebases, let’s explore how __all__
can streamline your Python experience.
What is all in Python?
The __all__
variable is a list that defines what symbols a module will export when from module import *
is used. By default, all public symbols in a module are exported. However, by defining __all__
, you can control exactly which attributes and methods are accessible to users of your module. This is particularly useful in large projects where you want to encapsulate functionality and avoid namespace pollution.
Here’s a simple example to illustrate how __all__
works:
# my_module.py
__all__ = ['function_a']
def function_a():
return "Function A"
def function_b():
return "Function B"
When you import from my_module
, only function_a
will be accessible.
Output:
Function A
In this example, even though function_b
is defined in the module, it won’t be available for import when using the wildcard import method. This helps to keep your module clean and focused on the functionalities that you want to expose.
How to Use all in Your Modules
Using __all__
is straightforward. Simply define it at the top of your module as a list of strings, each representing the names of the attributes you wish to expose. Here’s a more detailed example:
# utilities.py
__all__ = ['add', 'subtract']
def add(x, y):
return x + y
def subtract(x, y):
return x - y
def multiply(x, y):
return x * y
In this case, if you perform a wildcard import:
from utilities import *
print(add(5, 3))
print(subtract(5, 3))
Output:
8
2
However, if you try to call multiply
, it will raise an error because it is not included in the __all__
list. This selective export helps maintain a clean API and prevents users from relying on functions that are intended to be private or internal.
Best Practices for Using all
When you decide to implement __all__
, consider the following best practices:
- Keep it Updated: Whenever you add or remove functions or classes, make sure to update the
__all__
list accordingly. - Limit Exports: Only include those functions or classes that are meant to be public. This helps prevent misuse and confusion.
- Document Your Exports: Provide clear documentation on what each exported symbol does. This is especially important for larger modules where the purpose of each function may not be immediately obvious.
Here’s an example that combines these practices:
# math_operations.py
__all__ = ['divide']
def divide(x, y):
if y == 0:
raise ValueError("Cannot divide by zero.")
return x / y
def square(x):
return x * x
def cube(x):
return x * x * x
In this case, only the divide
function is exposed. The other functions, square
and cube
, are kept private, ensuring that users of the module only have access to the intended functionality.
Output:
2.0
By following these best practices, you create a more robust and user-friendly module that is easier to maintain and understand.
Conclusion
Understanding and using __all__
in Python can significantly enhance your coding practices. By controlling what gets imported from your modules, you can create cleaner, more maintainable code. This feature helps manage complexity, especially in larger projects, allowing you to define a clear interface for your modules. So, the next time you’re working on a Python module, consider implementing __all__
to keep your code organized and user-friendly.
FAQ
-
What is the purpose of all in Python?
all is used to define a list of public symbols for a module, controlling what is imported when using wildcard imports. -
Can I use all with classes and variables?
Yes, all can include classes, functions, and variables, allowing you to manage all exports from a module. -
Is all mandatory in Python modules?
No, all is optional. If it is not defined, all public symbols are exported by default. -
How does all affect code readability?
By explicitly defining what is exported, all improves code readability and helps users understand the intended use of a module. -
Can all be used in packages?
Yes, all can be defined in modules within packages to control exports at different levels of the package structure.