The @ Symbol in Python
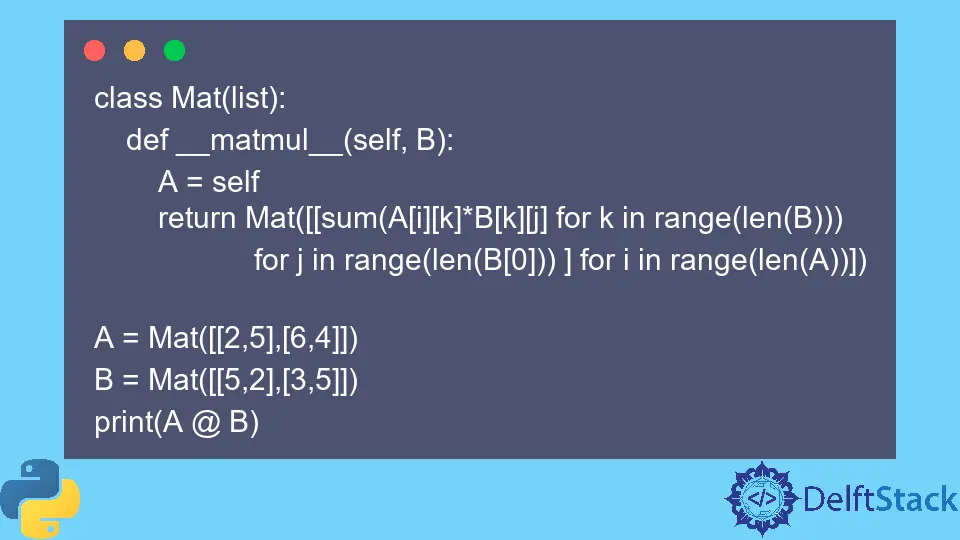
The most common use case of the @
symbol in Python is in decorators. A decorator lets you change the behavior of a function or class.
The @
symbol can also be used as a mathematical operator, as it can multiply matrices in Python. This tutorial will teach you to use Python’s @
symbol.
Use the @
Symbol in Decorators in Python
A decorator is a function accepting a function as an argument, adding some functionalities to it, and returning the modified function.
For example, see the following code.
def decorator(func):
return func
@decorator
def some_func():
pass
This is equivalent to the code below.
def decorator(func):
return func
def some_func():
pass
some_func = decorator(some_func)
A decorator modifies the original function without altering any script in the original function.
Let’s see a practical example of the above code snippet.
def message(func):
def wrapper():
print("Hello Decorator")
func()
return wrapper
def myfunc():
print("Hello World")
The @
symbol is used with the name of the decorator function. It should be written at the top of the function that will be decorated.
@message
def myfunc():
print("Hello World")
myfunc()
Output:
Hello Decorator
Hello World
The above decorator example does the same work as this code.
def myfunc():
print("Hello World")
myfunc = message(myfunc)
myfunc()
Output:
Hello Decorator
Hello World
Some commonly used decorators in Python are @property
, @classmethod
, and @staticmethod
.
Use the @
Symbol to Multiply Matrices in Python
From Python 3.5, the @
symbol can also be used as an operator to perform matrix multiplication in Python.
The following example is a simple implementation of multiplying matrices in Python.
class Mat(list):
def __matmul__(self, B):
A = self
return Mat(
[
[
sum(A[i][k] * B[k][j] for k in range(len(B)))
for j in range(len(B[0]))
]
for i in range(len(A))
]
)
A = Mat([[2, 5], [6, 4]])
B = Mat([[5, 2], [3, 5]])
print(A @ B)
Output:
[[25, 29], [42, 32]]
That’s it. The @
symbols in Python are used in decorators and matrix multiplications.
You should now understand what the @
symbol does in Python. We hope you find this tutorial helpful.