3D Interpolation in Python
- Importance of Interpolation
- Install SciPy for Interpolation in Python
-
Use SciPy’s
interpn()
Method for 3D Interpolation in Python -
Use
RegularGridInterpolator
for 3D Interpolation in Python - Conclusion
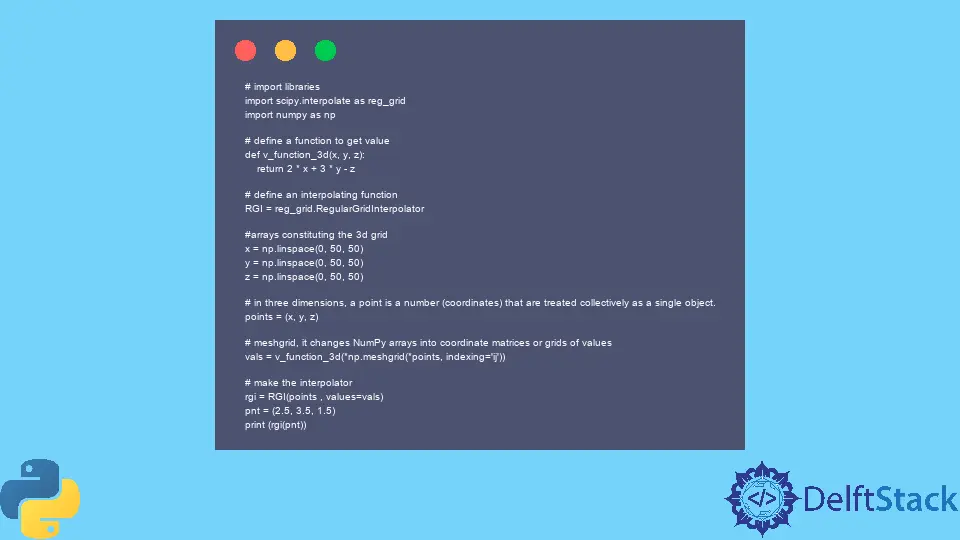
Interpolation is the method of constructing new data points within a defined range of a discrete set. Interpolation means finding value between points or curves.
From a mathematical point of view, interpolation is obtaining the value of specific unknown data points located between other known data points.
Importance of Interpolation
Interpolation is a powerful tool for making predictions, data analysis, and many other applications. It predicts unknown values for geographical-related data, such as noise level, rainfall, elevation, etc.
Interpolation has many uses. In Machine Learning (ML), we often deal with missing data in a dataset, and interpolation is often used to substitute those values.
Install SciPy for Interpolation in Python
To use interpolation in Python, we need to use the SciPy core library and, more specifically, the interpolation
module.
SciPy provides many valuable functions for mathematical processing and data analysis optimization. It provides useful functions for obtaining one-dimensional, two-dimensional, and three-dimensional interpolation.
We need to know how to install the SciPy library in Python to move forward.
To install SciPy, go to your command line interface and type the following command.
pip install scipy
Once it is successfully installed, we can import it into our Python program using the import
keyword.
Use SciPy’s interpn()
Method for 3D Interpolation in Python
We can perform 3D interpolation using the SciPy library’s interpn()
method. It means we can find three or higher dimensions with the help of this method.
Syntax of the interpn()
function:
scipy.interpolate.interpn(
points, values, xi, method="linear", bounds_error=True, fill_value=nan
)
Parameters of the interpn()
function:
-
points
: tuple ofndarray
of float, having shapes(m1, ),…,(mn, )
.A regular grid in
n
dimensions is defined through these points. The points in each dimension (i.e., every element of the points tuple) must be strictly ascending or descending. -
values
: array-like, shape(m1,…,mn,…)
.The data is on the regular grid in
n
dimensions. Complex data can be acceptable. -
xi
:ndarray
of shape(…,ndim)
.This is where the coordinates for sampling the gridded data are at.
-
method
: str, optionalThe methods of interpolation are
linear
,nearest
, andsplinef2d
.splinef2d
is only supported for 2D data. -
bounds_error
: bool, optionalIf it is true, when interpolated values are requested from outside of the domain of the input data, a
ValueError
is raised. If it is false, thenfill_value
is used. -
fill_value
: number, optional
If we provide a number, the function can use points outside the interpolation domain; if None
, the values outside the domain are extrapolated. Extrapolation is not supported by the method splinef2d
.
The interpn()
method returns values_x
: ndarray
, shape xi.shape[:-1] + values.shape[ndim:]
. The interpolated values.ndarray
is a multidimensional container where the shape length of each dimension and ndim
is the number of dimensions.
Now we have understood the interpn()
function and its parameters. Let’s give a practical example of it.
In this example, we pass three arguments to the interpn()
method.
Example Code:
# import libraries
from scipy.interpolate import interpn
import numpy as np
# define a function to get the value
def v_function_3d(x, y, z):
return 3 * x + 4 * y - z
# the np.linspace() function returns the interval between the given numbers.
x = np.linspace(0, 4, 5)
y = np.linspace(0, 5, 6)
z = np.linspace(0, 6, 7)
# in three dimensions, a point's coordinates are treated collectively as a single object.
points = (x, y, z)
# meshgrid, it changes NumPy arrays into coordinate matrices or grids of values
values = v_function_3d(*np.meshgrid(*points, indexing="ij"))
# coordinates to sample the gridded data are
point = np.array([2.21, 3.12, 1.15])
# evaluate the 3d interpolating function at a point
print(interpn(points, values, point))
Output:
[17.96]
Use RegularGridInterpolator
for 3D Interpolation in Python
Interpolation on a regular or rectilinear grid in any number of dimensions is performed using the class RegularGridInterpolator
.
A rectilinear grid, or a rectangular grid with regular or uneven spacing, is required to define the data. It supports linear, nearest-neighbor, and spline interpolations.
We can choose an interpolation method after setting up the interpolator object.
We use RegularGridInterpolator
when we linearly interpolate the surrounding grid points to interpolate. Input data in regularly spaced.
The following example explains how to use RegularGridInterpolator
for interpolation.
# import libraries
import scipy.interpolate as reg_grid
import numpy as np
# define a function to get value
def v_function_3d(x, y, z):
return 2 * x + 3 * y - z
# define an interpolating function
RGI = reg_grid.RegularGridInterpolator
# arrays constituting the 3d grid
x = np.linspace(0, 50, 50)
y = np.linspace(0, 50, 50)
z = np.linspace(0, 50, 50)
# in three dimensions, a point is a number (coordinates) that are treated collectively as a single object.
points = (x, y, z)
# meshgrid, it changes NumPy arrays into coordinate matrices or grids of values
vals = v_function_3d(*np.meshgrid(*points, indexing="ij"))
# make the interpolator
rgi = RGI(points, values=vals)
pnt = (2.5, 3.5, 1.5)
print(rgi(pnt))
Output:
13.999999999999998
Conclusion
We can use 3D
interpolation in Python with the help of the scipy
library and its method interpn()
or RegularGridInterpolator
. We can easily make predictions, data analysis, and many other applications.
Interpolation help users determine what data might exist outside the collected data.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn