How to Display Text in Pygame
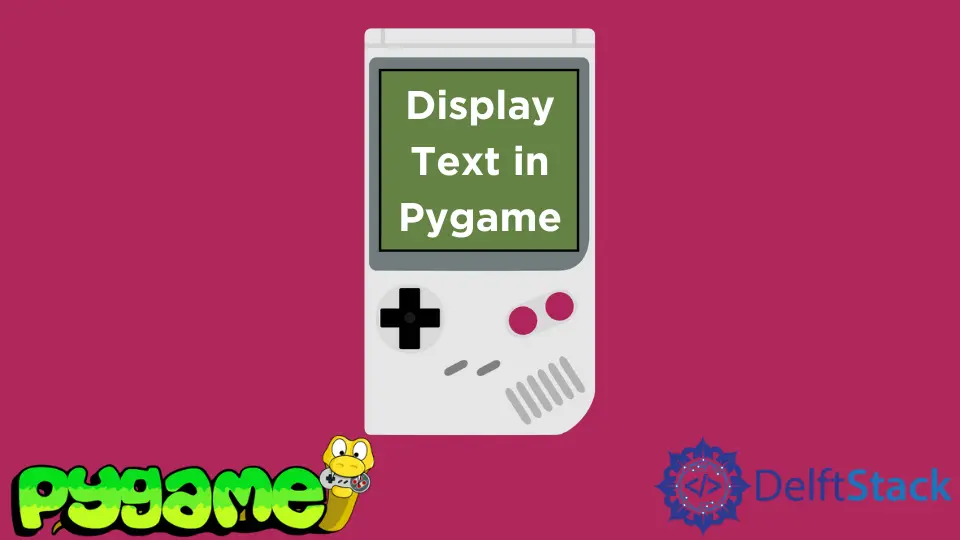
Creating engaging games often requires displaying text on the screen, whether it’s for instructions, scores, or dialogue. Pygame, a powerful library for game development in Python, offers a straightforward way to render text.
In this tutorial, we’ll guide you through the process of displaying text in Pygame, helping you enhance your game’s user experience. With easy-to-follow Python code examples and detailed explanations, you’ll be able to incorporate text seamlessly into your projects. Let’s dive in and learn how to effectively display text in Pygame!
Let’s create a basic Pygame window where we’ll display our text. The following code snippet sets up a simple Pygame window.
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Display Text in Pygame")
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0))
pygame.display.flip()
Output:
This code initializes Pygame, creates a window of size 800x600 pixels, and sets a title for it. The while
loop keeps the window open until you close it. The screen is filled with black color, providing a clean background for our text.
Displaying Basic Text
Now that we have our Pygame window set up, let’s move on to displaying text. Pygame uses the Font
class to render text. You can create a font object and then render text onto the screen. Here’s how you can do it:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Display Text in Pygame")
font = pygame.font.Font(None, 74)
text = font.render("Hello, Pygame!", True, (255, 255, 255))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0))
screen.blit(text, (250, 250))
pygame.display.flip()
Output:
In this code, we create a font object with a size of 74 pixels using pygame.font.Font(None, 74)
. The None
parameter indicates that we are using the default font. The text “Hello, Pygame!” is rendered in white using the render
method. Finally, we use blit
to draw the text onto the screen at the coordinates (250, 250).
Customizing Text Appearance
You might want to customize the appearance of your text further. This includes changing the font style, size, and color. Pygame allows you to load custom fonts and change the text color easily. Here’s an example of how to do that:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Display Text in Pygame")
font = pygame.font.Font("freesansbold.ttf", 64)
text = font.render("Customized Text!", True, (0, 255, 0))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((0, 0, 0))
screen.blit(text, (150, 250))
pygame.display.flip()
Output:
In this example, we load a custom font, freesansbold.ttf
, and set the font size to 64 pixels. The text color is changed to green by specifying the RGB value (0, 255, 0). This customization helps your text stand out and match the theme of your game.
Adding Text Effects
To make your text even more appealing, you might want to add effects like shadows or outlines. Pygame allows you to create these effects by rendering the text multiple times with different colors or positions. Here’s how you can create a shadow effect for your text:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Display Text in Pygame")
font = pygame.font.Font("freesansbold.ttf", 64)
text = font.render("Shadow Effect!", True, (255, 255, 255))
shadow = font.render("Shadow Effect!", True, (0, 0, 0))
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill((64, 64, 64))
screen.blit(shadow, (152, 252)) # Draw shadow first
screen.blit(text, (150, 250)) # Draw text on top
pygame.display.flip()
Output:
In this code, we first render the shadow text in black and then render the actual text in white slightly offset from the shadow. This creates a visually appealing effect that enhances the readability of your text against the background.
Conclusion
Displaying text in Pygame is a fundamental skill for game developers, allowing you to communicate effectively with players. From basic text rendering to customizing fonts and adding effects, Pygame provides all the tools you need to create engaging visual elements. By following the methods outlined in this tutorial, you can easily incorporate text into your games, making them more interactive and enjoyable. So, get creative with your text displays and enhance your Pygame projects today!
FAQ
-
How do I install Pygame?
You can install Pygame using the command pip install pygame in your terminal or command prompt. -
Can I use custom fonts in Pygame?
Yes, you can use custom fonts by specifying the font file path when creating a font object. -
How can I change the text color in Pygame?
You can change the text color by passing an RGB tuple as the third argument in the render method. -
Is it possible to add effects like shadows to text in Pygame?
Yes, you can create shadow effects by rendering the text multiple times with different colors or positions. -
What is the default font in Pygame?
The default font can be accessed by passing None when creating a font object, as shown in the examples.
Hi, my name is Maxim Maeder, I am a young programming enthusiast looking to have fun coding and teaching you some things about programming.
GitHub